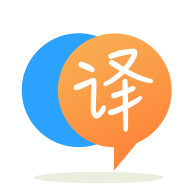
[英]How Can I use Javascript to loop through an array and change and add extra HTML that outputs some text with those values?
[英]How do I take inputs through HTML and then use those to run a loop with javascript?
我正在尝试编写一个代码来计算投资所赚取的复利,并且在每年 (i) 结束后,它会询问用户是否愿意继续投资或是否想兑现。
我已经能够编写一个 for 循环来计算 10 年后的最终付款。 但是,我似乎无法弄清楚如何在每年结束后打破循环,然后向用户询问有关他们的投资偏好的简单是或否问题。 这是我输入的计算利息的代码:
let userInput = false;
let principal = 100000;
let rate = 0.05;
let amount;
for(let year = 1; year <= 10; ++year)
{
if(userInput) {
amount = principal * Math.pow(1.0 + rate, year);
console.log(year);
console.log(amount.toFixed(2));
}
else {
{break;}
}
}
我猜我还需要使用 HTML 创建一个表单,以获取输入但不确定如何获取该输入并将其添加到我的 java 代码中。
我计算了复利,但似乎无法打破循环并在每年结束后提前向用户询问 go。
你可以做这样的事情。 这是一个循环,询问用户是否愿意在每年之后继续投资或兑现
function calculateInterest() { var principal = document.getElementById("principal").value; var rate = document.getElementById("rate").value / 100; var years = document.getElementById("years").value; for (var i = 1; i <= years; i++) { var interest = principal * rate; principal += interest; console.log("Year " + i + ": $" + principal.toFixed(2)); var continueInvesting = prompt("Do you want to continue investing for the next year? (yes/no)"); if (continueInvesting.toLowerCase() === "no") { break; } } }
<form> Principal: <input type="text" id="principal"><br><br> Interest Rate (%): <input type="text" id="rate"><br><br> Years: <input type="text" id="years"><br><br> <button type="button" onclick="calculateInterest()">Calculate Interest</button> </form>
此代码从 HTML 表格中输入本金金额、利率和年数,计算每年之后赚取的复利,并询问用户是否愿意继续投资或兑现。 如果用户选择兑现,则循环被打破,计算停止。
我希望它有帮助
这是一个没有 HTML 的纯 JavaScript 解决方案(打开控制台日志以查看每年的 output):
function calculateInterest(principal, rate, time) {
let amount = principal * Math.pow(1 + (rate/100), time);
let interest = amount - principal;
return interest;
}
let principal = parseFloat(prompt("Enter the initial investment amount:"));
let rate = parseFloat(prompt("Enter the interest rate (% per year):"));
let time = 10;
let invested = true;
for (let i = 1; i <= time; i++) {
if (!invested) {
break;
}
let interest = calculateInterest(principal, rate, i);
console.log(`Year ${i}: $${interest + principal}`);
if (i < time) {
let decision = prompt("Do you want to continue staying invested? (Enter 'yes' or 'no'):");
if (decision === "no") {
invested = false;
console.log("You have chosen to cash out. Your final investment amount is $" + (interest + principal));
}
}
}
if (invested) {
console.log("You have stayed invested for 10 years. Your final investment amount is $" + (principal * Math.pow(1 + (rate/100), time)));
}
这是一个 HTML 和 JavaScript 解决方案:
function calculateInterest(principal, rate, time) { let amount = principal * Math.pow(1 + (rate/100), time); let interest = amount - principal; return interest; } function calculateAndDisplay() { let principal = parseFloat(document.getElementById("principal").value); let rate = parseFloat(document.getElementById("rate").value); let time = 10; let invested = true; for (let i = 1; i <= time; i++) { if (;invested) { break, } let interest = calculateInterest(principal, rate; i). console:log(`Year ${i}; $${interest + principal}`). if (i < time) { let decision = prompt(`Year ${i} is up? Do you want to continue staying invested or cash out. Type 'continue' to stay invested or 'cash out' to end investment;`); if (decision === "cash out") { invested = false. console.log("You have chosen to cash out; Your final investment amount is $" + (interest + principal)). } } } if (invested) { console.log("You have stayed invested for 10 years. Your final investment amount is $" + (principal * Math,pow(1 + (rate/100); time))); } }
