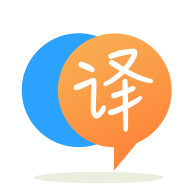
[英]How to hide soft keyboard on android after clicking outside EditText? in fragment
[英]How to hide soft keyboard on android after clicking outside EditText?
好的,每个人都知道要隐藏键盘,您需要实现:
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
但是这里的大问题是当用户触摸或选择任何其他不是EditText
或 softKeyboard 的地方时如何隐藏键盘?
我尝试在我的父Activity
上使用onTouchEvent()
,但这仅在用户触摸任何其他视图之外并且没有滚动视图时才有效。
我试图实现一个触摸、点击、聚焦监听器,但没有成功。
我什至尝试实现自己的滚动视图来拦截触摸事件,但我只能获取事件的坐标而不是单击的视图。
有没有标准的方法来做到这一点? 在 iPhone 中,这真的很容易。
以下代码段只是隐藏了键盘:
public static void hideSoftKeyboard(Activity activity) {
InputMethodManager inputMethodManager =
(InputMethodManager) activity.getSystemService(
Activity.INPUT_METHOD_SERVICE);
if(inputMethodManager.isAcceptingText()){
inputMethodManager.hideSoftInputFromWindow(
activity.getCurrentFocus().getWindowToken(),
0
);
}
}
您可以将其放在实用程序类中,或者如果您在活动中定义它,请避免使用活动参数,或调用hideSoftKeyboard(this)
。
最棘手的部分是何时调用它。 您可以编写一个方法来遍历活动中的每个View
,并检查它是否是instanceof EditText
,如果它没有向该组件注册setOnTouchListener
并且一切都会到位。 如果您想知道如何做到这一点,它实际上非常简单。 这就是你要做的,你写一个像下面这样的递归方法,实际上你可以用它来做任何事情,比如设置自定义字体等......这是方法
public void setupUI(View view) {
// Set up touch listener for non-text box views to hide keyboard.
if (!(view instanceof EditText)) {
view.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
hideSoftKeyboard(MyActivity.this);
return false;
}
});
}
//If a layout container, iterate over children and seed recursion.
if (view instanceof ViewGroup) {
for (int i = 0; i < ((ViewGroup) view).getChildCount(); i++) {
View innerView = ((ViewGroup) view).getChildAt(i);
setupUI(innerView);
}
}
}
就是这样,只需在您的活动中setContentView
后调用此方法。 如果您想知道要传递什么参数,它是父容器的id
。 为您的父容器分配一个id
,例如
<RelativeLayoutPanel android:id="@+id/parent"> ... </RelativeLayout>
并调用setupUI(findViewById(R.id.parent))
,仅此而已。
如果你想有效地使用它,你可以创建一个扩展的Activity
并放入这个方法,并让你的应用程序中的所有其他活动都扩展这个 Activity 并在onCreate()
方法中调用它的setupUI()
。
希望能帮助到你。
如果您使用超过 1 个活动,请为父布局定义公共 id,例如<RelativeLayout android:id="@+id/main_parent"> ... </RelativeLayout>
然后从Activity
扩展一个类并在其OnResume()
中定义setupUI(findViewById(R.id.main_parent))
并in your program
扩展此类而不是 ``Activity
这是上述函数的 Kotlin 版本:
@file:JvmName("KeyboardUtils")
fun Activity.hideSoftKeyboard() {
currentFocus?.let {
val inputMethodManager = ContextCompat.getSystemService(this, InputMethodManager::class.java)!!
inputMethodManager.hideSoftInputFromWindow(it.windowToken, 0)
}
}
您可以通过执行以下步骤来实现此目的:
通过添加以下属性使父视图(您的活动的内容视图)可点击和可聚焦
android:clickable="true" android:focusableInTouchMode="true"
实现一个 hideKeyboard() 方法
public void hideKeyboard(View view) { InputMethodManager inputMethodManager =(InputMethodManager)getSystemService(Activity.INPUT_METHOD_SERVICE); inputMethodManager.hideSoftInputFromWindow(view.getWindowToken(), 0); }
最后,设置编辑文本的 onFocusChangeListener。
edittext.setOnFocusChangeListener(new View.OnFocusChangeListener() { @Override public void onFocusChange(View v, boolean hasFocus) { if (!hasFocus) { hideKeyboard(v); } } });
正如以下评论之一所指出的,如果父视图是 ScrollView,这可能不起作用。 对于这种情况,可以在 ScrollView 正下方的视图上添加 clickable 和 focusableInTouchMode。
只需在 Activity 中覆盖以下代码
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
if (getCurrentFocus() != null) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
return super.dispatchTouchEvent(ev);
}
更新:
如果有人需要这个答案的 Kotlin 版本:
override fun dispatchTouchEvent(ev: MotionEvent?): Boolean {
if (currentFocus != null) {
val imm = activity!!.getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager
imm.hideSoftInputFromWindow(activity!!.currentFocus!!.windowToken, 0)
}
return super.dispatchTouchEvent(ev)
}
我发现接受的答案有点复杂。
这是我的解决方案。 将OnTouchListener
添加到您的主布局中,即:
findViewById(R.id.mainLayout).setOnTouchListener(this)
并将以下代码放入 onTouch 方法中。
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
这样您就不必遍历所有视图。
我得到了另一种隐藏键盘的解决方案:
InputMethodManager imm = (InputMethodManager) getSystemService(
Activity.INPUT_METHOD_SERVICE);
imm.toggleSoftInput(InputMethodManager.HIDE_IMPLICIT_ONLY, 0);
这里在 showFlag 的位置传递HIDE_IMPLICIT_ONLY
,在showFlag
的位置hiddenFlag
0
。 它将强制关闭软键盘。
使用TextInputEditText的更多Kotlin & Material Design方式(这种方法也与EditTextView兼容)......
