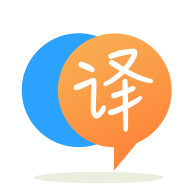
[英]Custom Settings-class exception on first use (ApplicationSettingsBase)
[英]ApplicationSettingsBase writes empty tag for custom collection
我已经断断续续地与这个作斗争了好几天了。 我需要将一组自定义对象存储为用户设置的一部分。 基于大量谷歌工作,似乎从ApplicationSettingsBase
构建首选项 class 是一种合适的方法。 我遇到的问题是,一旦我尝试存储自定义类型的集合,就没有为该属性保存任何数据。 如果我保持 collections 的基本类型(例如字符串),则一切正常。 我有一个单独的概念验证项目,过去一天我一直在使用它来隔离这个问题。
该项目由 WPF window 组成,带有一个列表框和两个按钮,“+”和“-”。 我有一个 Prefs class,其中三个属性定义了不同类型的 collections。 在我的 window 代码中,我将列表框绑定到这些列表之一,并且按钮可以在列表中添加或删除项目。 关闭 window 应该将列表的内容保存到当前用户的 users.config 文件中。 重新打开它应该显示列表的保存内容。
如果我使用 List1 ( ObservableCollection<string>
) 并单击 + 按钮几次然后关闭 window 数据正确保存到 user.config。 但是,如果我更改并使用 List2( ObservableCollection<Foo>
) 或 List3( FooCollection
) 我只会得到一个空值标签。 我试图实现ISerializable
和IXmlSerializable
以试图在不改变行为的情况下使其正常工作。 事实上,在带断点的调试模式下运行表明,当调用 save 方法时集合包含数据,但从不调用序列化接口方法。
我错过了什么?
更新:我已经改变了我的方法,暂时将数据写入 user.config 旁边的另一个文件,以便我可以在应用程序的其他部分取得一些进展。 但我仍然想知道为什么应用程序设置库无法记录我的收集数据。
这是所有相关代码,如果有人认为省略的部分很重要,我会将其添加回帖子中。
向每个列表属性添加几个元素后的 user.config
<setting name="List1" serializeAs="Xml">
<value>
<ArrayOfString xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<string>0</string>
<string>1</string>
<string>2</string>
</ArrayOfString>
</value>
</setting>
<setting name="List2" serializeAs="Xml">
<value />
</setting>
<setting name="List3" serializeAs="Xml">
<value />
</setting>
Window XAML
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition Width="Auto"></ColumnDefinition>
</Grid.ColumnDefinitions>
<ListBox Name="l1" Grid.Column="0" Grid.Row="0" ItemsSource="{Binding}"></ListBox>
<StackPanel Grid.Column="1" Grid.Row="0">
<Button Name="bP" Margin="5" Padding="5" Click="bP_Click">+</Button>
<Button Name="bM" Margin="5" Padding="5" Click="bM_Click">-</Button>
</StackPanel>
</Grid>
Window 的代码隐藏
public partial class Window1 : Window
{
Prefs Prefs = new Prefs();
public Window1()
{
InitializeComponent();
//l1.DataContext = Prefs.List1;
//l1.DataContext = Prefs.List2;
l1.DataContext = Prefs.List3;
}
private void Window_Closed(object sender, EventArgs e)
{
Prefs.Save();
}
private void bP_Click(object sender, RoutedEventArgs e)
{
//Prefs.List1.Add(Prefs.List1.Count.ToString());
//Prefs.List2.Add(new Foo(Prefs.List2.Count.ToString()));
Prefs.List3.Add(new Foo(Prefs.List3.Count.ToString()));
}
private void bM_Click(object sender, RoutedEventArgs e)
{
//Prefs.List1.RemoveAt(Prefs.List1.Count - 1);
//Prefs.List2.RemoveAt(Prefs.List2.Count - 1);
Prefs.List3.RemoveAt(Prefs.List3.Count - 1);
}
}
首选项 class
class Prefs : ApplicationSettingsBase
{
[UserScopedSettingAttribute()]
[DefaultSettingValueAttribute(null)]
public System.Collections.ObjectModel.ObservableCollection<string> List1
{
get
{
System.Collections.ObjectModel.ObservableCollection<string> Value = this["List1"] as System.Collections.ObjectModel.ObservableCollection<string>;
if (Value == null)
{
Value = new System.Collections.ObjectModel.ObservableCollection<string>();
this["List1"] = Value;
}
return Value;
}
}
[UserScopedSettingAttribute()]
[DefaultSettingValueAttribute(null)]
public System.Collections.ObjectModel.ObservableCollection<Foo> List2
{
get
{
System.Collections.ObjectModel.ObservableCollection<Foo> Value = this["List2"] as System.Collections.ObjectModel.ObservableCollection<Foo>;
if (Value == null)
{
Value = new System.Collections.ObjectModel.ObservableCollection<Foo>();
this["List2"] = Value;
}
return Value;
}
}
[UserScopedSettingAttribute()]
[DefaultSettingValueAttribute(null)]
public FooCollection List3
{
get
{
FooCollection Value = this["List3"] as FooCollection;
if (Value == null)
{
Value = new FooCollection();
this["List3"] = Value;
}
return Value;
}
}
}
富 Class
[Serializable()]
class Foo : System.ComponentModel.INotifyPropertyChanged, ISerializable, IXmlSerializable
{
private string _Name;
private const string PropName_Name = "Name";
public string Name
{
get { return this._Name; }
set
{
if (value != this._Name)
{
this._Name = value;
RaisePropertyChanged(Foo.PropName_Name);
}
}
}
public override string ToString()
{
return Name;
}
public Foo() { }