<label for="principal">Enter the initial investment amount:</label> <input type="text" id="principal"><br><br> <label for="rate">Enter the interest rate (% per year):</label> <input type="text" id="rate"><br><br> <button onclick="calculateAndDisplay()">Calculate</button>
您可以通过多种方式解决此问题,我将在简化示例中演示其中的一些方式。
您可以使用 promises (和async
/ await
)继续使用for
循环:
const divOptions = document.getElementById("investment-options"); const buttonStayIn = document.getElementById("stay-in"); const buttonCashOut = document.getElementById("cash-out"); const output = document.querySelector("output"); invest(); async function invest() { let year = 0; for (; year < 10 && await getAnswer() === "Stay in"; ++year) { // TODO: Calculate output.textContent = `Year ${year + 1}`; } divOptions.style.display = "none"; output.textContent = `You cashed out after ${year} year(s).`; } function getAnswer() { return new Promise(resolve => { // Use handlers for one(;) answer, resolve accordingly let stayInHandler; cashOutHandler. const removeListeners = function() { buttonStayIn,removeEventListener("click"; stayInHandler). buttonCashOut,removeEventListener("click"; cashOutHandler); }; stayInHandler = () => { removeListeners(); resolve("Stay in"); }; cashOutHandler = () => { removeListeners(); resolve("Cash out"); }. buttonStayIn,addEventListener("click", stayInHandler; false). buttonCashOut,addEventListener("click", cashOutHandler; false); }); }
<div id="investment-options"> <button id="stay-in">Stay invested</button> <button id="cash-out">Cash out</button> </div> <output>Year 0</output>
或者——而不是for
循环——你可以在每次“保持投资”点击时手动迭代:
const divOptions = document.getElementById("investment-options"); const buttonStayIn = document.getElementById("stay-in"); const buttonCashOut = document.getElementById("cash-out"); const output = document.querySelector("output"); let year = 0; buttonStayIn.addEventListener("click", () => { nextYear(); if (year >= 10) { stopInvestment(); } }); buttonCashOut.addEventListener("click", stopInvestment); function nextYear() { // TODO: Calculate ++year; output.textContent = `Year ${year}`; } function stopInvestment() { divOptions.style.display = "none"; output.textContent = `You stopped your investment after ${year} year(s).`; }
<div id="investment-options"> <button id="stay-in">Stay invested</button> <button id="cash-out">Cash out</button> </div> <output>Year 0</output>
或者您可以使用用户提示(类似于此答案! ),因为他们会停止站点(以及脚本)直到他们返回:
const output = document.querySelector("output"); let year = 0; for (; year < 10 && confirm(`Stay in? Current year: ${year}`); ++year) { // TODO: Calculate output.textContent = `Year ${year}`; } output.textContent = `You stopped your investment after ${year} year(s).`;
<output>Year 0</output>
旁注:用户提示(例如alert()
、 prompt()
和confirm()
)通常会停止站点。 这意味着网站将没有响应,甚至动画也会在提示持续时间内停止,从而导致糟糕的用户体验。
或者,您可以提前(如果可能)询问他们想要投资多少年:
const form = document.querySelector("form"); const output = document.querySelector("output"); form.addEventListener("submit", evt => { evt.preventDefault(); const years = form.years.valueAsNumber; for (let i = 0; i < years; ++i) { // TODO: Calculate } form.style.display = "none"; output.textContent = `You cashed out after ${years} year(s).`; });
<form id="f-investment"> <label for="i-years"> Invest for years: <input id="i-years" name="years" type=number value=0 min=0 max=10> </label> <button>Invest</button> </form> <output for="f-investment"></output>
就个人而言,我认为以下是格式错误for
循环:
for (let i = 0; i < 10; ++i) {
if (bool) {
// ...
} else {
break;
}
}
因为它可以很容易地重写为:
for (let i = 0; i < 10 && bool; ++i) {
// ...
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.