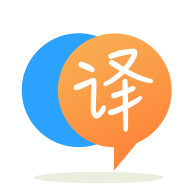
[英]Java code to display expiration date of certificates in a Java KeyStore
[英]Checking certificates expiration dates in java keystore
我的 java 应用程序使用一个密钥库文件,其中我有一个证书,该证书用于与活动目录服务器的 ssl 连接。 我要做的是检查它的到期日期并提示用户它是否接近到期。 我必须在我的应用程序启动时这样做。 我的想法是使用外部程序:keytool 在密钥库中显示有关某些证书的信息,然后对 keytool 输出的字符串进行一些解析操作以查找此验证日期。
这是特定 keytool 命令的输出:
Owner:
Issuer: CN=CPD Root CA, DC=cpd, DC=local<br>
Serial number: 39e8d1610002000000cb
<br>Valid from: Wed Feb 22 21:36:31 CET 2012 until: Thu Feb 21 21:36:31 CET 2013
Certificate fingerprints: <br>
MD5: 82:46:8B:DB:BC:5C:64:21:84:BB:68:E3:4B:D4:35:70<br>
SHA1: 35:52:CA:F2:11:66:1E:50:63:BC:53:A5:50:C1:F0:1E:62:81:BC:3F<br>
Signature algorithm name: SHA1withRSA
问题在于解析日期,因为我无法确定它以哪种格式显示。
有没有更简单的方法来检查 java 密钥库文件中包含的证书的到期日期?
感谢 EJP 的指导,这是我想出的一个块。
try {
KeyStore keystore = KeyStore.getInstance(KeyStore.getDefaultType());
keystore.load(new FileInputStream("keystoreLocation"), "keystorePassword".toCharArray());
Enumeration<String> aliases = keystore.aliases();
while(aliases.hasMoreElements()){
String alias = aliases.nextElement();
if(keystore.getCertificate(alias).getType().equals("X.509")){
System.out.println(alias + " expires " + ((X509Certificate) keystore.getCertificate(alias)).getNotAfter());
}
}
} catch (Exception e) {
e.printStackTrace();
}
使用 java.security.Keystore 类加载密钥库并枚举其内容,并检查每个证书是否过期。
字符串秘密 = "secret123";
InputStream ins = new FileInputStream("/test.jks");
KeyStore keyStore = KeyStore.getInstance("JKS");
keyStore.load(ins, secret.toCharArray());
枚举别名 = keyStore.aliases();
而(别名。hasMoreElements()){
String alias = aliases.nextElement();
System.out.println("alias : " + alias);
if(keyStore.getCertificate(alias).getType().equals("X.509")) {
X509Certificate cert = (X509Certificate) keyStore.getCertificate(alias);
System.out.println(alias + " from " + cert.getNotBefore());
System.out.println(alias + " to " + cert.getNotAfter());
try {
cert.checkValidity();
} catch (CertificateExpiredException e) {
// if the certificate has expired.
e.printStackTrace();
} catch (CertificateNotYetValidException e) {
// if the certificate is not yet valid. it means the certificate validitity start date is future date.
