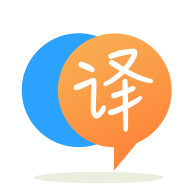
[英]How should I implement a copy constructor & assignment operator for a matrix class?
[英]How should I write a copy constructor in case of Singleton class and how should I overload = operator for same?
我应该如何为我的单例类编写一个复制构造函数,以防止创建一个新对象,因为我已经有了一个。 什么是overload = operator的最佳实践
#include <iostream>
#include <stdio.h>
#include <conio.h>
using namespace std;
class Rect
{
int length;
int breadth;
static int count;
static int maxcount;
Rect()
{};
Rect(const Rect& abc){};
public :
~Rect();
int area_rect()
{return length*breadth;}
void set_value(int a,int b);
static Rect* instance()
{
Rect* ptr=NULL;
if(count < maxcount)
{
ptr=new Rect ;
count++;
}
return ptr;
}
};
int Rect::count = 0;
int Rect::maxcount = 1;
void Rect::set_value(int a,int b)
{
length=a;
breadth=b;
}
Rect::~Rect()
{
count --;
}
int main()
{
Rect* a= Rect::instance(); //creates first object
// Rect* c= Rect::instance(); //fails to create second object
//as maxcount=1 and returns NULL
a->set_value(10,3);
cout << "area realted to object a : " << a->area_rect() <<"\n";
Rect* b=a;//allows creation of second object which I dont want
b->set_value(10,4);
cout << "area realted to object b : " << b->area_rect() <<"\n";
delete a;
delete b;
getch();
return 0;
}
如何编写复制构造函数代码并重载相等的运算符以防止创建更多对象?
要么使其不可复制,如此处所述
或者您定义复制构造函数和赋值运算符,以便获得相同的单例。 取决于您实际需要的功能。
通常也应该禁止分配(如上面的链接):
class Rect{
Rect( const Rect& ) = delete;
Rect& operator=( const Rect& ) = delete;
. . .
}
这也禁止移动操作。
你可能也想知道这一点: 单身人士真的那么糟糕吗?
单身人士很荒谬,只需使用免费功能。
仍然,回答你的问题......
C ++ 11:
class Foo {
public:
Foo (const Foo &) = delete;
Foo & operator = (const Foo &) = delete;
};
C ++ 03;
class Foo {
private:
// Don't write bodies.
Foo (const Foo &);
Foo & operator = (const Foo &);
};
以下是相关答案:如果您不希望有人创建对象,请不要给他们公共构造函数!
说真的,你可以拥有一个工厂对象,使它成为你想要限制构造的类的朋友,并使该类的构造函数成为私有的。 这样,访问对象的唯一方法(此时甚至不必创建它)将通过您的工厂。
例如
#include <boost/noncopyable.hpp>
#include <string>
class ConnectionFactory;
class DatabaseConnection : boost::noncopyable {
// can only be created by ConnectionFactory which happens to
// know how to connect to our database server!
friend class ConnectionFactory;
DatabaseConnection(std::string username, std::string password /*etc*/);
// don't want any random user to reset the connection!
~DatabaseConnection();
public:
// public interface bits
void Execute(const Select&);
// ...
};
ConnectionFactory {
public:
DatabaseConnection& conn();
};
class MayNeedDbConnection {
ConnectionFactory factory;
public:
explicit MayNeedDbConnection(const ConnectionFactory& f) : factory(f)
{}
void SelectStuff() {
DatabaseConnection& conn = factory.conn();
conn.Execute(....);
}
};
int main()
{
ConnectionFactory factory;
MayNeedDbConnection md(factory);
md.SelectStuff();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.