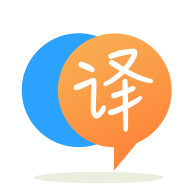
[英]Java - How to check if dynamically instantiated method is called at runtime?
[英]How To Check If A Method Exists At Runtime In Java?
go 如何检查 Java 中的 class 是否存在方法? try {...} catch {...}
语句会是一个好习惯吗?
我假设您想检查方法doSomething(String, Object)
。
你可以试试这个:
boolean methodExists = false;
try {
obj.doSomething("", null);
methodExists = true;
} catch (NoSuchMethodError e) {
// ignore
}
这将不起作用,因为该方法将在编译时解析。
您确实需要为此使用反射。 并且如果您可以访问您要调用的方法的源代码,那么最好为您要调用的方法创建一个接口。
[更新] 附加信息是:有一个接口可能存在两个版本,一个旧的(没有通缉的方法)和一个新的(有通缉的方法)。 基于此,我提出以下建议:
package so7058621;
import java.lang.reflect.Method;
public class NetherHelper {
private static final Method getAllowedNether;
static {
Method m = null;
try {
m = World.class.getMethod("getAllowedNether");
} catch (Exception e) {
// doesn't matter
}
getAllowedNether = m;
}
/* Call this method instead from your code. */
public static boolean getAllowedNether(World world) {
if (getAllowedNether != null) {
try {
return ((Boolean) getAllowedNether.invoke(world)).booleanValue();
} catch (Exception e) {
// doesn't matter
}
}
return false;
}
interface World {
//boolean getAllowedNether();
}
public static void main(String[] args) {
System.out.println(getAllowedNether(new World() {
public boolean getAllowedNether() {
return true;
}
}));
}
}
此代码测试接口中是否存在getAllowedNether
方法,因此实际对象是否具有该方法并不重要。
如果必须经常调用getAllowedNether
方法并且因此遇到性能问题,我将不得不考虑更高级的答案。 这个暂时应该没问题。
使用Class.getMethod(...)
函数时,反射 API 会抛出NoSuchMethodException
。
Otherwise Oracle has a nice tutorial about reflection http://download.oracle.com/javase/tutorial/reflect/index.html
在 java 中,这称为反射。 API 允许您发现方法并在运行时调用它们。 这是指向文档的指针。 这是非常冗长的语法,但它会完成工作:
http://java.sun.com/developer/technicalArticles/ALT/Reflection/
我将使用单独的方法来处理异常,并使用 null 检查方法是否存在
例如: if (null,= getDeclaredMethod(obj, "getId", null))
做你的事情......
private Method getDeclaredMethod(Object obj, String name, Class<?>... parameterTypes) {
// TODO Auto-generated method stub
try {
return obj.getClass().getDeclaredMethod(name, parameterTypes);
} catch (NoSuchMethodException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SecurityException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
如果您在项目中使用 Spring ,则可以检查ReflectionUtil.findMethod(..)
。 如果方法不存在或不符合您的要求,则返回 null。 文档。
Roland Illig 是正确的,但想添加一个示例,说明如何使用Class.getMethod检查是否存在需要参数的方法。 如果您尝试访问私有方法,也可以使用Class.getDeclaredMethod 。
class World {
public void star(String str) {}
private void mars(String str) {}
}
try {
World.class.getMethod("star", String.class);
World.class.getDeclaredMethod("mars", String.class);
} catch (Exception e) {}
其他解决方案:
public static <O> boolean existsMethod(final String methodName, final O out) {
return Stream.of(out.getClass().getMethods()).anyMatch(e -> methodName.equals(e.getName()));
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.