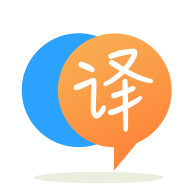
[英]Read numbers separated by comma and write them to a file separated by tab
[英]How to read-write into/from text file with comma separated values
如果我的文件是逗号分隔值,我如何从文件中读取数据
1, 2, 3, 4, 5\n
6, 7, 8, 9, 10\n
\n
在读完文件后,我想将数据写回到上面相同格式的其他文件中。
我可以使用获得总行数
string line;
while(!file.eof()){
getline(file,line);
numlines++;
}
numline--; // remove the last empty line
但我如何知道行/行中的总位数?
我还有一些存储数据的向量。 所以,我想读取第一行然后计算该行中元素的总数,这里是5(1,2,3,4,5)并将它们存储在数组/向量中,然后读取下一行并将它们存储在向量中再等等,直到我到达EOF。
然后,我想将数据写入文件,我想这将完成将数据写入文件的工作,
numOfCols=1;
for(int i = 0; i < vector.size(); i++)
{
file << vector.at(i);
if((numOfCols<5) file << ",";//print comma (,)
if((i+1)%5==0)
{
file << endl;//print newline after 5th value
numOfCols=1;//start from column 1 again, for the next line
}
numOfCols++;
}
file << endl;// last new line
所以,我的主要问题是如何用逗号分隔值从文件中读取数据?
谢谢
第1步:不要这样做:
while(!file.eof())
{
getline(file,line);
numlines++;
}
numline--;
在您尝试阅读之前,EOF是不正确的。 标准模式是:
while(getline(file,line))
{
++numline;
}
另请注意, std::getline()
可以选择使用第三个参数。 这是要打破的角色。 默认情况下,这是行终止符,但您可以指定逗号。
while(getline(file,line))
{
std::stringstream linestream(line);
std::string value;
while(getline(linestream,value,','))
{
std::cout << "Value(" << value << ")\n";
}
std::cout << "Line Finished" << std::endl;
}
如果将所有值存储在单个矢量中,则使用固定宽度将其打印出来。 然后我会做这样的事情。
struct LineWriter
{
LineWriter(std::ostream& str,int size)
:m_str(str)
,m_size(size)
,m_current(0)
{}
// The std::copy() does assignement to an iterator.
// This looks like this (*result) = <value>;
// So overide the operator * and the operator = to
LineWriter& operator*() {return *this;}
void operator=(int val)
{
++m_current;
m_str << val << (((m_current % m_size) == 0)?"\n":",");
}
// std::copy() increments the iterator. But this is not usfull here
// so just implement too empty methods to handle the increment.
void operator++() {}
void operator++(int) {}
// Local data.
std::ostream& m_str;
int const m_size;
int m_current;
};
void printCommaSepFixedSizeLinesFromVector(std::vector const& data,int linesize)
{
std::copy(data.begin(),data.end(),LineWriter(std::cout,linesize));
}
这里我发布了CSV读取代码以及编写代码。 我检查了它的工作正常。
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
using namespace std;
void readCSV(istream &input, vector< vector<string> > &output)
{
string csvLine;
// read every line from the stream
while( getline(input, csvLine) )
{
istringstream csvStream(csvLine);
vector<string> csvColumn;
string csvElement;
// read every element from the line that is seperated by commas
// and put it into the vector or strings
while( getline(csvStream, csvElement, ',') )
{
csvColumn.push_back(csvElement);
}
output.push_back(csvColumn);
}
}
int main()
{
ofstream myfile;
string a;
fstream file("b.csv", ios::in);
myfile.open ("ab.csv");
if(!file.is_open())
{
cout << "File not found!\n";
return 1;
}
// typedef to save typing for the following object
typedef vector< vector<string> > csvVector;
csvVector csvData;
readCSV(file, csvData);
// print out read data to prove reading worked
for(csvVector::iterator i = csvData.begin(); i != csvData.end(); ++i)
{
for(vector<string>::iterator j = i->begin(); j != i->end(); ++j)
{
a=*j;
cout << a << " ";
myfile <<a<<",";
}
myfile <<"\n";
cout << "\n";
}
myfile.close();
system("pause");
}
尝试stringstream类:
#include <sstream>
以防万一,查看Stroustrup的“The C ++ Programming Language Special Edition”页面641示例。
它适用于我,我有一段时间试图找出这个。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.