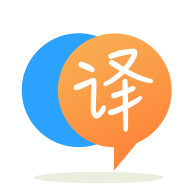
[英]Time complexity to convert a integer to string using itoa() function in c++?
[英]Convert a number from string to integer without using inbuilt function
我正在尝试这项技术,但错误即将来临。 请帮助我将数字从字符串转换为整数。
#include<iostream>
using namespace std;
int main()
{
char *buffer[80];
int a;
cout<<"enter the number";
cin.get(buffer,79);
char *ptr[80] = &buffer;
while(*ptr!='\0')
{
a=(a*10)+(*ptr-48);
}
cout<<"the value"<<a;
delete ptr[];
return 0;
}
错误是:
当将变量定义为“ char * buffer [80]”时,实际上是由80个char指针组成的数组,而不是由大小为80的数组组成。此外,您不应删除未使用new分配的任何内容(或delete [ ](在这种情况下,未分配给new []的任何内容)。
编辑:另一件事,您实际上并没有推进ptr,因此您将始终看着第一个字符。
正如@Tal所提到的,您正在创建char*
缓冲区,但是将它们视为char
缓冲区。 但是,推荐的C ++方法根本不使用原始缓冲区:
#include<iostream>
#include <string>
using namespace std;
int main()
{
string buffer;
int a = 0;
cout<<"enter the number";
cin >> buffer;
for(string::iterator it = buffer.begin(); it != buffer.end(); ++it)
{
a=(a*10) + (*it-48);
}
cout<<"the value"<<a;
return 0;
}
当然,可以将其缩短为:
#include<iostream>
using namespace std;
int main()
{
int a;
cout<<"enter the number";
cin >> a;
cout<<"the value"<<a;
}
但这已经使用了库函数。
编辑:还修复int a
未初始化的int a
。 这导致程序返回垃圾。
下面会有所帮助。 我在项目中使用这种方式。
bool stringToInt(std::string numericString, int *pIntValue)
{
int dVal = 0;
if (numericString.empty())
return false;
for (auto ch : numericString)
{
if (ch >= '0' && ch <= '9')
{
dVal = (dVal * 10) + (ch - '0');
}
else
{
return false;
}
}
*pIntValue = dVal;
return true;
}
int main()
{
int num = 0;
std::string numStr;
std::cin >> numStr;
if (!stringToInt(numStr, &num))
std::cout << "convertionFailed\n";
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.