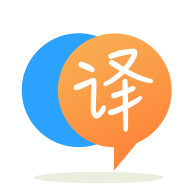
[英]How do I get the key and it's object from a JSON file in c# using JSON.net?
[英]How do I get formatted JSON in .NET using C#?
我正在使用 .NET JSON 解析器,并且想序列化我的配置文件以便它可读。 所以不是:
{"blah":"v", "blah2":"v2"}
我想要更好的东西,比如:
{
"blah":"v",
"blah2":"v2"
}
我的代码是这样的:
using System.Web.Script.Serialization;
var ser = new JavaScriptSerializer();
configSz = ser.Serialize(config);
using (var f = (TextWriter)File.CreateText(configFn))
{
f.WriteLine(configSz);
f.Close();
}
您将很难使用 JavaScriptSerializer 完成此任务。
试试JSON.Net 。
对 JSON.Net 示例稍作修改
using System;
using Newtonsoft.Json;
namespace JsonPrettyPrint
{
internal class Program
{
private static void Main(string[] args)
{
Product product = new Product
{
Name = "Apple",
Expiry = new DateTime(2008, 12, 28),
Price = 3.99M,
Sizes = new[] { "Small", "Medium", "Large" }
};
string json = JsonConvert.SerializeObject(product, Formatting.Indented);
Console.WriteLine(json);
Product deserializedProduct = JsonConvert.DeserializeObject<Product>(json);
}
}
internal class Product
{
public String[] Sizes { get; set; }
public decimal Price { get; set; }
public DateTime Expiry { get; set; }
public string Name { get; set; }
}
}
结果
{
"Sizes": [
"Small",
"Medium",
"Large"
],
"Price": 3.99,
"Expiry": "\/Date(1230447600000-0700)\/",
"Name": "Apple"
}
文档: 序列化对象
Json.Net 库的较短示例代码
private static string FormatJson(string json)
{
dynamic parsedJson = JsonConvert.DeserializeObject(json);
return JsonConvert.SerializeObject(parsedJson, Formatting.Indented);
}
如果您有一个 JSON 字符串并想对其进行“美化”,但又不想将其序列化为已知的 C# 类型或从已知的 C# 类型序列化,那么以下方法可以解决问题(使用 JSON.NET):
using System;
using System.IO;
using Newtonsoft.Json;
class JsonUtil
{
public static string JsonPrettify(string json)
{
using (var stringReader = new StringReader(json))
using (var stringWriter = new StringWriter())
{
var jsonReader = new JsonTextReader(stringReader);
var jsonWriter = new JsonTextWriter(stringWriter) { Formatting = Formatting.Indented };
jsonWriter.WriteToken(jsonReader);
return stringWriter.ToString();
}
}
}
美化现有 JSON 的最短版本:(编辑:使用 JSON.net)
JToken.Parse("mystring").ToString()
输入:
{"menu": { "id": "file", "value": "File", "popup": { "menuitem": [ {"value": "New", "onclick": "CreateNewDoc()"}, {"value": "Open", "onclick": "OpenDoc()"}, {"value": "Close", "onclick": "CloseDoc()"} ] } }}
输出:
{
"menu": {
"id": "file",
"value": "File",
"popup": {
"menuitem": [
{
"value": "New",
"onclick": "CreateNewDoc()"
},
{
"value": "Open",
"onclick": "OpenDoc()"
},
{
"value": "Close",
"onclick": "CloseDoc()"
}
]
}
}
}
要漂亮地打印对象:
JToken.FromObject(myObject).ToString()
Oneliner 使用Newtonsoft.Json.Linq
:
string prettyJson = JToken.Parse(uglyJsonString).ToString(Formatting.Indented);
所有这些都可以在一个简单的行中完成:
string jsonString = JsonConvert.SerializeObject(yourObject, Formatting.Indented);
这是使用 Microsoft 的System.Text.Json库的解决方案:
static string FormatJsonText(string jsonString)
{
using var doc = JsonDocument.Parse(
jsonString,
new JsonDocumentOptions
{
AllowTrailingCommas = true
}
);
MemoryStream memoryStream = new MemoryStream();
using (
var utf8JsonWriter = new Utf8JsonWriter(
memoryStream,
new JsonWriterOptions
{
Indented = true
}
)
)
{
doc.WriteTo(utf8JsonWriter);
}
return new System.Text.UTF8Encoding()
.GetString(memoryStream.ToArray());
}
您可以使用以下标准方法获取格式化的 Json
JsonReaderWriterFactory.CreateJsonWriter(Stream stream, Encoding encoding, bool ownsStream, bool indent, string indentChars)
只设置“缩进==真”
尝试这样的事情
public readonly DataContractJsonSerializerSettings Settings =
new DataContractJsonSerializerSettings
{ UseSimpleDictionaryFormat = true };
public void Keep<TValue>(TValue item, string path)
{
try
{
using (var stream = File.Open(path, FileMode.Create))
{
//var currentCulture = Thread.CurrentThread.CurrentCulture;
//Thread.CurrentThread.CurrentCulture = CultureInfo.InvariantCulture;
try
{
using (var writer = JsonReaderWriterFactory.CreateJsonWriter(
stream, Encoding.UTF8, true, true, " "))
{
var serializer = new DataContractJsonSerializer(type, Settings);
serializer.WriteObject(writer, item);
writer.Flush();
}
}
catch (Exception exception)
{
Debug.WriteLine(exception.ToString());
}
finally
{
//Thread.CurrentThread.CurrentCulture = currentCulture;
}
}
}
catch (Exception exception)
{
Debug.WriteLine(exception.ToString());
}
}
注意线条
var currentCulture = Thread.CurrentThread.CurrentCulture;
Thread.CurrentThread.CurrentCulture = CultureInfo.InvariantCulture;
....