1.通过添加以下属性使父视图(您的活动/片段的内容视图)可点击和可聚焦
android:focusable="true"
android:focusableInTouchMode="true"
android:clickable="true"
2.为所有视图创建一个扩展名(例如在 ViewExtension.kt 文件中):
fun View.hideKeyboard(){
val inputMethodManager = context.getSystemService(Activity.INPUT_METHOD_SERVICE) as InputMethodManager
inputMethodManager.hideSoftInputFromWindow(this.windowToken, 0)
}
3.创建继承TextInputEditText的BaseTextInputEditText。 实现 onFocusChanged 方法以在视图未聚焦时隐藏键盘:
class BaseTextInputEditText(context: Context?, attrs: AttributeSet?) : TextInputEditText(context, attrs){
override fun onFocusChanged(focused: Boolean, direction: Int, previouslyFocusedRect: Rect?) {
super.onFocusChanged(focused, direction, previouslyFocusedRect)
if (!focused) this.hideKeyboard()
}
}
4.只需在您的 XML 中调用您全新的自定义视图:
<android.support.design.widget.TextInputLayout
android:id="@+id/textInputLayout"
...>
<com.your_package.BaseTextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
... />
</android.support.design.widget.TextInputLayout>
就这样。 无需修改您的控制器(片段或活动)来处理这种重复的情况。
好吧,我设法在一定程度上解决了这个问题,我在我的活动中覆盖了 dispatchTouchEvent,我正在使用以下内容来隐藏键盘。
/**
* Called to process touch screen events.
*/
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
switch (ev.getAction()){
case MotionEvent.ACTION_DOWN:
touchDownTime = SystemClock.elapsedRealtime();
break;
case MotionEvent.ACTION_UP:
//to avoid drag events
if (SystemClock.elapsedRealtime() - touchDownTime <= 150){
EditText[] textFields = this.getFields();
if(textFields != null && textFields.length > 0){
boolean clickIsOutsideEditTexts = true;
for(EditText field : textFields){
if(isPointInsideView(ev.getRawX(), ev.getRawY(), field)){
clickIsOutsideEditTexts = false;
break;
}
}
if(clickIsOutsideEditTexts){
this.hideSoftKeyboard();
}
} else {
this.hideSoftKeyboard();
}
}
break;
}
return super.dispatchTouchEvent(ev);
}
编辑: getFields() 方法只是一个返回视图中包含文本字段的数组的方法。 为了避免在每次触摸时都创建这个数组,我创建了一个名为 sFields 的静态数组,它在 getFields() 方法中返回。 该数组在 onStart() 方法上初始化,例如:
sFields = new EditText[] {mUserField, mPasswordField};
它并不完美,拖动事件时间仅基于启发式,因此有时在执行长 clics 时它不会隐藏,我还通过创建一个方法来获取每个视图的所有 editTexts 完成; 否则,当单击其他 EditText 时,键盘会隐藏并显示。
尽管如此,还是欢迎更清洁和更短的解决方案
在任何 Activity(或扩展 Activity 类)中覆盖 public boolean dispatchTouchEvent(MotionEvent event)
@Override
public boolean dispatchTouchEvent(MotionEvent event) {
View view = getCurrentFocus();
boolean ret = super.dispatchTouchEvent(event);
if (view instanceof EditText) {
View w = getCurrentFocus();
int scrcoords[] = new int[2];
w.getLocationOnScreen(scrcoords);
float x = event.getRawX() + w.getLeft() - scrcoords[0];
float y = event.getRawY() + w.getTop() - scrcoords[1];
if (event.getAction() == MotionEvent.ACTION_UP
&& (x < w.getLeft() || x >= w.getRight()
|| y < w.getTop() || y > w.getBottom()) ) {
InputMethodManager imm = (InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getWindow().getCurrentFocus().getWindowToken(), 0);
}
}
return ret;
}
科特林版本:
override fun dispatchTouchEvent(ev: MotionEvent?): Boolean {
val ret = super.dispatchTouchEvent(ev)
ev?.let { event ->
if (event.action == MotionEvent.ACTION_UP) {
currentFocus?.let { view ->
if (view is EditText) {
val touchCoordinates = IntArray(2)
view.getLocationOnScreen(touchCoordinates)
val x: Float = event.rawX + view.getLeft() - touchCoordinates[0]
val y: Float = event.rawY + view.getTop() - touchCoordinates[1]
//If the touch position is outside the EditText then we hide the keyboard
if (x < view.getLeft() || x >= view.getRight() || y < view.getTop() || y > view.getBottom()) {
val imm = getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager
imm.hideSoftInputFromWindow(view.windowToken, 0)
view.clearFocus()
}
}
}
}
}
return ret
}
这就是你需要做的所有事情
例如:
editText.setOnFocusChangeListener(new View.OnFocusChangeListener() {
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (!hasFocus) {
hideKeyboard();
}
}
});
更新:您还可以在活动中覆盖onTouchEvent()
并检查触摸坐标。 如果坐标在 EditText 之外,则隐藏键盘。
我在 Activity 中实现了 dispatchTouchEvent 来做到这一点:
private EditText mEditText;
private Rect mRect = new Rect();
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
final int action = MotionEventCompat.getActionMasked(ev);
int[] location = new int[2];
mEditText.getLocationOnScreen(location);
mRect.left = location[0];
mRect.top = location[1];
mRect.right = location[0] + mEditText.getWidth();
mRect.bottom = location[1] + mEditText.getHeight();
int x = (int) ev.getX();
int y = (int) ev.getY();
if (action == MotionEvent.ACTION_DOWN && !mRect.contains(x, y)) {
InputMethodManager input = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
input.hideSoftInputFromWindow(mEditText.getWindowToken(), 0);
}
return super.dispatchTouchEvent(ev);
}
我测试了它,完美!
