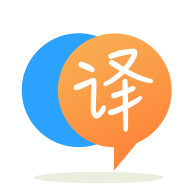
[英]How to split multi-level list of tuples into multi-level lists of values using LINQ?
[英]How to do multi-level sort on a List of a class with multiple variables
我有一个名为 Movies 的 class 声明了以下变量:
string Title;
string Plot;
string MPAA;
string Certification;
string[] Genres;
我使用以下代码创建了一个电影列表:
Movie m = new Movie(strTitle, strPlot, strMPAA, strCertification, strArrGenres);
MovieList.Add(m);
我现在正试图找出对列表进行排序的最佳方法。 我需要做两种排序,第一种是简单的按标题排序。 我尝试使用 LINQ,但我不知道如何正确访问电影中的变量。
第二个会更棘手。 我需要按类型然后标题排序。 当然,每部电影都可以有多个流派,而且我知道我最终会制作多部电影,因为这部电影将属于每个流派。
MovieList.OrderBy(m => m.Title)
和
MovieList.OrderBy(m => m.Genre).ThenBy(m => m.Title)
应该这样做。
如果您希望任一排序为降序,请使用.OrderByDescending()
和.ThenByDescending()
如果电影中包含流派,我不明白你想如何按流派排序,也许你想按流派过滤,然后按标题排序?
class Movie
{
public string Title { get; set; }
public string[] Genres { get; set; }
}
static void Main(string[] args)
{
var movies = new List<Movie>();
movies.Add(new Movie { Title = "Pulp Fiction", Genres = new string[] { "Crime", "Thriller" } });
movies.Add(new Movie { Title = "Back to the Future", Genres = new string[] { "Adventure", "Sci-Fi" } });
movies.Add(new Movie { Title = "The Dark Knight", Genres = new string[] { "Action", "Crime" } });
var byTitle = from m in movies orderby m.Title select m;
var crimeMovies = from m in movies where m.Genres.Contains("Crime") orderby m.Title select m;
}
编辑:选择具有流派的电影并按流派排序,然后按标题(根据评论):
var distinctGenres = from m in movies
from genre in m.Genres
group genre by genre into genres
select genres.First();
var moviesWithGenre = from g in distinctGenres
from m in movies
where m.Genres.Contains(g)
orderby g, m.Title
select new { Genre = g, Movie = m };
foreach (var m in moviesWithGenre)
{
Console.WriteLine("Genre: "+ m.Genre + " - " + m.Movie.Title);
}
Output:
Genre: Action - The Dark Knight
Genre: Adventure - Back to the Future
Genre: Crime - Pulp Fiction
Genre: Crime - The Dark Knight
Genre: Sci-Fi - Back to the Future
Genre: Thriller - Pulp Fiction
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.