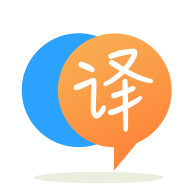
[英]how to set the selected value tag <select> html from database in php?
[英]How to set selected value of HTML select box with PHP
我有下一块模板:
<select name="interest">
<option value="seo">SEO и Блоговодство</option>
<option value="auto">Авто</option>
<option value="business">Бизнес</option>
<option value="design">Дизайн</option>
...
并将结果值存储在$result['interest']
中。
如何使用 PHP 将option
元素标记为选中?
谢谢!
手动方式.....
<select name="interest">
<option value="seo"<?php if($result['interest'] == 'seo'): ?> selected="selected"<?php endif; ?>>SEO и Блоговодство</option>
.....
更好的方法是遍历兴趣
$interests = array(
'seo' => 'SEO и Блоговодство',
'auto' => 'Авто',
....
);
<select name="interest">
<?php foreach( $interests as $var => $interest ): ?>
<option value="<?php echo $var ?>"<?php if( $var == $result['interest'] ): ?> selected="selected"<?php endif; ?>><?php echo $interest ?></option>
<?php endforeach; ?>
</select>
<?php
$interests = array('seo' => 'SEO и Блоговодство', 'auto' => 'Aвто', 'business' => 'Бизнес', ...);
?>
<select name="interest">
<?php
foreach($interests as $k => $v) {
?>
<option value="<?php echo $k; ?>" <?php if($k == $result['interest']) ?> selected="selected" <?php } ?>><?php echo $v;?></option>
<?php
}
?>
</select>
<?php
$list='<select name="interest">
<option value="seo">SEO и Блоговодство</option>
<option value="auto">Авто</option>
<option value="business">Бизнес</option>
<option value="design">Дизайн</option>
...';
echo str_replace('value="' . $result['interest'] . '"','value="' . $result['interest'] . '" selected',$list);
?> 这涉及制作一个包含您的列表的字符串,然后使用字符串替换功能找到正确的选项并将选定的选项添加到标签中。 如果使用 XHTML,您将需要使用 selected="selected"。
http://sandbox.onlinephpfunctions.com/code/37eb8f5a213fe5a252cd4da6712f3db0c5558ae3
<select name="interest">
<option value="seo"<?php if($result['interest'] == 'seo'){ echo ' selected="selected"'; } ?>>SEO</option>
<option value="auto"<?php if($result['interest'] == 'auto'){ echo ' selected="selected"'; } ?>>Auto</option>
<option value="business"<?php if($result['interest'] == 'business'){ echo ' selected="selected"'; } ?>>Business</option>
<option value="design"<?php if($result['interest'] == 'design'){ echo ' selected="selected"'; } ?>>Design</option>
</select>
<select name="interest">
<option value="seo" <?php echo $result['interest'] == 'seo' ? 'selected' : ''?> >SEO и Блоговодство</option>
<option value="auto" <?php echo $result['interest'] == 'auto' ? 'selected' : ''?>>Авто</option>
<option value="business" <?php echo $result['interest'] == 'business' ? 'selected' : ''?>>Бизнес</option>
<option value="design" <?php echo $result['interest'] == 'design' ? 'selected' : ''?>>Дизайн</option>
您需要让 PHP 为适当的<option>
标签插入selected="selected"
属性,如下所示:
<?php $result=$userRow['ad_type']; ?>
<select class="form-control" name="ad_type">
<option <?php if($result == 'text'){ echo ' selected="selected"'; } ?> value="text">Text</option>
<option <?php if($result == 'image'){ echo ' selected="selected"'; } ?> value="image">Image</option>
<option <?php if($result == 'video'){ echo ' selected="selected"'; } ?> value="video">Video</option>
<option <?php if($result == 'htmladsense'){ echo ' selected="selected"'; } ?> value="htmladsense">Html Adsense</option>
</select>
另一种方式(在 WordPress 中使用) 链接到使用可重用性的Wordpress 代码是:
<select name='result[interest]' style="width:400px">
<option value='SEO' <?php selected($result['interest'], 'SEO'); ?>>SEO</option>
<option value='AUTO' <?php selected($result['interest'], 'AUTO'); ?>>Auto</option>
</select>
selected() 函数确定是否选择了该值并设置所选选项。
/**
* Outputs the html selected attribute.
*
* Compares the first two arguments and if identical marks as selected
*
* @since 1.0.0
*
* @param mixed $selected One of the values to compare
* @param mixed $current (true) The other value to compare if not just true
* @param bool $echo Whether to echo or just return the string
* @return string html attribute or empty string
*/
function selected( $selected, $current = true, $echo = true ) {
return __checked_selected_helper( $selected, $current, $echo, 'selected' );
}
selected() 函数依次调用下面的辅助函数来比较和确定该值是否被选中。 它返回 'selected=selected' 或 ''。
/**
* Private helper function for checked, selected, disabled and readonly.
*
* Compares the first two arguments and if identical marks as $type
*
* @since 2.8.0
* @access private
*
* @param mixed $helper One of the values to compare
* @param mixed $current (true) The other value to compare if not just true
* @param bool $echo Whether to echo or just return the string
* @param string $type The type of checked|selected|disabled|readonly we are doing
* @return string html attribute or empty string
*/
function __checked_selected_helper( $helper, $current, $echo, $type ) {
if ( (string) $helper === (string) $current ) {
$result = " $type='$type'";
} else {
$result = '';
}
if ( $echo ) {
echo $result;
}
return $result;
}
简单的速记方法是
//after pulling your content from database
$value = interest['value'];
<option value="seo" <?php echo ($value == "seo") ? 'selected = "selected"' : '' ;?> >SEO и Блоговодство</option>
...
我希望这会有所帮助
不需要写任何东西。 只需输入以下代码
`<div class="form-group">
<label for="title">Blog Trending Or Not ?</label><br>
<select class="custom-select" id="inputGroupSelect01" name="interest">
<option value="seo"<?php if($row['is_trending']=="seo"){echo "selected";} ?>>SEO и Блоговодство</option>
<option value="auto"<?php if($row['is_trending']=="auto"){echo "selected";} ?>>Авто</option>
<option value="business"<?php if($row['is_trending']=="business"){echo "selected";} ?>>Бизнес</option>
<option value="design"<?php if($row['is_trending']=="design"){echo "selected";} ?>>Дизайн</option>
</select>
</div>`
通过设置一个包含您的选项数据的数组来保持您的脚本干燥和清洁。 循环遍历数组并将数据打印到带有占位符的模板字符串中,以避免丑陋、笨拙的插值、连接和内联条件。
代码:(演示)
$interests = [
'seo' => 'SEO и Блоговодство',
'auto' => 'Aвто',
'business' => 'Бизнес',
'design' => 'Дизайн',
];
$selected = 'business';
echo '<select name="interest">';
foreach ($interests as $value => $text) {
printf(
'<option value="%s"%s>%s</option>',
$value,
$selected === $value ? ' selected' : '',
$text
);
}
echo '</select>';
对于任何付出额外努力来生成带有适当制表符的行分隔选项的人,有几种方法可以在 PHP 中打印时添加制表符和换行符,但我不会在这里演示它们。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.