Thread.CurrentThread.CurrentCulture = currentCulture;
对于某些类型的 xml 序列化器,您应该使用InvariantCulture以避免在具有不同区域设置的计算机上反序列化期间出现异常。 例如, double或DateTime 的无效格式有时会导致它们。
用于反序列化
public TValue Revive<TValue>(string path, params object[] constructorArgs)
{
try
{
using (var stream = File.OpenRead(path))
{
//var currentCulture = Thread.CurrentThread.CurrentCulture;
//Thread.CurrentThread.CurrentCulture = CultureInfo.InvariantCulture;
try
{
var serializer = new DataContractJsonSerializer(type, Settings);
var item = (TValue) serializer.ReadObject(stream);
if (Equals(item, null)) throw new Exception();
return item;
}
catch (Exception exception)
{
Debug.WriteLine(exception.ToString());
return (TValue) Activator.CreateInstance(type, constructorArgs);
}
finally
{
//Thread.CurrentThread.CurrentCulture = currentCulture;
}
}
}
catch
{
return (TValue) Activator.CreateInstance(typeof (TValue), constructorArgs);
}
}
谢谢!
netcoreapp3.1
var js = JsonSerializer.Serialize(obj, new JsonSerializerOptions {
WriteIndented = true
});
使用System.Text.Json
设置JsonSerializerOptions.WriteIndented = true
:
JsonSerializerOptions options = new JsonSerializerOptions { WriteIndented = true };
string json = JsonSerializer.Serialize<Type>(object, options);
对于那些问我如何使用 C# 将 JSON 格式化为 .NET 并想立即查看如何使用它的人和在线爱好者。 以下是缩进的 JSON 字符串单行代码:
有 2 个著名的 JSON 格式化程序或解析器可以序列化:
using Newtonsoft.Json;
var jsonString = JsonConvert.SerializeObject(yourObj, Formatting.Indented);
using System.Text.Json;
var jsonString = JsonSerializer.Serialize(yourObj, new JsonSerializerOptions { WriteIndented = true });
首先我想在 Duncan Smart 帖子下添加评论,但不幸的是我还没有足够的声誉来发表评论。 所以我会在这里尝试。
我只想警告副作用。
JsonTextReader 在内部将 json 解析为类型化的 JToken,然后将它们序列化回来。
例如,如果您的原始 JSON 是
{ "double":0.00002, "date":"\/Date(1198908717056)\/"}
美化后你得到
{
"double":2E-05,
"date": "2007-12-29T06:11:57.056Z"
}
当然两个json字符串是等价的,会反序列化为结构上相等的对象,但是如果你需要保留原始字符串值,你需要考虑到这一点
这对我有用。 如果有人正在寻找 VB.NET 版本。
@imports System
@imports System.IO
@imports Newtonsoft.Json
Public Shared Function JsonPrettify(ByVal json As String) As String
Using stringReader = New StringReader(json)
Using stringWriter = New StringWriter()
Dim jsonReader = New JsonTextReader(stringReader)
Dim jsonWriter = New JsonTextWriter(stringWriter) With {
.Formatting = Formatting.Indented
}
jsonWriter.WriteToken(jsonReader)
Return stringWriter.ToString()
End Using
End Using
End Function
我有一些非常简单的东西。 您可以将任何要转换为 json 格式的对象作为输入:
private static string GetJson<T> (T json)
{
return JsonConvert.SerializeObject(json, Formatting.Indented);
}
下面的代码对我有用:
JsonConvert.SerializeObject(JToken.Parse(yourobj.ToString()))
对于使用 .NET Core 3.1 的 UTF8 编码的 JSON 文件,我终于能够根据来自 Microsoft 的这些信息使用 JsonDocument: https ://docs.microsoft.com/en-us/dotnet/standard/serialization/system-text-json -how-to#utf8jsonreader-utf8jsonwriter-and-jsondocument
string allLinesAsOneString = string.Empty;
string [] lines = File.ReadAllLines(filename, Encoding.UTF8);
foreach(var line in lines)
allLinesAsOneString += line;
JsonDocument jd = JsonDocument.Parse(Encoding.UTF8.GetBytes(allLinesAsOneString));
var writer = new Utf8JsonWriter(Console.OpenStandardOutput(), new JsonWriterOptions
{
Indented = true
});
JsonElement root = jd.RootElement;
if( root.ValueKind == JsonValueKind.Object )
{
writer.WriteStartObject();
}
foreach (var jp in root.EnumerateObject())
jp.WriteTo(writer);
writer.WriteEndObject();
writer.Flush();
.NET 5 内置了用于在 System.Text.Json 命名空间下处理 JSON 解析、序列化、反序列化的类。 下面是将 .NET 对象转换为 JSON 字符串的序列化程序示例,
using System.Text.Json;
using System.Text.Json.Serialization;
private string ConvertJsonString(object obj)
{
JsonSerializerOptions options = new JsonSerializerOptions();
options.WriteIndented = true; //Pretty print using indent, white space, new line, etc.
options.NumberHandling = JsonNumberHandling.AllowNamedFloatingPointLiterals; //Allow NANs
string jsonString = JsonSerializer.Serialize(obj, options);
return jsonString;
}
using System.Text.Json;
...
var parsedJson = JsonSerializer.Deserialize<ExpandoObject>(json);
var options = new JsonSerializerOptions() { WriteIndented = true };
return JsonSerializer.Serialize(parsedJson, options);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.