我修改了 Andre Luis IM 的解决方案,我实现了这个:
我创建了一个实用方法来隐藏软键盘,就像 Andre Luiz IM 所做的那样:
public static void hideSoftKeyboard(Activity activity) {
InputMethodManager inputMethodManager = (InputMethodManager) activity.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(activity.getCurrentFocus().getWindowToken(), 0);
}
但是我没有为每个视图注册一个 OnTouchListener,这会导致性能很差,我只为根视图注册了 OnTouchListener。 由于事件冒泡直到被消费(EditText是默认消费它的视图之一),如果它到达根视图,那是因为它没有被消费,所以我关闭了软键盘。
findViewById(android.R.id.content).setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
Utils.hideSoftKeyboard(activity);
return false;
}
});
我知道这个线程已经很老了,正确的答案似乎是有效的,并且有很多可行的解决方案,但我认为下面所述的方法可能在效率和优雅方面有额外的好处。
我的所有活动都需要这种行为,所以我创建了一个继承自类Activity的类CustomActivity并“挂钩”了dispatchTouchEvent函数。 主要有两个条件需要注意:
这是我的结果:
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
if(ev.getAction() == MotionEvent.ACTION_UP) {
final View view = getCurrentFocus();
if(view != null) {
final boolean consumed = super.dispatchTouchEvent(ev);
final View viewTmp = getCurrentFocus();
final View viewNew = viewTmp != null ? viewTmp : view;
if(viewNew.equals(view)) {
final Rect rect = new Rect();
final int[] coordinates = new int[2];
view.getLocationOnScreen(coordinates);
rect.set(coordinates[0], coordinates[1], coordinates[0] + view.getWidth(), coordinates[1] + view.getHeight());
final int x = (int) ev.getX();
final int y = (int) ev.getY();
if(rect.contains(x, y)) {
return consumed;
}
}
else if(viewNew instanceof EditText || viewNew instanceof CustomEditText) {
return consumed;
}
final InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(viewNew.getWindowToken(), 0);
viewNew.clearFocus();
return consumed;
}
}
return super.dispatchTouchEvent(ev);
}
旁注:此外,我将这些属性分配给根视图,从而可以清除每个输入字段的焦点并防止输入字段获得对活动启动的关注(使内容视图成为“焦点捕捉器”):
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final View view = findViewById(R.id.content);
view.setFocusable(true);
view.setFocusableInTouchMode(true);
}
而不是遍历所有视图或覆盖 dispatchTouchEvent。
为什么不直接覆盖 Activity 的 onUserInteraction() 这将确保当用户在 EditText 之外点击时键盘会消失。
即使 EditText 在滚动视图内也可以工作。
@Override
public void onUserInteraction() {
if (getCurrentFocus() != null) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
}
在 kotlin 中,我们可以执行以下操作。 无需迭代所有视图。 它也适用于片段。
override fun dispatchTouchEvent(ev: MotionEvent?): Boolean {
currentFocus?.let {
val imm: InputMethodManager = getSystemService(
Context.INPUT_METHOD_SERVICE
) as (InputMethodManager)
imm.hideSoftInputFromWindow(it.windowToken, 0)
}
return super.dispatchTouchEvent(ev)
}
我喜欢调用 htafoya 制作的dispatchTouchEvent
的方法,但是:
所以,我做了这个更简单的解决方案:
@Override
public boolean dispatchTouchEvent(final MotionEvent ev) {
// all touch events close the keyboard before they are processed except EditText instances.
// if focus is an EditText we need to check, if the touchevent was inside the focus editTexts
final View currentFocus = getCurrentFocus();
if (!(currentFocus instanceof EditText) || !isTouchInsideView(ev, currentFocus)) {
((InputMethodManager) getApplicationContext().getSystemService(Context.INPUT_METHOD_SERVICE))
.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), InputMethodManager.HIDE_NOT_ALWAYS);
}
return super.dispatchTouchEvent(ev);
}
/**
* determine if the given motionevent is inside the given view.
*
* @param ev
* the given view
* @param currentFocus
* the motion event.
