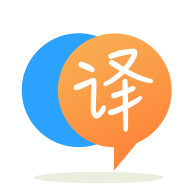
[英]How to change Tab from TabControl in WPF without violating the MVVM pattern
[英]How to implement a caching model without violating MVC pattern?
我有一个ASP.NET MVC 3(Razor)Web应用程序,其特定页面是高度数据库密集型的 ,并且用户体验是最重要的。
因此,我在这个特定的页面上引入了缓存。
我试图找到一种方法来实现这种缓存模式,同时保持我的控制器很薄 ,就像它目前没有缓存:
public PartialViewResult GetLocationStuff(SearchPreferences searchPreferences)
{
var results = _locationService.FindStuffByCriteria(searchPreferences);
return PartialView("SearchResults", results);
}
如您所见,控制器非常薄,应该如此。 它不关心如何/从哪里获取它的信息 - 这是服务的工作。
关于控制流程的几点注意事项:
IQueryable<T>
存储库并将结果具体化为T
或ICollection<T>
。 我想如何实现缓存:
[HttpPost]
从客户端(jQuery / AJAX)调用此操作方法,根据HTTP标准,不应将其作为请求缓存。 其次,我不想纯粹基于HTTP请求参数进行缓存 - 缓存逻辑比这复杂得多 - 实际上有两级缓存正在进行。 Cache["somekey"] = someObj;
。 首先想到的是告诉我创建另一个服务(继承LocationService ),并在那里提供缓存工作流(首先检查缓存,如果没有调用db,则添加到缓存,返回结果)。
这有两个问题:
System.Web
的引用。 我还考虑过使用Web应用程序中的Models
文件夹(我目前仅用于ViewModels ),但在模型文件夹中使用缓存服务听起来不对。
那么 - 任何想法? 是否有一个MVC特定的东西(比如Action Filter),我可以在这里使用吗?
一般建议/提示将不胜感激。
动作属性似乎是实现此目的的好方法。 这是一个例子(免责声明:我从头顶写这篇文章:我写这篇文章时已经消耗了一定数量的啤酒,所以一定要广泛测试:-)):
public class CacheModelAttribute : ActionFilterAttribute
{
private readonly string[] _paramNames;
public CacheModelAttribute(params string[] paramNames)
{
// The request parameter names that will be used
// to constitute the cache key.
_paramNames = paramNames;
}
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
base.OnActionExecuting(filterContext);
var cache = filterContext.HttpContext.Cache;
var model = cache[GetCacheKey(filterContext.HttpContext)];
if (model != null)
{
// If the cache contains a model, fetch this model
// from the cache and short-circuit the execution of the action
// to avoid hitting the repository
var result = new ViewResult
{
ViewData = new ViewDataDictionary(model)
};
filterContext.Result = result;
}
}
public override void OnResultExecuted(ResultExecutedContext filterContext)
{
base.OnResultExecuted(filterContext);
var result = filterContext.Result as ViewResultBase;
var cacheKey = GetCacheKey(filterContext.HttpContext);
var cache = filterContext.HttpContext.Cache;
if (result != null && result.Model != null && cache[key] == null)
{
// If the action returned some model,
// store this model into the cache
cache[key] = result.Model;
}
}
private string GetCacheKey(HttpContextBase context)
{
// Use the request values of the parameter names passed
// in the attribute to calculate the cache key.
// This function could be adapted based on the requirements.
