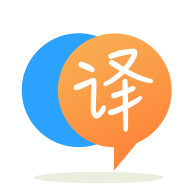
[英]How do I recursively and reflectively get a list of all possible field name paths?
[英]how do i list all possible directories and paths to subfolders ignoring files
我希望从根目录开始所有可能的文件夹路径,而忽略所有仅以文件结尾的路径。 getdirectories并未列出所有可能的路径。
例如:root:文件夹a和b
path a - c
path a - c - d
path a - c - x
path b - e
path b - e - f
path b - e - g
您需要递归地迭代并访问每个文件夹。 请参见此MSDN中的示例 。
Directory.GetDirectories()返回所有直接子级。
您需要使调用递归。 下面的伪代码-我目前无法访问计算机。
// Output All Leaf Nodes From Root
EnumerateDirectory(@"c:\");
public void EnumerateDirectory(string baseDirectory)
{
if( Directory.GetDirectories(baseDirectory).Count == 0 )
{
// This is an end, leaf node.
Output("This is a leaf node.");
return;
}
foreach(var directory in Directory.GetDirectories(baseDirectory) )
{
EnumerateDirectory(directory);
return;
}
}
没有理由编写递归函数。 您可以只使用重载之一。
oRoot.GetDirectories("*", SearchOption.AllDirectories);
这种方法解决了Strillo在评论Nick的答案时提到的问题。 效率也更高。
DirectoryInfo info = new DirectoryInfo(yourRoot);
foreach (var d in info.EnumerateDirectories("*",SearchOption.AllDirectories))
{
Console.WriteLine(d.FullName);
}
阅读此MSDN文档上的“备注”部分。
如果您要浏览的目录很多,并且需要跳过用户无权访问的目录,那么最好的选择是EnumerateDirectories :
// LINQ query.
var dirs = from dir in
Directory.EnumerateDirectories("C:\", "*", SearchOption.AllDirectories)
select dir;
// Show results.
foreach (var dir in dirs)
{
// Do something with the dir
}
private void ListAllDirectories(string root)
{
DirectoryInfo dr = new DirectoryInfo(root);
Console.WriteLine(dr.FullName);
var directories = dr.GetDirectories();
foreach (var directory in directories)
{
try
{
ListAllDirectories(directory.FullName);
}
catch
{
continue;
}
}
}
Catch用于处理未经授权的异常等,只是为了让您入门。
最后将我的VB.NET版本转换为C#:
namespace ExtensionMethods
{
public static class DirectoryExtensions
{
public static List<DirectoryInfo> GetSubFolders(this DirectoryInfo rootFolder)
{
if (rootFolder == null)
{
throw new ArgumentException("Root-Folder must not be null!", "rootFolder");
}
List<DirectoryInfo> subFolders = new List<DirectoryInfo>();
AddSubFoldersRecursively(rootFolder, ref subFolders);
return subFolders;
}
private static void AddSubFoldersRecursively(DirectoryInfo rootFolder, ref List<DirectoryInfo> allFolders)
{
try
{
allFolders.Add(rootFolder);
foreach (DirectoryInfo subFolder in rootFolder.GetDirectories())
{
AddSubFoldersRecursively(subFolder, ref allFolders);
}
}
catch (UnauthorizedAccessException exUnauthorized)
{
// go on
}
catch (DirectoryNotFoundException exNotFound)
{
// go on
}
catch (Exception ex)
{
throw;
}
}
}
}
如果有人对VB.NET版本感兴趣:
Public Module DirectoryExtensions
<Runtime.CompilerServices.Extension()>
Public Function GetSubFolders(ByVal rootFolder As DirectoryInfo) As List(Of DirectoryInfo)
If rootFolder Is Nothing Then
Throw New ArgumentException("Root-Folder must not be null!", "rootFolder")
End If
Dim subFolders As New List(Of DirectoryInfo)
AddSubFoldersRecursively(rootFolder, subFolders)
Return subFolders
End Function
Private Sub AddSubFoldersRecursively(rootFolder As DirectoryInfo, ByRef allFolders As List(Of DirectoryInfo))
Try
allFolders.Add(rootFolder)
For Each subFolder In rootFolder.GetDirectories
AddSubFoldersRecursively(subFolder, allFolders)
Next
Catch exUnauthorized As UnauthorizedAccessException
' go on '
Catch exNotFound As DirectoryNotFoundException
' go on '
Catch ex As Exception
Throw
End Try
End Sub
End Module
经过测试:
Dim result = Me.FolderBrowserDialog1.ShowDialog()
If result = Windows.Forms.DialogResult.OK Then
Dim rootPath = Me.FolderBrowserDialog1.SelectedPath
Dim rootFolder = New DirectoryInfo(rootPath)
Dim query = From folder In rootFolder.GetSubFolders()
Select folder.FullName
If query.Any Then
Me.ListBox1.Items.AddRange(query.ToArray)
End If
End If
这将处理DirectoryNotFoundException
和UnauthorizedAccessException
:
IEnumerable<string> GetFoldersRecursive(string directory)
{
var result = new List<string>();
var stack = new Stack<string>();
stack.Push(directory);
while (stack.Count > 0)
{
var dir = stack.Pop();
try
{
result.AddRange(Directory.GetDirectories(dir, "*.*"));
foreach (string dn in Directory.GetDirectories(dir))
{
stack.Push(dn);
}
}
catch
{
}
}
return result;
}
请注意,由于try
/ catch
块,此方法的性能可能不如其他方法。
* 免责声明:我最初并没有编写此代码(在SO上找到它!),我只是为了满足需要对其进行了修改。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.