public Foo(string name)
{
this._Name = name;
}
#region INotifyPropertyChanged Members
/***Omitted for space***/
#endregion
#region ISerializable Members
public Foo(SerializationInfo info, StreamingContext context)
{
this._Name = (string)info.GetValue(Foo.PropName_Name, typeof(string));
}
public void GetObjectData(SerializationInfo info, StreamingContext context)
{
info.AddValue(Foo.PropName_Name, this._Name);
}
#endregion
#region IXmlSerializable Members
public System.Xml.Schema.XmlSchema GetSchema()
{
return null;
}
public void ReadXml(System.Xml.XmlReader reader)
{
reader.MoveToContent();
_Name = reader.GetAttribute(Foo.PropName_Name);
bool Empty = reader.IsEmptyElement;
reader.ReadStartElement();
if (!Empty)
{
reader.ReadEndElement();
}
}
public void WriteXml(System.Xml.XmlWriter writer)
{
writer.WriteAttributeString(Foo.PropName_Name, _Name);
}
#endregion
}
和 FooCollection class
[Serializable()]
class FooCollection : ICollection<Foo>, System.ComponentModel.INotifyPropertyChanged, INotifyCollectionChanged, ISerializable, IXmlSerializable
{
List<Foo> Items;
private const string PropName_Items = "Items";
public FooCollection()
{
Items = new List<Foo>();
}
public Foo this[int index]
{
/***Omitted for space***/
}
#region ICollection<Foo> Members
/***Omitted for space***/
#endregion
public void RemoveAt(int index)
{
/***Omitted for space***/
}
#region IEnumerable Members
/***Omitted for space***/
#endregion
#region INotifyCollectionChanged Members
/***Omitted for space***/
#endregion
#region INotifyPropertyChanged Members
/***Omitted for space***/
#endregion
#region ISerializable Members
public FooCollection(SerializationInfo info, StreamingContext context)
{
this.Items = (List<Foo>)info.GetValue(FooCollection.PropName_Items, typeof(List<Foo>));
}
public void GetObjectData(SerializationInfo info, StreamingContext context)
{
info.AddValue(FooCollection.PropName_Items, this.Items);
}
#endregion
#region IXmlSerializable Members
public System.Xml.Schema.XmlSchema GetSchema()
{
return null;
}
public void ReadXml(System.Xml.XmlReader reader)
{
XmlSerializer FooSerializer = new XmlSerializer(typeof(Foo));
reader.MoveToContent();
bool Empty = reader.IsEmptyElement;
reader.ReadStartElement();
if (!Empty)
{
if (reader.IsStartElement(FooCollection.PropName_Items))
{
reader.ReadStartElement();
while (reader.IsStartElement("Foo"))
{
this.Items.Add((Foo)FooSerializer.Deserialize(reader));
}
reader.ReadEndElement();
}
reader.ReadEndElement();
}
}
public void WriteXml(System.Xml.XmlWriter writer)
{
XmlSerializer FooSerializer = new XmlSerializer(typeof(Foo));
writer.WriteStartElement(FooCollection.PropName_Items);
foreach (Foo Item in Items)
{
writer.WriteStartElement("Foo");
FooSerializer.Serialize(writer, Item);
writer.WriteEndElement();//"Foo"
}
writer.WriteEndElement(); //FooCollection.PropName_Items
}
#endregion
}
我也遇到了类似的问题,我设法解决了这个问题,但我没有使用ObservableCollection
,而是使用普通的List<Column>
, Column
是我的 class ,它包含为公共成员:string、int 和 bool,它们是所有 xmlserializable。 List<>
,因为它实现了IEnumerable
也是XMLSerializable
。
在自定义 Foo Class 中,您唯一需要注意的是:您必须将成员设为公共、无参数构造函数,并且 class 本身必须是公共的。 您不需要为 xml 序列化添加[Serializable]
标签。
我不需要实现 FooCollection class,因为我使用的 List 与 xml 序列化没有问题。
另一件事:
ApplicationSettingsBase
的 class 可以内部密封 - 无需公开。在列表道具上方添加它是 xml 可序列化的事实:
[global::System.Configuration.UserScopedSettingAttribute()] [SettingsSerializeAs(SettingsSerializeAs.Xml)] [global::System.Configuration.DefaultSettingValueAttribute("")] public List Columns { get { return ((List)this["Columns" ]); } set { this["Columns"] = (List)value; } }
如果这不起作用,您还可以尝试实现TypeConverter
。
我也为一个非常相似的问题苦苦挣扎了两天,但现在我发现了一个可能对这里有所帮助的缺失链接。 如果您和我的情况实际上相似,那么“Foo”和“FooCollection”类需要明确公开!
我假设,在 List2( ObservableCollection<Foo>
) 和List3(FooCollection)
的情况下,.NET 会遇到IXmlSerializable
,这需要以某种方式进行显式公共访问(跨越自己程序集的边界)。
相反的选项 List1 ( ObservableCollection<string>
)似乎在一个扁平的字符串类型转换上运行,它对(内部)class 'Foo'感到满意......
(来自 ApplicationsettingsBase 文档: ApplicationSettingsBase
使用两种主要机制来序列化设置:1)如果存在可以转换为字符串和从字符串转换的TypeConverter
,我们使用它。 2)如果没有,我们回XmlSerializer
)
亲切的问候,鲁迪
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.