return string.Join(
"_",
(_paramNames ?? Enumerable.Empty<string>())
.Select(pn => (context.Request[pn] ?? string.Empty).ToString())
.ToArray()
);
}
}
然后你的控制器动作可能如下所示:
[CacheModel("id", "name")]
public PartialViewResult GetLocationStuff(SearchPreferences searchPreferences)
{
var results = _locationService.FindStuffByCriteria(searchPreferences);
return View(results);
}
至于您在服务层中引用System.Web
程序集的问题,这不再是.NET 4.0中的问题。 有一个全新的程序集,它提供了可扩展的缓存功能: System.Runtime.Caching ,因此您可以使用它来直接在服务层中实现缓存。
或者甚至更好,如果您在服务层使用ORM,这个ORM可能提供缓存功能吗? 我希望如此。 例如,NHibernate提供二级缓存 。
我将提供一般性建议,希望他们能指出正确的方向。
如果这是您在应用程序中第一次尝试缓存,则不要缓存HTTP响应,而是缓存应用程序数据。 通常,您从缓存数据开始,并为您的数据库提供一些喘息空间; 那么,如果它还不够,你的app / web服务器承受着巨大的压力,你可以考虑缓存HTTP响应。
将您的数据缓存层视为MVC范例中的另一个模型,具有所有后续含义。
无论你做什么,都不要编写自己的缓存。 它总是看起来比实际更容易。 使用像memcached这样的东西。
我的答案基于您的服务实现接口的假设,例如_locationService的类型实际上是ILocationService,但是注入了具体的LocationService。 创建一个实现ILocationService接口的CachingLocationService,并更改容器配置以将该服务的缓存版本注入此控制器。 CachingLocationService本身对ILocationService有依赖性,它将使用原始的LocationService类注入。 它将使用它来执行真正的业务逻辑,并仅通过从缓存中拉取和推送来关注自身。
您不需要在与原始LocationService相同的程序集中创建CachingLocationService。 它可能在您的Web程序集中。 但是,我个人将它放在原始程序集中并添加新的引用。
至于添加对HttpContext的依赖; 你可以通过依赖来删除它
Func<HttpContextBase>
并在运行时注入此类似的东西
() => HttpContext.Current
然后在您的测试中,您可以模拟HttpContextBase,但是您可能无法在不使用TypeMock之类的情况下模拟Cache对象。
编辑:在进一步阅读.NET 4 System.Runtime.Caching命名空间时,您的CachingLocationService应该依赖于ObjectCache。 这是缓存实现的抽象基类。 然后,您可以使用System.Runtime.Caching.MemoryCache.Default注入它。
听起来你正试图缓存从数据库中获取的数据 。 以下是我处理这个问题的方法(我在许多开源MVC项目中看到的方法):
/// <summary>
/// remove a cached object from the HttpRuntime.Cache
/// </summary>
public static void RemoveCachedObject(string key)
{
HttpRuntime.Cache.Remove(key);
}
/// <summary>
/// retrieve an object from the HttpRuntime.Cache
/// </summary>
public static object GetCachedObject(string key)
{
return HttpRuntime.Cache[key];
}
/// <summary>
/// add an object to the HttpRuntime.Cache with an absolute expiration time
/// </summary>
public static void SetCachedObject(string key, object o, int durationSecs)
{
HttpRuntime.Cache.Add(
key,
o,
null,
DateTime.Now.AddSeconds(durationSecs),
Cache.NoSlidingExpiration,
CacheItemPriority.High,
null);
}
/// <summary>
/// add an object to the HttpRuntime.Cache with a sliding expiration time. sliding means the expiration timer is reset each time the object is accessed, so it expires 20 minutes, for example, after it is last accessed.
/// </summary>
public static void SetCachedObjectSliding(string key, object o, int slidingSecs)
{
HttpRuntime.Cache.Add(
key,
o,
null,
Cache.NoAbsoluteExpiration,
new TimeSpan(0, 0, slidingSecs),
CacheItemPriority.High,
null);
}
/// <summary>
/// add a non-removable, non-expiring object to the HttpRuntime.Cache
/// </summary>
public static void SetCachedObjectPermanent(string key, object o)
{
HttpRuntime.Cache.Remove(key);
HttpRuntime.Cache.Add(
key,
o,
null,
Cache.NoAbsoluteExpiration,
Cache.NoSlidingExpiration,
CacheItemPriority.NotRemovable,
null);
}
我在名为Current.cs
的静态类中有这些方法 。 以下是如何将这些方法应用于控制器操作:
public PartialViewResult GetLocationStuff(SearchPreferences searchPreferences)
{
var prefs = (object)searchPreferences;
var cachedObject = Current.GetCachedObject(prefs); // check cache
if(cachedObject != null) return PartialView("SearchResults", cachedObject);
var results = _locationService.FindStuffByCriteria(searchPreferences);
Current.SetCachedObject(prefs, results, 60); // add to cache for 60 seconds
return PartialView("SearchResults", results);
}
我已经接受了@Josh的答案,但我想我会添加自己的答案,因为我并没有完全按照他的建议去做(关闭),所以考虑到完整性,我会添加我实际做的。
关键是我现在正在使用System.Runtime.Caching
。 因为它存在于特定于.NET且不是ASP.NET特定的程序集中,所以在我的服务中引用它时没有问题。
所以我所做的就是将缓存逻辑放在需要缓存的特定服务层方法中。
重要的一点是,我正在使用System.Runtime.Caching.ObjectCache
类 - 这就是将get注入到服务的构造函数中。
我当前的DI注入了一个System.Runtime.Caching.MemoryCache
对象。 ObjectCache
类的ObjectCache
在于它是抽象的,所有核心方法都是虚拟的。
这意味着对于我的单元测试,我创建了一个MockCache
类,覆盖所有方法并使用简单的Dictionary<TKey,TValue>
实现底层缓存机制。
我们计划很快切换到Velocity - 所以我需要做的就是创建另一个ObjectCache
派生类,我很高兴。
感谢大家的帮助!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.