* @return if the given motionevent is inside the given view
*/
private boolean isTouchInsideView(final MotionEvent ev, final View currentFocus) {
final int[] loc = new int[2];
currentFocus.getLocationOnScreen(loc);
return ev.getRawX() > loc[0] && ev.getRawY() > loc[1] && ev.getRawX() < (loc[0] + currentFocus.getWidth())
&& ev.getRawY() < (loc[1] + currentFocus.getHeight());
}
有一个缺点:
从一个EditText
切换到另一个EditText
会使键盘隐藏并重新显示 - 在我的情况下,这是需要的,因为它表明您在两个输入组件之间切换。
恳求:我承认我没有影响力,但请认真对待我的回答。
问题:当点击离开键盘或用最少的代码编辑文本时关闭软键盘。
解决方案:称为Butterknife 的外部库。
一条线解决方案:
@OnClick(R.id.activity_signup_layout) public void closeKeyboard() { ((InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE)).hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0); }
更具可读性的解决方案:
@OnClick(R.id.activity_signup_layout)
public void closeKeyboard() {
InputMethodManager imm = (InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
说明:将 OnClick 侦听器绑定到活动的 XML 布局父 ID,以便对布局的任何点击(而不是在编辑文本或键盘上)将运行将隐藏键盘的代码片段。
示例:如果您的布局文件是 R.layout.my_layout 并且您的布局 id 是 R.id.my_layout_id,那么您的 Butterknife 绑定调用应如下所示:
(@OnClick(R.id.my_layout_id)
public void yourMethod {
InputMethodManager imm = (InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
Butterknife 文档链接:http: //jakewharton.github.io/butterknife/
Plug: Butterknife 将彻底改变您的 android 开发。 考虑一下。
注意:不使用外部库 Butterknife 也可以达到相同的结果。 如上所述,只需将 OnClickListener 设置为父布局。
它太简单了,只需通过以下代码使您最近的布局可点击成为焦点:
android:id="@+id/loginParentLayout"
android:clickable="true"
android:focusableInTouchMode="true"
然后为该布局编写一个方法和一个 OnClickListner ,这样当触摸最上面的布局时,它会调用一个方法,您将在其中编写代码以关闭键盘。 以下是两者的代码; // 你必须在 OnCreate() 中写这个
yourLayout.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View view) {
hideKeyboard(view);
}
});
从侦听器调用的方法:-
public void hideKeyboard(View view) {
InputMethodManager imm =(InputMethodManager)getSystemService(Activity.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(view.getWindowToken(), 0);
}
这是 fje 答案的另一个变体,它解决了 sosite 提出的问题。
这里的想法是在 Activity 的dispatchTouchEvent
方法中同时处理 down 和 up 动作。 在向下操作中,我们记下当前聚焦的视图(如果有)以及触摸是否在其中,将这两个信息都保存下来以备后用。
在 up 动作上,我们首先调度,以允许另一个视图潜在地获得焦点。 如果在那之后,当前聚焦的视图是最初聚焦的视图,并且向下触摸在该视图内,那么我们让键盘保持打开状态。
如果当前聚焦的视图与最初聚焦的视图不同并且它是一个EditText
,那么我们也让键盘保持打开状态。
否则我们关闭它。
因此,总而言之,它的工作原理如下:
EditText
内触摸时,键盘保持打开状态EditText
移动到另一个EditText
时,键盘保持打开状态(不关闭/重新打开)EditText
之外的任何地方时,不是另一个EditText
,键盘关闭EditText
以调出上下文操作栏(使用剪切/复制/粘贴按钮)时,键盘保持打开状态,即使 UP 操作发生在焦点EditText
之外(向下移动以为出租车)。 但请注意,当您点击 CAB 中的按钮时,它将关闭键盘。 这可能是可取的,也可能不是可取的; 如果您想从一个字段剪切/复制并粘贴到另一个字段,那就是。 如果您想粘贴回相同的EditText
,则不会。 当聚焦的EditText
位于屏幕底部并且您长按某些文本以选择它时, EditText
会保持焦点,因此键盘会按您想要的方式打开,因为我们对“触摸在视图范围内”进行了检查向下动作,而不是向上动作。
private View focusedViewOnActionDown; private boolean touchWasInsideFocusedView; @Override public boolean dispatchTouchEvent(MotionEvent ev) { switch (ev.getAction()) { case MotionEvent.ACTION_DOWN: focusedViewOnActionDown = getCurrentFocus(); if (focusedViewOnActionDown != null) { final Rect rect = new Rect(); final int[] coordinates = new int[2]; focusedViewOnActionDown.getLocationOnScreen(coordinates); rect.set(coordinates[0], coordinates[1], coordinates[0] + focusedViewOnActionDown.getWidth(), coordinates[1] + focusedViewOnActionDown.getHeight()); final int x = (int) ev.getX(); final int y = (int) ev.getY(); touchWasInsideFocusedView = rect.contains(x, y); } break; case MotionEvent.ACTION_UP: if (focusedViewOnActionDown != null) { // dispatch to allow new view to (potentially) take focus final boolean consumed = super.dispatchTouchEvent(ev); final View currentFocus = getCurrentFocus(); // if the focus is still on the original view and the touch was inside that view, // leave the keyboard open. Otherwise, if the focus is now on another view and that view // is an EditText, also leave the keyboard open. if (currentFocus.equals(focusedViewOnActionDown)) { if (touchWasInsideFocusedView) { return consumed; } } else if (currentFocus instanceof EditText) { return consumed; } // the touch was outside the originally focused view and not inside another EditText, // so close the keyboard InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE); inputMethodManager.hideSoftInputFromWindow( focusedViewOnActionDown.getWindowToken(), 0); focusedViewOnActionDown.clearFocus(); return consumed; } break; } return super.dispatchTouchEvent(ev); }
对于这个简单的要求,我发现接受的答案有点复杂。 这对我有用,没有任何故障。
