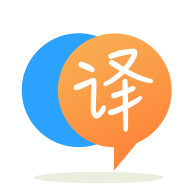
[英]Regular expression pattern to match url with or without http(s) and without tags
[英]Regular expression pattern to match URL with or without http://www
我一点也不擅长正则表达式。
迄今为止,我一直在使用很多框架代码,但我找不到能够匹配诸如http://www.example.com/etcetc
之类的 URL 的框架代码,但它也能够捕捉到一些东西像www.example.com/etcetc
和example.com/etcetc
。
为了匹配所有类型的 URL,下面的代码应该可以工作:
<?php
$regex = "((https?|ftp)://)?"; // SCHEME
$regex .= "([a-z0-9+!*(),;?&=$_.-]+(:[a-z0-9+!*(),;?&=$_.-]+)?@)?"; // User and Pass
$regex .= "([a-z0-9\-\.]*)\.(([a-z]{2,4})|([0-9]{1,3}\.([0-9]{1,3})\.([0-9]{1,3})))"; // Host or IP
$regex .= "(:[0-9]{2,5})?"; // Port
$regex .= "(/([a-z0-9+$_%-]\.?)+)*/?"; // Path
$regex .= "(\?[a-z+&\$_.-][a-z0-9;:@&%=+/$_.-]*)?"; // GET Query
$regex .= "(#[a-z_.-][a-z0-9+$%_.-]*)?"; // Anchor
?>
然后,检查正则表达式的正确方法如下:
<?php
if(preg_match("~^$regex$~i", 'www.example.com/etcetc', $m))
var_dump($m);
if(preg_match("~^$regex$~i", 'http://www.example.com/etcetc', $m))
var_dump($m);
?>
礼貌: splattermania在 PHP 手册中的评论:http: //php.net/manual/en/function.preg-match.php
在我测试过的所有情况下,这对我都有效:
$url_pattern = '/((http|https)\:\/\/)?[a-zA-Z0-9\.\/\?\:@\-_=#]+\.([a-zA-Z0-9\&\.\/\?\:@\-_=#])*/';
测试:
http://test.test-75.1474.stackoverflow.com/
https://www.stackoverflow.com
https://www.stackoverflow.com/
http://wwww.stackoverflow.com/
http://wwww.stackoverflow.com
http://test.test-75.1474.stackoverflow.com/
http://www.stackoverflow.com
http://www.stackoverflow.com/
stackoverflow.com/
stackoverflow.com
http://www.example.com/etcetc
www.example.com/etcetc
example.com/etcetc
user:pass@example.com/etcetc
example.com/etcetc?query=aasd
example.com/etcetc?query=aasd&dest=asds
http://stackoverflow.com/questions/6427530/regular-expression-pattern-to-match-url-with-or-without-http-www
http://stackoverflow.com/questions/6427530/regular-expression-pattern-to-match-url-with-or-without-http-www/
每个有效的 Internet URL 都至少有一个点,因此上述模式将简单地尝试查找由点链接的任何至少两个字符串,并且该 URL 可能具有有效的字符。
试试这个:
/^http:\\/\\/|(www\\.)?[a-z0-9]+([\\-\\.]{1}[a-z0-9]+)*\\.[az]{2,5}(:[0-9]{1,5})?(\\/.*)?$/
它完全按照人们想要的方式工作。
它需要或不带http://
、 https://
和www
。
您可以在正则表达式后使用问号使其有条件,因此您可以使用:
http:\/\/(www\.)?
这将匹配具有 http://www 的任何内容。 或 http://(没有 www.)
您可以使用替换方法删除上述内容,从而获得域。 这取决于您需要该域的用途。
利用:
/(https?://)?((?:(\w+-)*\w+)\.)+(?:[a-z]{2})(\/?\w?-?=?_?\??&?)+[\.]?([a-z0-9\?=&_\-%#])?/g
它匹配something.com
、 http(s)://
或www
。 虽然它与其他[something]://
URL 不匹配,但出于我的目的,这不是必需的。
正则表达式匹配例如:
http://foo.co.uk/
www.regex.com/foo.html?q=bar$some=thi-ng,regex
regex.foo.com/blog
尝试这样的事情:
.*([\w-]+\.)+[a-z]{2,5}(/[\w-]+)*
你可以试试这个:
r"(http[s]:\/\/)?([\w-]+\.)+([a-z]{2,5})(\/+\w+)? "
选择:
可以以 http:// 或 https:// 开头(可选)
任何(单词)都以点(。)结尾
后跟 2 到 5 个字符 [az]
后跟“/[anything]”(可选)
后跟空格
试试这个
$url_reg = /(ftp|https?):\/\/(\w+:?\w*@)?(\S+)(:[0-9]+)?(\/([\w#!:.?+=&%@!\/-])?)?/;
我一直在使用以下内容,它适用于我的所有测试用例,并修复了它会在句末以句号 ( end.
) 开头的句子结尾或有单个字符首字母的地方触发的任何问题,例如“CC 管道”。
以下正则表达式包含多个{2,}
,这意味着前一个模式的两个或多个匹配项。
((http|https)\:\/\/)?[a-zA-Z0-9\.\/\?\:@\-_=#]{2,}\.([a-zA-Z0-9\&\.\/\?\:@\-_=#]){2,}
匹配URL,例如但不限于:
不匹配非 URL,例如但不限于:
ab
或xy
请注意:由于上述原因,这不会匹配任何单个字符的 URL,例如: a.co
,但如果它前面有一个 URL 方案,它将匹配,例如: http://a.co
://a.co。
由于最近的 PHP 允许字符串中的$
并且 preg 匹配不起作用,我在从 anubhava 获得答案时遇到了很多问题。
这是我使用的:
// Regular expression
$re = '/((https?|ftp):\/\/)?([a-z0-9+!*(),;?&=.-]+(:[a-z0-9+!*(),;?&=.-]+)?@)?([a-z0-9\-\.]*)\.(([a-z]{2,4})|([0-9]{1,3}\.([0-9]{1,3})\.([0-9]{1,3})))(:[0-9]{2,5})?(\/([a-z0-9+%-]\.?)+)*\/?(\?[a-z+&$_.-][a-z0-9;:@&%=+\/.-]*)?(#[a-z_.-][a-z0-9+$%_.-]*)?/i';
// Match all
preg_match_all($re, $blob, $matches, PREG_SET_ORDER, 0);
// Print the entire match result
var_dump($matches);
// The first element of the array is the full match
这个 PHP Composer 包URL 亮点在 PHP 中做得很好:
<?php
use VStelmakh\UrlHighlight\UrlHighlight;
$urlHighlight = new UrlHighlight();
$matches = $urlHighlight->getUrls($string);
?>
如果它不必是正则表达式,您总是可以使用 PHP 中的验证过滤器。
filter_var('http://example.com', FILTER_VALIDATE_URL);
filter_var (混合$variable [, int $filter = FILTER_DEFAULT [, mixed $options ]]);
如果要确保 URL 以 HTTP/HTTPS 开头,则使用正则表达式:
https?:\/\/(www\.)?[-a-zA-Z0-9@:%._\+~#=]{1,256}\.[a-zA-Z0-9()]{1,6}\b([-a-zA-Z0-9()@:%_\+.~#?&//=]*)
如果您不需要 HTTP 协议:
[-a-zA-Z0-9@:%._\+~#=]{1,256}\.[a-zA-Z0-9()]{1,6}\b([-a-zA-Z0-9()@:%_\+.~#?&//=]*)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.