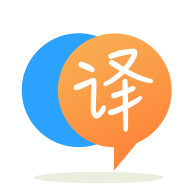
[英]How I can save image with using a best practices in Laravel (php)?
[英]Best Practices: How to check for a constant in an array using PHP?
我不太确定该怎么做。 我有一个对象,并且想在其上设置一个'type'属性,但是在此之前,我想检查一下以确保它是有效的类型。
我以为这是使用常量的好时机,因为类型名称不会更改(我也不希望它们更改)。
// this is just a sample of what I was thinking:
class Foobar
{
const TYPE_1 = 'type 1';
protected $_types = array(
self::TYPE_1
);
public function setType($value)
{
// check to make sure value is a valid type
// I'm thinking it should be able to accept
// Foobar::TYPE_1, 'TYPE_1', or 'type 1'
// for flexibility sake. But I don't know
}
}
$foobar = new Foobar();
$foobar->setType('TYPE_1'); //what should go here? (best practice)
更新:我认为我一开始确实不需要使用常量,但是我接受了一个我认为可以完成工作的答案。
如果您的数组具有所有类型$_types
,则可以直接检查其中是否存在value:
class Foobar
{
const TYPE_1 = 'type 1';
## declare all available types as static variable
## don't forget to change it when new type will be added
protected static $_types = array(
self::TYPE_1
);
public function setType($value) {
if (in_array($value, self::$_types)) {
// type is valid, we can use it
}
else {
// type is wrong, reporting error
throw new Exception("wrong type [".$value."]");
}
}
}
但这需要额外的工作来支持此数组,以确保所有可能的类型都在数组中。 也应该是static
。 因此,唯一的数据副本将用于所有实例。
要获得类中的可用常量,您将必须使用Reflection 。
例如:
$ref = new ReflectionClass( $this );
$constants = $ref->getConstants();
// Constants now contains an associative array with the keys being
// the constant name, and the value the constant value.
如果您要打印数组。 您将看到字符串值。 因此,您只需检查$ value是否在$ _values中。
你可以做这样的事情。 实际的代码是我从生产应用程序中提取的一些代码,但是您明白了。
<?php
class Example {
protected $SEARCH_TYPES = array('keyword', 'title', 'subject');
function getSearchType($val) {
if (in_array($val, $this->SEARCH_TYPES)) {
return $val;
}
return FALSE;
}
}
?>
in_array
将为您提供帮助,但请注意, in_array
必须扫描所有数组以找到该值。 所以可能是这样的:
class Foobar
{
const TYPE_1 = 'type 1';
protected $_types = array(
self::TYPE_1 => true
);
public function setType($value)
{
$value = strtolower($value); // case-insensitive
if(isset($this->_types[$value]) // check "type 1"
|| isset($this->_types[str_replace($value, '_', ' ')])) // check "type_1"
{
// OK!
} else {
throw new Exception("Invalid type: $value");
}
}
}
如果您还想检查Foobar :: TYPE_1,请使用constant($ value) -它允许检查类常量。 或者,如果您想要确切的类名,请使用反射:
list($class, $const) = explode('::', $value);
$refclass = new ReflectionClass($this);
if(get_class($this) == $class && $refclass->getConstant($value)) {
// OK!
}
您不能将数组设置为类变量的值!
参见http://php.net/manual/en/language.oop5.constants.php
该值必须是常量表达式, 而不是变量,属性,数学运算的结果或函数调用。
您可以通过为变量而不是常量使用setter和getter方法来实现此功能。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.