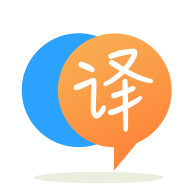
[英]How to determine if dll file was compiled as x64 or x86 bit using either Delphi or Lazarus
[英]How to use 32-bit DLL in x64 environment? It won't let me force x86 compile!
VC ++ 2008,CLR控制台应用程序,在win7 x64下开发。
我将MS Office v.12 PIA与.net结合使用来实现一些Excel自动化,而且进展非常顺利。 但是现在,我正在启动代码的下一部分,其中涉及做一些简单的电子邮件事务,所以我试图让MAPI在我的代码中工作。 基本上,它读取适当的注册表项以获取OLMAPI32.DLL文件的完整路径,然后尝试从该dll中加载LoadLibrary / GetProcAddress。
这是一个片段:
using namespace System;
using namespace Microsoft::Office::Interop;
using namespace Microsoft::Win32;
int main(array<System::String ^> ^args)
{
RegistryKey^ subK = Registry::LocalMachine->OpenSubKey("SOFTWARE\\Clients\\Mail\\Microsoft Outlook");
String^ mailDll = safe_cast<String^>(subK->GetValue("DLLPathEx"));
CStringW cStrMailDll = mailDll; //Just to put mailDll in a format that LoadLibrary() can read.
LPMAPIINITIALIZE mapiInit = NULL;
HMODULE mapiLib = LoadLibrary(cStrMailDll); // This Returns NULL
if(mapiLib == NULL)
{
printf("\nError: %d\n", GetLastError()); // System Error 193 - ERROR_BAD_EXE_FORMAT
return 1;
}
...(more code)
...
LoadLibrary设置系统错误193:“%1不是有效的Win32应用程序”,这对我来说并不震惊。 经过研究,我认为我所要做的只是强制x86编译。 因此,我转到Configuration Manager,在Active解决方案平台下,我唯一的选择是Win32,New和Edit。 因此,我单击“新建”,在“类型或选择新平台”下键入“ x86”,然后单击“复制设置来自”以选择“任何CPU”或其他合适的选项,但我唯一的选择是Win32,然后选择! 我想也许是因为我已经瞄准了.net,所以为了测试这个理论,我开始了一个新项目,这次是作为Win32控制台应用程序。 即使在这种类型的项目下,我唯一的选择是Win32。 我听说过的x86,x64,任何CPU和Itanium在我的VS2008中都不存在!
所以我在这里不知所措。 如何强制VS将exe编译为x86,以便可以使用mapi接口? 还是我可以使用64位版本的OLMAPI32.DLL? 如果没有64位库,如何使MAPI在我的代码中工作?当我尝试为x86设置环境时,VS给了我极大的帮助吗? 我简直不敢相信我的64位环境会自动使我失去使用MAPI的资格。
谢谢
我相信Win32在Visual Studio C ++中是x86
您可以通过usihg corflags强制使用32位CLR。 例如CorFlags.exe /32bit+ file.exe
有一个64位版本的MAPI与64位版本的MS Outlook一起提供。 此MSDN文章对其进行了详细描述。
所以,为了帮助那些帮助我的人,以及任何可能有类似问题并且发生在这个主题上的人,这就是我最终做的......
我放弃了纯MAPI路由,而是决定通过Outlook PIA。 所以现在我的代码看起来像这样:
#define nul Reflection::Missing::Value
#include "stdafx.h"
using namespace System;
using namespace Microsoft::Office::Interop;
int main(array<System::String ^> ^args)
{
// Create the outlook object
Outlook::Application^ olApp = gcnew Outlook::Application();
// Get an instance of the MAPI namespace via the outlook object
Outlook::NameSpace^ olNs = olApp->GetNamespace("MAPI");
// Log this instance into the MAPI interface
// Note that this will cause the user to be prompted for which profile
// to use. At least, it prompts me, and I only have one profile anyway. Whatever.
// Change the first argument to the name of the profile you want it to use (String)
// to bypass this prompt. I personally have mine set to "Outlook".
olNs->Logon(nul, nul, true, true);
// Get my Inbox
Outlook::Folder^ defFolder = safe_cast<Outlook::Folder^>(olNs->GetDefaultFolder(Outlook::OlDefaultFolders::olFolderInbox));
// Get all of the items in the folder (messages, meeting notices, etc.)
Outlook::Items^ fItems = defFolder->Items;
// Sort them according to when they were received, descending order (most recent first)
fItems->Sort("[ReceivedTime]", true);
// Show me the folder name, and how many items are in it
printf("\n%s: %d\n", defFolder->Name, fItems->Count);
// Make an array of _MailItems to hold the messages.
// Note that this is a _MailItem array, so it will only hold email messages.
// Other item types (in my case, meeting notices) cannot be added to this array.
array<Outlook::_MailItem^>^ mail = gcnew array<Outlook::_MailItem^>(fItems->Count);
int itemNum = 1;
int offset = 0;
// If there's anything in my Inbox, do the following...
if(fItems->Count)
{
// Try to grab the first email with "fItems->GetFirst()", and increment itemNum.
try
{
mail[itemNum++] = safe_cast<Outlook::_MailItem^>(fItems->GetFirst());
}
// If it threw an exception, it's probably because the item isn't a _MailItem type.
// Since nothing got assigned to mail[1], reset itemNum to 1
catch(Exception^ eResult)
{
itemNum = 1;
}
// Ok, now use "fItems->GetNext()" to grab the rest of the messages
for(; itemNum <= (fItems->Count-offset); itemNum++)
{
try
{
mail[itemNum] = safe_cast<Outlook::_MailItem^>(fItems->GetNext());
}
// If it puked, then nothing got assigned to mail[itemNum]. On the next iteration of
// this for-loop, itemNum will be incremented, which means that *this* particular index
// of the mail array would be left empty. To prevent this, decrement itemNum.
// Also, if itemNum ever gets decremented, then that will ultimately cause this loop
// to iterate more times than fItems->Count, causing an out-of-bounds error. To prevent
// that, increment "offset" each time you decrement "itemNum" to compensate for the
// offset.
catch(Exception^ eResult)
{
itemNum--;
offset++;
}
}
// Show me the money!
for(int i=1; i <= (fItems->Count-offset); i++)
printf("%d - %s\n", i, mail[i]->Subject);
}
olNs->Logoff();
return 0;
}
现在我知道如何进入,我可以继续完成我的项目! 谢谢各位的帮助!
干杯! d
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.