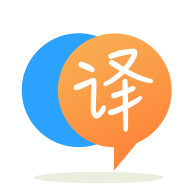
[英]Auto submit form every x seconds without page refresh and insert data into MYSQL?
[英]Write form data to MySQL without page refresh
我正在尝试创建一个页面,单击某个按钮时将显示一个表单。 然后,我希望用户将信息输入该表单,然后单击“提交”按钮。 我希望将这些信息提交给MySQL数据库,而不刷新/离开此页面。
我已经搜索了互联网,似乎必须使用AJAX和jQuery来执行此操作,但是问题是我完全不知道这两个。 我尝试遵循在几个站点上找到的示例和教程,但是我无法使用其中的任何一个。
我已经创建了表格。 它的代码如下。
<form name="classroom" method="post">
<fieldset>
<legend>Enter New MCP Information</legend>
<label for="date">Date:</label>
<input type="text" size="26" name="date" value="yyyy-mm-dd" onclick="this.value=''"onfocus="this.select()" onblur="this.value=!this.value?'yyyy-mm-dd':this.value;">
<p>
<label for="objective">Objective:</label>
<textarea name="objective" rows="3" cols="20" wrap="virtual"></textarea>
<p>
<label for="questions">Essential Questions:</label>
<textarea name="questions" rows="2" cols="20" wrap="virtual"></textarea>
<p>
<label for="criteria">Criteria for Success:</label>
<textarea name="criteria" rows="2" cols="20" wrap="virtual" onclick="this.value=''"onfocus="this.select()">Must be separated by commas.</textarea>
<p>
<label for="agenda">Agenda:</label>
<textarea name="agenda" rows="2" cols="20" wrap="virtual" onclick="this.value=''"onfocus="this.select()" >Must be separated by commas.</textarea>
<p class="submit"><input type="submit" class="submit" value="Submit"></p>
</fieldset>
</form>
下面是我用来编写表单数据的php脚本。 (我省去了所有的数据库连接和查询信息。一切正常。)
<?php
$day=addslashes($_POST['date']);
$objective=addslashes($_POST['objective']);
$questions=addslashes($_POST['questions']);
$criteria=addslashes($_POST['criteria']);
$agenda=addslashes($_POST['agenda']);
$connnect = mysql_connect("database","user","password");
if (!$connnect)
{
die('Could not connect: ' . mysql_error());
}
mysql_select_db("databasename") or die(mysql_error());
mysql_query("INSERT INTO mcp (Date, TPO, Questions, Criteria, Agenda)
VALUES ('$day', '$objective', '$questions', '$criteria', '$agenda')")
or die(mysql_error());
?>
您需要在表单提交事件之后运行ajax调用:
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript">
$(function() {
$('form').bind('submit', function(){
$.ajax({
type: 'post',
url: "/path-to-php-file",
data: $("form").serialize(),
success: function() {
alert("form was submitted");
}
});
return false;
});
});
</script>
现在,ajax调用将仅在提交表单时运行,而不是在页面开始时运行。
jQuery中的ajax()
方法将为您解决此问题:
<script type="text/javascript" src="path/to/jquery.js"></script>
<script type="text/javascript">
$(function() {
$.ajax({
type: 'POST',
url: "mypage.php",
data: $("FORM").serialize(),
success: function() {
alert("It worked!");
}
});
});
</script>
您只需要创建mypage.php
来处理帖子数据,然后将其插入数据库中即可。
通过以下链接获取有关.ajax()和.serialize()的更多信息
我不是网络开发人员,因此jQuery答案可能比这个更有意义。 但是,如果您愿意,这是一些不使用jQuery的页面的代码。
该页面具有一个带有单个textArea和一个按钮的表单。 与按钮相关联的onClick从textArea中获取值,并调用javascript函数创建对php页面的请求。
PHP页面运行数据库逻辑,并返回HTML格式的结果表。
然后,脚本将获取结果并替换表单下方的内容。
<html>
<head>
<script type="text/javascript">
function showResult(str)
{
if (str=="")
{
document.getElementById("txtHint").innerHTML="";
return;
}
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlhttp=new XMLHttpRequest();
}
else
{// code for IE6, IE5
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
xmlhttp.onreadystatechange=function()
{
if (xmlhttp.readyState==4 && xmlhttp.status==200)
{
document.getElementById("txtHint").innerHTML=xmlhttp.responseText;
}
else
{
document.getElementById("txtHint").innerHTML="ERROR";
}
}
xmlhttp.open("GET","getQuery.php?q="+str,true);
xmlhttp.send();
}
</script>
</head>
<body>
<form>
<textarea name="thequery" cols=60 rows=6>SHOW TABLES;</textarea>
<input type="button" value="Query" onClick="showResult(thequery.value)"/>
</form>
<br />
<div id="txtHint"><b>Query results will be listed here</b></div>
</body>
</html>
为了修复字符编码,我在PHP页面的输入中使用了以下行:
$query=$_GET["q"];
$query=stripslashes($query);
这是完整的PHP代码,用于运行任何SQL查询并返回结果的格式化表格。 可怕的安全性,但对测试有益。
<?php
/* set the database to connect to, the user, and the password */
$username="";
$password="";
$database="";
/* Here's the actual SQL query this page will run to get the data to display */
$query=$_GET["q"];
$query=stripslashes($query);
/* create a connection to the sql server with these credentials */
mysql_connect(localhost,$username,$password);
/* now connect to the specific database */
mysql_select_db($database) or die( "Unable to select database");
/* run the query we specified above - we get a "result set" */
$result=mysql_query($query);
if ($result) {
/* you can call some mysql functions on the result set to get information. */
/* here, we ask it how many rows were returned. */
$numrows=mysql_numrows($result);
/* ... and how many fields */
$numcols=mysql_num_fields($result);
/* This next block of code formats the result into a HTML table. */
/* Start by defining a table. Bad formatting practices, but whatever. */
print "<table width=600 border=1>\n";
/* print column headings in bold */
print "<tr>\n";
for ($i = 0; $i < $numcols; $i++) {
$colname = mysql_field_name($result, $i);
print "\t<td><b>$colname</b></td>\n";
}
print "</tr>\n";
/* Then fetch each row one-by-one and store it in $get_info.*/
while ($get_info = mysql_fetch_row($result)){
/* Everything between the { and } of the while loop will be run PER row */
/* So start a HTML table row */
print "<tr>\n";
/* Now loop over all "fields", or, columns */
/* this is the same as the while loop above, but now we take the
individual row and loop over its fields (as opposed to taking
the entire result set and looping over its rows)*/
foreach ($get_info as $field)
/* start HTML column, print the data and then close the column*/
print "\t<td>$field</td>\n";
/* And close the HTML table row */
print "</tr>\n";
/* End the while loop */
}
/* Close the HTML table */
print "</table>\n";
} else {
print 'Invalid Query: ' . mysql_error();
}
/* And close the connection */
mysql_close();
/* this ends our php script block, so everything after it shows up normally. */
?>
您可以使用jquery的ajax
和.serialize
$.ajax({
url:'/path',
type:'POST'
data:$("form").serialize(),
success:function(data){
//success handler code
},
error:function(jxhr){
console.log(jxhr.responseText);
}
});
是的,JavaScript是一个很好的答案。 我认为,如果您想在这种情况下使用jQuery是一个不错的选择,请搜索jQuery Form Plugin(http://jquery.malsup.com/form/),这是一个很大的帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.