e.printStackTrace();
}
}
}
这是我编写的一个 Powershell 脚本,用于检查 JKS、提取别名和到期日期,并通过电子邮件向我发送一份表格,其中列出了在接下来的 60 天内到期的证书(可根据需要调整)。 我已安排在每个月的 1 日在任务计划程序中运行它
它分为两个阶段:
CMD 存在的原因是,当您通过 CMD 使用命令时,它会提取所需的数据并使用 LF 和 CRLF 来分离证书的数据 - 这样就可以很容易地将这些行连接在一起以用于 Powershell。 通过 Powershell 脚本运行命令只会创建 CRLF 换行符
PS:我是初学者 Powershell 编码器,所以请温柔:) 但有更多经验的人请随时优化此代码
这是澳大利亚时间,符合标准 (AEST) 和夏令时 (AEDT)
命令:
D:\path_to_java\jvm\jvm\bin\keytool.exe -list -v -keystore D:\path_to_JKS\CertStore.jks -storepass "my_jks_pass" | findstr "Alias until" > D:\Tools\Scripts\CertExpiryCheck\certlist_temp.txt
电源外壳:
# MUST use CMD to run below command line so that it creates both LF and CRLF breaks (Powershell just creates CRLF)
Start-Process -NoNewWindow -Wait -FilePath "C:\Windows\System32\cmd.exe" -ArgumentList {/c "D:\Tools\Scripts\CertExpiryCheck\Extract_cert_info.cmd"}
$original_file ='D:\Tools\Scripts\CertExpiryCheck\certlist_temp.txt'
$new_file1 ='D:\Tools\Scripts\CertExpiryCheck\new_certs1.txt'
$new_file2 ='D:\Tools\Scripts\CertExpiryCheck\certlist.txt'
$to = "myemail@domain.com"
$add_days = 60
$fileexists = Test-Path -Path $original_file
$checkiffileisempty = (Get-ChildItem -Path $original_file).Length -eq "0"
If (($fileexists -eq $False) -or ($checkiffileisempty -eq $True))
{
Write-Host "$original_file does not exist or is empty !"
break
}
#replace CRLF with space
$text1 = [IO.File]::ReadAllText($original_file) -replace "`r`n", " "
[IO.File]::WriteAllText($new_file1, $text1)
#replace LF with CRLF
$text2 = [IO.File]::ReadAllText($new_file1) -replace "`n", "`r`n"
[IO.File]::WriteAllText($new_file2, $text2)
$InXDays = [DateTime]::Now.AddDays($add_days)
# Create a DataTable
$table = New-Object system.Data.DataTable "ExpiringCertsTable"
$col1 = New-Object system.Data.DataColumn CertAlias,([string])
$col2 = New-Object system.Data.DataColumn ExpiringOn,([string])
$table.columns.add($col1)
$table.columns.add($col2)
$File = Get-Content $new_file2
foreach($line in $File)
{
$alias1, $until1 = $line -Split "Valid from:"
$aliastext, $certname = $alias1 -Split ":"
$from1, $untildate = $until1 -Split "until: "
if($untildate.Contains("AEDT"))
{
$cert_exp_date = [datetime]::ParseExact($untildate, 'ddd MMM dd HH:mm:ss AEDT yyyy', [cultureinfo]'en-AU')
}
if($untildate.Contains("AEST"))
{
$cert_exp_date = [datetime]::ParseExact($untildate, 'ddd MMM dd HH:mm:ss AEST yyyy', [cultureinfo]'en-AU')
}
if($cert_exp_date -le $InXDays )
{
Write-Output ">>> $certname <<<`thas expired or will be expiring on `t*** $untildate ***"
$row = $table.NewRow()
$row.CertAlias = $certname
$row.ExpiringOn = $untildate
$table.Rows.Add($row)
}
}
# Create column headers of Table
$HtmlTable1 = "<table border='1' align='Left' cellpadding='2' cellspacing='0' style='color:black;font-family:arial,helvetica,sans-serif;text-align:left;'>
<tr style ='font-size:13px;font-weight: normal;background-color: LightBlue'>
<th align=left><b>Certificate Alias</b></th>
<th align=left><b>Expiring On</b></th>
</tr>"
# Insert data into Table
foreach ($row in $table.Rows)
{
$HtmlTable1 += "<tr style='font-size:13px;background-color:#FFFFFF'>
<td>" + $row[0] + "</td>
<td>" + $row[1] + "</td>
</tr>"
}
$HtmlTable1 += "</table>"
#email report
$smtpserver = "smtp.server.com"
$from = "$env:COMPUTERNAME@domain.com.au"
$subject = "Certs expiring in next $add_days days on $env:COMPUTERNAME"
$body = "List of certs in D:\path_to_JKS\CertStore.jks expiring in the next $add_days days:<br /><br />" + $HtmlTable1
Send-MailMessage -smtpserver $smtpserver -from $from -to $to -subject $subject -body $body -bodyashtml
#clean up temp files
Remove-Item $original_file
Remove-Item $new_file1
Remove-Item $new_file2
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.