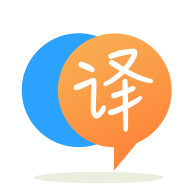
[英]Pagination in vanilla Javascript without any frameworks or libraries
[英]How to implement “prevUntil” in Vanilla JavaScript without libraries?
我需要在 Vanilla JavaScript 中实现 jQuery 的prevUntil()
方法的功能。
我在同一级别有几个<div>
元素:
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
我正在尝试使用onclick
事件来查找event.target
的previousSiblings
直到达到某个标准(例如,类名匹配)然后停止。
我如何实现这一目标?
此答案先前已在此处发布以回答类似问题。
有几种方法可以做到。
以下任一方法都可以解决问题。
// METHOD A (ARRAY.FILTER, STRING.INDEXOF)
var siblings = function(node, children) {
siblingList = children.filter(function(val) {
return [node].indexOf(val) != -1;
});
return siblingList;
}
// METHOD B (FOR LOOP, IF STATEMENT, ARRAY.PUSH)
var siblings = function(node, children) {
var siblingList = [];
for (var n = children.length - 1; n >= 0; n--) {
if (children[n] != node) {
siblingList.push(children[n]);
}
}
return siblingList;
}
// METHOD C (STRING.INDEXOF, ARRAY.SPLICE)
var siblings = function(node, children) {
siblingList = children;
index = siblingList.indexOf(node);
if(index != -1) {
siblingList.splice(index, 1);
}
return siblingList;
}
仅供参考:jQuery 代码库是观察 A 级 Javascript 的绝佳资源。
这是一个出色的工具,它以非常精简的方式展示了 jQuery 代码库。 http://james.padolsey.com/jquery/
使用 previousElementSibling 的示例:
var className = "needle";
var element = clickedElement;
while(element.previousElementSibling && element.previousElementSibling.className != className) {
element = element.previousElementSibling;
}
element.previousElementSibling; // the element or null
使用.children
与组合.parentNode
。 然后过滤NodeList
,将其转换为数组后: http://jsfiddle.net/pimvdb/DYSAm/ 。
var div = document.getElementsByTagName('div')[0];
var siblings = [].slice.call(div.parentNode.children) // convert to array
.filter(function(v) { return v !== div }); // remove element itself
console.log(siblings);
这个怎么样:
while ( node = node.previousElementSibling ) {
if ( ( ' ' + node.className + ' ' ).indexOf( 'foo' ) !== -1 ) {
// found; do your thing
break;
}
}
不要打扰告诉我这在 IE8 中不起作用......
HTML DOM 中有一个 previousSibling 属性
这里有一些参考
http://reference.sitepoint.com/javascript/Node/previousSibling
prevUntil: function( elem, i, until ) {
return jQuery.dir( elem, "previousSibling", until );
},
它使用名为 dir() 的 while / 循环函数。 prevUntil 会一直运行,直到previousSibling
与until
元素相同。
dir: function( elem, dir, until ) {
var matched = [],
cur = elem[ dir ];
while ( cur && cur.nodeType !== 9 && (until === undefined || cur.nodeType !== 1 || !jQuery( cur ).is( until )) ) {
if ( cur.nodeType === 1 ) {
matched.push( cur );
}
cur = cur[dir];
}
return matched;
},
您可以使用indexOf
来确定兄弟元素的索引是否小于目标元素的索引:
// jshint esversion: 9
// get the target node
const node = document.querySelector("div:nth-child(3)");
// get the target node's index
const node_index = node.parentNode.indexOf(node);
// get the siblings of the target node
const siblings = node => [...node.parentNode.children].filter(child =>
child !== node
);
console.log(siblings);
// get the prevUntil
const class_name = "\bmy_class\b";
const prevUntil = siblings.filter((sibling, i) =>
i < node_index && (sibling.getAttribute("class") || "").includes(class_name)
);
console.log(prevUntil);
祝你好运。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.