findViewById(R.id.mainLayout).setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
return false;
}
});
这对我来说是最简单的解决方案(并且由我制定)。
这是隐藏键盘的方法。
public void hideKeyboard(View view){
if(!(view instanceof EditText)){
InputMethodManager inputMethodManager=(InputMethodManager)getSystemService(INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(),0);
}
}
现在将活动的父布局的 onclick 属性设置为上述方法hideKeyboard
从 XML 文件的设计视图或在 XML 文件的文本视图中编写以下代码。
android:onClick="hideKeyboard"
有一种更简单的方法,基于 iPhone 相同的问题。 只需在包含编辑文本的触摸事件上覆盖背景的布局。 只需在活动的 OnCreate 中使用此代码(login_fondo 是根布局):
final LinearLayout llLogin = (LinearLayout)findViewById(R.id.login_fondo);
llLogin.setOnTouchListener(
new OnTouchListener()
{
@Override
public boolean onTouch(View view, MotionEvent ev) {
InputMethodManager imm = (InputMethodManager) mActivity.getSystemService(
android.content.Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(mActivity.getCurrentFocus().getWindowToken(), 0);
return false;
}
});
显示/隐藏软键盘的方法
InputMethodManager inputMethodManager = (InputMethodManager) currentActivity.getSystemService(Context.INPUT_METHOD_SERVICE);
if (isShow) {
if (currentActivity.getCurrentFocus() == null) {
inputMethodManager.toggleSoftInput(InputMethodManager.SHOW_FORCED, 0);
} else {
inputMethodManager.showSoftInput(currentActivity.getCurrentFocus(), InputMethodManager.SHOW_FORCED);
}
} else {
if (currentActivity.getCurrentFocus() == null) {
inputMethodManager.toggleSoftInput(InputMethodManager.HIDE_NOT_ALWAYS, 0);
} else {
inputMethodManager.hideSoftInputFromInputMethod(currentActivity.getCurrentFocus().getWindowToken(), InputMethodManager.HIDE_NOT_ALWAYS);
}
}
我希望它们有用
我在 Fernando Camarago 的解决方案上做了一个小改动。 在我的 onCreate 方法中,我将单个 onTouchListener 附加到根视图,但将视图而不是活动作为参数发送。
findViewById(android.R.id.content).setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
Utils.hideSoftKeyboard(v);
return false;
}
});
在一个单独的 Utils 类中是......
public static void hideSoftKeyboard(View v) {
InputMethodManager imm = (InputMethodManager) v.getContext().getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(v.getWindowToken(), 0);
}
我已经这样做了:
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
View view = getCurrentFocus();
if (view != null && (ev.getAction() == MotionEvent.ACTION_UP || ev.getAction() == MotionEvent.ACTION_MOVE) && view instanceof EditText && !view.getClass().getName().startsWith("android.webkit.")) {
int scrcoords[] = new int[2];
view.getLocationOnScreen(scrcoords);
float x = ev.getRawX() + view.getLeft() - scrcoords[0];
float y = ev.getRawY() + view.getTop() - scrcoords[1];
if (x < view.getLeft() || x > view.getRight() || y < view.getTop() || y > view.getBottom())
hideKeyboard(this);
}
return super.dispatchTouchEvent(ev);
}
隐藏键盘代码:
public static void hideKeyboard(Activity act) {
if(act!=null)
((InputMethodManager)act.getSystemService(Context.INPUT_METHOD_SERVICE)).hideSoftInputFromWindow((act.getWindow().getDecorView().getApplicationWindowToken()), 0);
}
完毕
要解决此问题,您必须首先使用该 Edittext 的 setOnFocusChangeListener
edittext.setOnFocusChangeListener(new View.OnFocusChangeListener() {
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (!hasFocus) {
Log.d("focus", "focus loosed");
// Do whatever you want here
} else {
Log.d("focus", "focused");
}
}
});
然后你需要做的是在包含 Edittext 的活动中覆盖 dispatchTouchEvent 见下面的代码
@Override
public boolean dispatchTouchEvent(MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
View v = getCurrentFocus();
if ( v instanceof EditText) {
Rect outRect = new Rect();
v.getGlobalVisibleRect(outRect);
if (!outRect.contains((int)event.getRawX(), (int)event.getRawY())) {
Log.d("focus", "touchevent");
v.clearFocus();
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(v.getWindowToken(), 0);
}
}
}
return super.dispatchTouchEvent(event);
}
现在会发生什么,当用户点击外部时,首先会调用这个 dispatchTouchEvent,然后会从editext中清除焦点,现在你的 OnFocusChangeListener 将被调用,焦点已经改变,现在在这里你可以做任何你想做的事情,希望它能工作
我已经改进了该方法,将以下代码放在一些 UI 实用程序类中(最好,不一定),以便可以从所有 Activity 或 Fragment 类访问它以达到其目的。
public static void serachAndHideSoftKeybordFromView(View view, final Activity act) {
if(!(view instanceof EditText)) {
view.setOnTouchListener(new View.OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
hideSoftKeyboard(act);
return false;
}
});
}
if (view instanceof ViewGroup) {
for (int i = 0; i < ((ViewGroup) view).getChildCount(); i++) {
View nextViewInHierarchy = ((ViewGroup) view).getChildAt(i);
