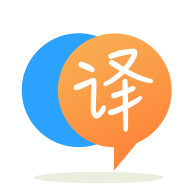
[英]Get value from uncomputed css style with javascript / jquery
[英]Get a CSS value from external style sheet with Javascript/jQuery
如果样式所引用的元素尚未生成,是否可以从页面的外部 CSS 中获取值? (元素是动态生成的)。
我见过的 jQuery 方法是$('element').css('property');
,但这依赖于页面上的element
。 有没有办法找出在 CSS 中设置的属性而不是元素的计算样式?
我是否必须做一些丑陋的事情,例如将元素的隐藏副本添加到我的页面,以便我可以访问其样式属性?
使用 jQuery:
// Scoping function just to avoid creating a global (function() { var $p = $("<p></p>").hide().appendTo("body"); console.log($p.css("color")); $p.remove(); })();
p {color: blue}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
直接使用DOM:
// Scoping function just to avoid creating a global (function() { var p = document.createElement('p'); document.body.appendChild(p); console.log(getComputedStyle(p).color); document.body.removeChild(p); })();
p {color: blue}
注意:在这两种情况下,如果您正在加载外部样式表,您需要等待它们加载以查看它们对元素的影响。 jQuery 的ready
和 DOM 的DOMContentLoaded
事件都没有做到这一点,您必须通过观察它们加载来确保它。
通常,您应该让浏览器应用所有规则,然后向浏览器询问结果,但在极少数情况下,您确实需要从样式表中获取值,您可以使用:( JSFiddle )
function getStyleSheetPropertyValue(selectorText, propertyName) {
// search backwards because the last match is more likely the right one
for (var s= document.styleSheets.length - 1; s >= 0; s--) {
var cssRules = document.styleSheets[s].cssRules ||
document.styleSheets[s].rules || []; // IE support
for (var c=0; c < cssRules.length; c++) {
if (cssRules[c].selectorText === selectorText)
return cssRules[c].style[propertyName];
}
}
return null;
}
alert(getStyleSheetPropertyValue("p", "color"));
请注意,这非常脆弱,因为您必须提供与您正在查找的规则匹配的完整选择器文本(未解析),并且它不处理重复条目或任何类型的优先规则。 我很难想到使用它是个好主意的案例,但这里只是一个例子。
在回应 Karim79 时,我只是想我会扔掉那个答案的函数版本。 我不得不这样做几次,所以这就是我写的:
function getClassStyles(parentElem, selector, style){
elemstr = '<div '+ selector +'></div>';
var $elem = $(elemstr).hide().appendTo(parentElem);
val = $elem.css(style);
$elem.remove();
return val;
}
val = getClassStyles('.container:first', 'class="title"', 'margin-top');
console.warn(val);
这个例子假设你有一个带有 class="container" 的元素,并且你正在寻找那个元素中标题类的边距顶部样式。 当然可以根据自己的需要进行调整。
在样式表中:
.container .title{ margin-top:num; }
让我知道您的想法 - 您会修改它吗,如果是,如何修改? 谢谢!
我编写了一个辅助函数,它接受一个对象,该对象具有要从给定 css 类中检索的 css 属性,并填充实际的 css 属性值。 包括示例。
function getStyleSheetValues(colScheme) {
var tags='';
var obj= colScheme;
// enumerate css classes from object
for (var prop in obj) {
if (obj.hasOwnProperty(prop) && typeof obj[prop]=="object") {
tags+= '<span class="'+prop+'"></span>';
}
}
// generate an object that uses the given classes
tags= $('<div>'+tags+'</div>').hide().appendTo("body");
// read the class properties from the generated object
var idx= 0;
for (var prop in obj) {
if (obj.hasOwnProperty(prop) && typeof obj[prop]=="object") {
var nobj= obj[prop];
for (var nprop in nobj) {
if (nobj.hasOwnProperty(nprop) && typeof(nobj[nprop])=="string") {
nobj[nprop]= tags.find("span:eq("+idx+")").css(nobj[nprop]);
}
}
idx++;
}
}
tags.remove();
}
// build an object with css class names where each class name contains one
// or more properties with an arbitrary name and the css attribute name as its value.
// This value will be replaced by the actual css value for the respective class.
var colorScheme= { chart_wall: {wallColor:'background-color',wallGrid:'color'}, chart_line1: { color:'color'} };
$(document).ready(function() {
getStyleSheetValues(colorScheme);
// debug: write the property values to the console;
if (window.console) {
var obj= colorScheme;
for (var prop in obj) {
if (obj.hasOwnProperty(prop) && typeof obj[prop]=="object") {
var nobj= obj[prop];
for (var nprop in nobj) {
if (nobj.hasOwnProperty(nprop)) {
console.log(prop+'.'+nprop +':'+ nobj[nprop]);
}
}
}
}
// example of how to read an individual css attribute value
console.log('css value for chart_wall.wallGrid: '+colorScheme.chart_wall.wallGrid);
}
});
我写了这个 js 函数,似乎也适用于嵌套类:
用法:
var style = get_css_property('.container-class .sub-container-class .child-class', 'margin');
console.log('style');
function get_css_property(class_name, property_name){
class_names = class_name.split(/\s+/);
var container = false;
var child_element = false;
for (var i = class_names.length - 1; i >= 0; i--) {
if(class_names[i].startsWith('.'))
class_names[i] = class_names[i].substring(1);
var new_element = $.parseHTML('<div class="' + class_names[i] + '"></div>');
if(!child_element)
child_element = new_element;
if(container)
$(new_element).append(container);
container = new_element;
}
$(container).hide().appendTo('body');
var style = $(child_element).css(property_name);
$(container).remove();
return style;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.