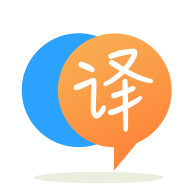
[英]Ruby/Rails - interate over complex (nested) JSON elements to create objects
[英]How to create complex Json response in ruby in rails
我正在研究 Rails 项目的 ruby,我想添加对 Json 的响应。
一种简单的方法是:--
def index
@users = User.all
respond_to do |format|
format.html # index.html.erb
format.xml { render :xml => @users }
format.json { render :json => @users.to_json }
end
end
但这有一些问题:-
所以问题是如何在 index.json.erb 文件中编码 json 响应,就像我们在 index.html.erb 中所做的那样。 根据需要格式化您自己的 json 响应。
编辑
def index
@users = User.all
respond_to do |format|
format.html # index.html.erb
format.xml { render :xml => @users }
format.json { render :file => "index.json.erb", :content_type => 'application/json' }
end
end
index.json.erb 文件:-
<% @users.each do |user| %>
{
first_name: <%= user.first_name %>,
last_name: <%= user.last_name %>
}
<% end %>
错误: -
模板丢失。
PS:--我只是尝试使用创建此文件。 这只是一个样本
编辑
编辑
{ { first_name: Mohit , last_name: Jain } { first_name: Sahil Miglani, last_name: } { first_name: Hitesh Bansal, last_name: } { first_name: Sudhanshu, last_name: } { first_name: Saakshi, last_name: } { first_name: Kutta, last_name: } { first_name: bc, last_name: } { first_name: hey, last_name: } { first_name: fewhjfbwfb, last_name: vdsv } }
编辑
[ { first_name: Mohit , last_name: Jain } , { first_name: Sahil Miglani, last_name: } , { first_name: Hitesh Bansal, last_name: } , { first_name: Sudhanshu, last_name: } , { first_name: Saakshi, last_name: } , { first_name: Kutta, last_name: } , { first_name: bc, last_name: } , { first_name: hey, last_name: } , { first_name: fewhjfbwfb, last_name: vdsv } ]
编辑,非常接近找出解决方案: -
[
<% @users.each_with_index do |user,index| %>
{
<%= "first_name".to_json.html_safe %>: <%= user.first_name.to_json.html_safe %>,
<%= "last_name".to_json.html_safe %>: <%= user.last_name.to_json.html_safe %>
}
<% unless index== @users.count - 1%>
,
<% end %>
<% end %>
]
回复:-
[
-
{
first_name: "Mohit "
last_name: "Jain"
}
-
{
first_name: "Sahil Miglani"
last_name: null
}
-
{
first_name: "Hitesh Bansal"
last_name: null
}
-
{
first_name: "Sudhanshu"
last_name: null
}
-
{
first_name: "Saakshi"
last_name: null
}
-
{
first_name: "Kutta"
last_name: null
}
-
{
first_name: "bc"
last_name: null
}
-
{
first_name: "hey"
last_name: null
}
-
{
first_name: "fewhjfbwfb"
last_name: "vdsv"
}
]
现在我想要的只是将每个响应部分包含在用户数组中
您可以通过实现 model 中的代码来自定义 output:
def as_json(options={})
super(:only => [:name, :email])
end
然后在您的 controller 中,您可以使用:
render :json => @user
有关详细信息,请参阅“ Rails to_json 或 as_json? ”。
您可以渲染 json.erb 文件并像普通模板一样使用它:
# file: app/views/your_controller/your_action.json.erb
{
filed1: <%= @some_var %>,
field2: <%= @another_var %>,
fieldN: <%= @yet_another_var %>,
data: <%= @some_data.to_json.html_safe %>
}
在您的 controller 中,使用content_type
调用显式渲染:
render :file => "your_file.json.erb", :content_type => 'application/json'
您可以发送 JSON 格式的选项:
:only
(明确的属性列表):except
(属性排除列表):methods
(执行的方法列表并包含它们的内容):include
(关系) 如果您想始终使用此 JSON output 格式,请将它们放在as_json
方法中(参见 Devin 的答案):
format.json { render :json => @users.to_json{:only=>[:name, :email, :id], :methods=>[:last_question_answered], :include=>[:profile] }
看一下rabl gem ,自定义您的视图:
# app/views/posts/index.rabl
collection @posts
attributes :id, :title, :subject
child(:user) { attributes :full_name }
node(:read) { |post| post.read_by?(@user) }
这将产生:
[{ post :
{
id : 5, title: "...", subject: "...",
user : { full_name : "..." },
read : true
}
}]
格式化是简单的部分,但我相信您所要求的,应该在 controller 中实现,而不是在您的视图中。
Refer to http://ar.rubyonrails.org/classes/ActiveRecord/Serialization.html#M000049 and see how you can use to_json
better to actually output just what you need rather than have the entire object serialized.
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.