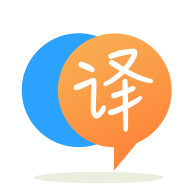
[英]Why do I'm getting error: NoSuchAlgorithmException; must be caught or declared to be thrown?
[英]Why am I getting "must be caught or declared to be thrown" on my program?
我已经在这个程序上工作了很长一段时间,我的大脑被炸了。 我可以从别人那里得到一些帮助。
我正在尝试制作一个逐行读取文本文件的程序,每一行都被制成一个ArrayList
这样我就可以访问每个令牌。 我究竟做错了什么?
import java.util.*;
import java.util.ArrayList;
import java.io.*;
import java.rmi.server.UID;
import java.util.concurrent.atomic.AtomicInteger;
public class PCB {
public void read (String [] args) {
BufferedReader inputStream = null;
try {
inputStream = new BufferedReader(new FileReader("processes1.txt"));
String l;
while ((l = inputStream.readLine()) != null) {
write(l);
}
}
finally {
if (inputStream != null) {
inputStream.close();
}
}
}
public void write(String table) {
char status;
String name;
int priority;
ArrayList<String> tokens = new ArrayList<String>();
Scanner tokenize = new Scanner(table);
while (tokenize.hasNext()) {
tokens.add(tokenize.next());
}
status = 'n';
name = tokens.get(0);
String priString = tokens.get(1);
priority = Integer.parseInt(priString);
AtomicInteger count = new AtomicInteger(0);
count.incrementAndGet();
int pid = count.get();
System.out.println("PID: " + pid);
}
}
我都快把眼球挖出来了。 我得到了三个错误:
U:\Senior Year\CS451- Operating Systems\Project1 PCB\PCB.java:24: unreported exception java.io.IOException; must be caught or declared to be thrown
inputStream.close();}
^
U:\Senior Year\CS451- Operating Systems\Project1 PCB\PCB.java:15: unreported exception java.io.FileNotFoundException; must be caught or declared to be thrown
inputStream = new BufferedReader(new FileReader("processes1.txt"));
^
U:\Senior Year\CS451- Operating Systems\Project1 PCB\PCB.java:18: unreported exception java.io.IOException; must be caught or declared to be thrown
while ((l = inputStream.readLine()) != null) {
^
我究竟做错了什么?
当您在 Java 中使用 I/O 时,大部分时间您必须处理 IOException,这可能会在您读/写甚至关闭流时发生。
您必须将敏感块放在 try//catch 块中并在此处处理异常。
例如:
try{
// All your I/O operations
}
catch(IOException ioe){
//Handle exception here, most of the time you will just log it.
}
资源:
Java 在编译时检查异常规范。 您必须捕获异常或在方法签名中声明它抛出。 以下是您如何声明它可能会从您的方法中抛出:
public void read (String [] args) throws java.io.IOException {
如果您的方法需要做一些响应,请捕获异常。 如果您的调用者需要知道失败,则将其声明为抛出。
这些并不相互排斥。 有时捕获异常,做一些事情并重新抛出异常或包装原始异常(“原因”)的新异常很有用。
RuntimeException 及其子类不需要声明。
好的 IDE 会为您创建 catch 块或将异常添加到方法声明中。
请注意,如果您按照 Colin 的解决方案将异常添加到方法声明中,则调用您的方法的任何方法也必须具有合适的 catch 块或在方法声明中声明异常。
你宁愿做
try{
// All your I/O operations
}
catch(Exception e){
//Handle exception here, most of the time you will just log it.
}
一般来说,在一段时间内捕获类本身并不是一个坏主意,如果您需要非常具体的日志,则决定将逻辑转换为捕获或执行 instanceof。
每当“未报告的异常 IOException;必须被捕获或声明为抛出”时,就会出现此错误,然后需要将代码放入 try catch 块中。 例子
try{
// All your I/O operations
}
catch(IOException ioe){
//Handle exception here, most of the time you will just log it.
}
我曾经也有过一样的问题。 我通过添加 spring 库“org.springframework.core”解决了这个问题
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.