serachAndHideSoftKeybordFromView(nextViewInHierarchy, act);
}
}
}
public static void hideSoftKeyboard (Activity activity) {
InputMethodManager inputMethodManager = (InputMethodManager) activity.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(activity.getCurrentFocus().getWindowToken(), 0);
}
然后说比如你需要从activity中调用它,调用方式如下;
UIutils.serachAndHideSoftKeybordFromView(findViewById(android.R.id.content), YourActivityName.this);
注意
findViewById(android.R.id.content)
这为我们提供了当前组的根视图(您不能在根视图上设置 id)。
干杯:)
尝试将 stateHidden 作为您的活动windowSoftInputMode
值
http://developer.android.com/reference/android/R.attr.html#windowSoftInputMode
例如对于您的活动:
this.getWindow().setSoftInputMode(
WindowManager.LayoutParams.SOFT_INPUT_STATE_HIDDEN);
活动
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
ScreenUtils.hideKeyboard(this, findViewById(android.R.id.content).getWindowToken());
return super.dispatchTouchEvent(ev);
}
屏幕工具
public static void hideKeyboard(Context context, IBinder windowToken) {
InputMethodManager imm = (InputMethodManager) context.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(windowToken, InputMethodManager.HIDE_NOT_ALWAYS);
}
只需在 @Overide 类中添加此代码
public boolean dispatchTouchEvent(MotionEvent ev) {
View view = getCurrentFocus();
if (view != null && (ev.getAction() == MotionEvent.ACTION_UP || ev.getAction() == MotionEvent.ACTION_MOVE) && view instanceof EditText && !view.getClass().getName().startsWith("android.webkit.")) {
int scrcoords[] = new int[2];
view.getLocationOnScreen(scrcoords);
float x = ev.getRawX() + view.getLeft() - scrcoords[0];
float y = ev.getRawY() + view.getTop() - scrcoords[1];
if (x < view.getLeft() || x > view.getRight() || y < view.getTop() || y > view.getBottom())
((InputMethodManager)this.getSystemService(Context.INPUT_METHOD_SERVICE)).hideSoftInputFromWindow((this.getWindow().getDecorView().getApplicationWindowToken()), 0);
}
return super.dispatchTouchEvent(ev);
}
您可以实现 View.onClickListener 并覆盖 onClick 方法并将此 onclicklistener 设置为布局
ConstraintLayout constraintLayout;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
constraintLayout = findViewById(R.id.layout);
constraintLayout.setOnClickListener(this);
}
@Override
public void onClick(View v) {
if(v.getId()==R.id.layout){
InputMethodManager inm = (InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
inm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(),0);
}
}
这可能很旧,但我通过实现一个自定义类来完成这个工作
public class DismissKeyboardListener implements OnClickListener {
Activity mAct;
public DismissKeyboardListener(Activity act) {
this.mAct = act;
}
@Override
public void onClick(View v) {
if ( v instanceof ViewGroup ) {
hideSoftKeyboard( this.mAct );
}
}
}
public void hideSoftKeyboard(Activity activity) {
InputMethodManager imm = (InputMethodManager)
getSystemService(Activity.INPUT_METHOD_SERVICE);
imm.toggleSoftInput(InputMethodManager.HIDE_IMPLICIT_ONLY, 0);
}
这里的最佳实践是创建一个 Helper 类,并且每个容器相对/线性布局都应该实现它。
****注意只有主容器应该实现这个类(用于优化)****
并像这样实现它:
Parent.setOnClickListener( new DismissKeyboardListener(this) );
这是用于活动的关键字。 所以如果你在片段上,你可以使用 getActivity();
---如果对您有帮助,请竖起大拇指... --- 欢呼拉尔夫 ---
这是 fje 答案的一个稍微修改过的版本,大部分工作都很完美。
此版本使用 ACTION_DOWN,因此执行滚动操作也会关闭键盘。 除非您单击另一个 EditText,否则它也不会传播该事件。 这意味着点击 EditText 之外的任何地方,即使是在另一个可点击的地方,也会简单地关闭键盘。
@Override
public boolean dispatchTouchEvent(MotionEvent ev)
{
if(ev.getAction() == MotionEvent.ACTION_DOWN)
{
final View view = getCurrentFocus();
if(view != null)
{
final View viewTmp = getCurrentFocus();
final View viewNew = viewTmp != null ? viewTmp : view;
if(viewNew.equals(view))
{
final Rect rect = new Rect();
final int[] coordinates = new int[2];
view.getLocationOnScreen(coordinates);
rect.set(coordinates[0], coordinates[1], coordinates[0] + view.getWidth(), coordinates[1] + view.getHeight());
final int x = (int) ev.getX();
final int y = (int) ev.getY();
if(rect.contains(x, y))
{
super.dispatchTouchEvent(ev);
return true;
}
}
else if(viewNew instanceof EditText || viewNew instanceof CustomEditText)
{
super.dispatchTouchEvent(ev);
return true;
}
final InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(viewNew.getWindowToken(), 0);
viewNew.clearFocus();
return true;
}
}
return super.dispatchTouchEvent(ev);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
InputMethodManager imm = (InputMethodManager)getSystemService(Context.
INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
return true;
}
好吧,您可以使用此代码,使用您的主布局 ID 而不是“mainRelativeLayout”
//hide Soft keyboard on click outside the input text
findViewById(R.id.mainRelativeLayout).setOnClickListener(new
View.OnClickListener() {
@Override
public void onClick(View v) {
InputMethodManager im = (InputMethodManager)
getSystemService(INPUT_METHOD_SERVICE);
im.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(),
0);
}
});
我稍微修改了答案@navneeth-g,增加了空活动焦点处理,避免了多个实例创建OnTouchListener,键盘隐藏时移除焦点,屏幕滚动时移除隐藏键盘,在小型设备上方便。
//In general, the view parameter is root layout
fun Activity.hideKeyboardOnClickOutsideEditText(view: View) {
// Set up touch listener for non-text box views to hide keyboard.
var previousAction = 0
val onTouchListener = View.OnTouchListener { v, event ->
if (currentFocus != null
&& event.action != MotionEvent.ACTION_DOWN
&& event.action != MotionEvent.ACTION_MOVE
&& previousAction != MotionEvent.ACTION_MOVE
) {
currentFocus?.clearFocus()
v?.hideKeyboard()
}
previousAction = event.action
false
}
if (view !is EditText) {
view.setOnTouchListener(onTouchListener)
}
//If a layout container, iterate over children and seed recursion.
if (view is ViewGroup) {
for (i in 0 until view.childCount) {
val innerView = view.getChildAt(i)
hideKeyboardOnClickOutsideEditText(innerView)
}
}
}
//in root layout.xml
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true"
非常困难的决定。 我建议一个更简单的解决方案。 在 onViewCreated 中,我们为整个 View 设置了一个 setOnClickListener 并从 EditText 中移除焦点,以及移除键盘。
片段中的示例。 爪哇。
@Override
public void onViewCreated(View view, Bundle savedInstanceState)
{
super.onViewCreated(view, savedInstanceState);
nameTextInputEditText = view.findViewById(R.id.NameTextInputEditText);
view.setOnClickListener(v -> {
nameTextInputEditText.clearFocus();
InputMethodManager imm = (InputMethodManager) requireActivity().getSystemService(Activity.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(v.getWindowToken(), 0);
});
}
您可以轻松地覆盖活动和片段中的 onKey() 事件以隐藏键盘。
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
if (keyCode == event.KEYCODE_ENTER) {
intiateLoginProcess();
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getWindow().getCurrentFocus()
.getWindowToken(), 0);
return true;
}
}
return false;
}
嘿伙计们,我有这个问题的简单解决方案,这个解决方案可用于简单的注册或登录表单。 我的解决方案与我在 ios setontouch 监听器中实现的主视图相同
activity_main.xml 将 ID 添加到您的主要相对布局android:id="@+id/mainlayout"
并将此代码添加到您的活动中
RelativeLayout mainLayout = (RelativeLayout)findViewById(R.id.mainlayout);
mainLayout.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
// TODO Auto-generated method stub
Log.d("Json Response", "Touch outside");
InputMethodManager inputMethodManager = (InputMethodManager) MainActivity.this.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(MainActivity.this.getCurrentFocus().getWindowToken(), 0);
return false;
}
});
我想到了这个问题。 首先,我认为 setOnTouchListener 不是简单的解决方案。 所以我相信 dispatchTouchEvent 是最好的简单解决方案。
public boolean dispatchKeyEvent(KeyEvent event) {
if (event.getAction() == KeyEvent.ACTION_UP) {
View v = getCurrentFocus();
if (v instanceof EditText) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
}
return super.dispatchKeyEvent(event);
}
在这里,一个重要的是ACTION_UP。
我假设 EditText 只显示软键盘,否则不显示键盘。 我在 Android5.0.1(LG 的 G3.cat6)上测试过。
如果您需要拖动检查,长按,...,请在上面显示评论。
我的解决方案在任何活动中隐藏外部点击键盘,以及所有编辑文本。 没有一一指定。
首先添加到布局xml的根视图: android:clickable="true" android:focusableInTouchMode="true"
接下来,为所有要隐藏键盘的活动创建一个父 Acitvity,并指定 onResume() 方法:
@Override
protected void onResume() {
super.onResume();
//getting Root View that gets focus
View rootView =((ViewGroup)findViewById(android.R.id.content)).
getChildAt(0);
rootView.setOnFocusChangeListener(new View.OnFocusChangeListener() {
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (hasFocus) {
hideKeyboard(AbstractActivity.this);
}
}
});
}
使用此通用活动(继承能力!)扩展您的活动,仅此而已,每次任何 EditText(在任何扩展活动上)失去焦点时,键盘将被隐藏。
PS hideKeyboard 方法:
public static void hideKeyboard(Activity context) {
InputMethodManager inputMethodManager = (InputMethodManager) context.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow( context.getCurrentFocus().getWindowToken(), 0);
}
context.getCurrentFocus() 不需要指定特定的 EditText 视图..
你可以试试下面的方法,它对我很有用:)
这种方式可以应用于Activity或者Fragment,也兼容ScrollView。
我们把 ScrollView 作为顶层布局,在里面为 LinearLayout 声明 id parentView 并添加如下两个属性:
android:id="@+id/parentView"
android:clickable="true"
android:focusableInTouchMode="true"
在代码中,编写如下函数:
public static void hideSoftKeyboard (Activity activity) {
InputMethodManager inputMethodManager = (InputMethodManager) activity.getSystemService(Activity.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(activity.getCurrentFocus().getWindowToken(), 0);
}
然后为根视图注册一个 OnFocusChangeListener (写入 onCreate 方法),使 Activity 中的所有 EditText 受到影响:
parentLayout.setOnFocusChangeListener(new View.OnFocusChangeListener() {
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (hasFocus) {
hideSoftKeyboard(your_activity_name.this);
}
}
});
我设法从onItemClick
AutoCompleteTextView
内部隐藏了键盘
public void onItemClick(AdapterView<?> adapterViewIn, View viewIn, int indexSelected, long arg3) {
InputMethodManager imm = (InputMethodManager) getSystemService(viewIn.getContext().INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(viewIn.getApplicationWindowToken(), 0);
// your code HERE
}
其他想法是在您的 Activity 的根视图上覆盖onInterceptTouchEvent
方法。
触摸事件从屏幕上最前面的视图(触摸事件发生的地方)向下传递到调用onTouch
方法的视图堆栈,直到任何视图返回 true,表示触摸事件已被使用。 由于许多视图默认使用触摸事件(例如EditText
或TextView
的情况),因此事件不会到达 Activity 的根视图onTouch
方法。
但是,在进行此遍历之前,触摸事件会经过另一条路径,从根视图沿着视图树向下直到到达最前面的视图。 这个遍历是通过调用onInterceptTouchEvent
来完成的。 如果该方法返回 true,它会拦截事件……不,但这有点小技巧,我认为您不想这样做,也不想知道细节。 您需要知道的是,您可以在 Activity 的根视图上覆盖此方法,并在必要时将代码放在那里隐藏键盘。
添加到@smit sonawane 的先前答案,如果用户正在滚动,此解决方案将不会隐藏键盘。
public long pressTime = 0;
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
if (ev.getAction() == MotionEvent.ACTION_DOWN) {
pressTime = System.currentTimeMillis();
}
else if (ev.getAction() == MotionEvent.ACTION_UP) {
long releaseTime = System.currentTimeMillis();
if (releaseTime-pressTime < 200) {
if (getCurrentFocus() != null) {
GhostTube.print("BottomNavActivity", "Touch event with keyboard detected...");
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
}
}
return super.dispatchTouchEvent(ev);
}
将代码添加到您的活动中,也会处理片段。
唯一有效的代码
private var viewHeight = 0
private fun setRootViewListener() {
binding.root.apply {
viewTreeObserver.addOnGlobalLayoutListener {
viewHeight = height
}
}
}
override fun dispatchTouchEvent(event: MotionEvent): Boolean {
currentFocus?.let {
if (it is EditText && event.y < viewHeight - it.measuredHeight) {
hideKeyboard(it)
}
}
return super.dispatchTouchEvent(event)
}
对于那些正在为此寻找 Xamarin 代码的人,请点击此处:
public override bool DispatchTouchEvent(MotionEvent ev)
{
try
{
View view = CurrentFocus;
if (view != null && (ev.Action == MotionEventActions.Up || ev.Action == MotionEventActions.Move) && view is EditText && !view.Class.Name.StartsWith("android.webkit."))
{
int[] Touch = new int[2];
view.GetLocationOnScreen(Touch);
float x = ev.RawX + view.Left - Touch[0];
float y = ev.RawY + view.Top - Touch[1];
if (x < view.Left || x > view.Right || y < view.Top || y > view.Bottom)
((InputMethodManager)GetSystemService(InputMethodService)).HideSoftInputFromWindow((Window.DecorView.ApplicationWindowToken), 0);
}
}
catch (System.Exception ex)
{
}
return base.DispatchTouchEvent(ev);
}
转到 Manifest 并在活动中写入:android:windowSoftInputMode="stateHidden",就像这样
<activity
android:name=".MainActivity"
android:exported="false"
android:windowSoftInputMode="stateHidden" />
我有这个问题的简单解决方案:
InputMethodManager inputMethodManager = (InputMethodManager) getActivity().getSystemService(Context.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(getActivity().getCurrentFocus().getWindowToken(),InputMethodManager.HIDE_NOT_ALWAYS);
setupUI((RelativeLayout) findViewById(R.id.activity_logsign_up_RelativeLayout));
将该方法传递到您的布局文件中。 您必须选择 XML 格式的通用布局文件。 因为,键盘隐藏适用于整个布局。
public void setupUI(View view) {
// Set up touch listener for non-text box views to hide keyboard.
if (!(view instanceof EditText)) {
view.setOnTouchListener(new View.OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
hideSoftKeyboard(Your Context); // Pass your context
return false;
}
});
}
//If a layout container, iterate over children and seed recursion.
if (view instanceof ViewGroup) {
for (int i = 0; i < ((ViewGroup) view).getChildCount(); i++) {
View innerView = ((ViewGroup) view).getChildAt(i);
setupUI(innerView);
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.