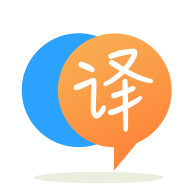
[英]Check that no option was explicitly selected in a select box without using jquery?
[英]Check if an option exist in select element without JQuery?
不幸的是,我无权访问 JQuery,而且这一切都很好。 但我确实可以访问 JavaScript。 如何检查 HTML 选择中是否存在 OPTION?
编辑:为了澄清,我需要知道是否存在选项。 例如:
<select>
<option>Volvo</option>
<option>Saab</option>
<option>Mercedes</option>
<option>Audi</option>
</select>
我检查“现代”是否是一个选项,它不是。
document.getElementById("myselect").options[0] //accesses first option via options[]
将在您的选择中选择第一个选项。 如果失败,您就知道您的选择中没有选项。 如果您通过在.options[0]
之后附加.value
获取数据,则它不为空。 如果没有 javascript,您将无法实现这一目标。 只有 HTML 不能提供您想要的功能。
for (i = 0; i < document.getElementById("myselect").length; ++i){
if (document.getElementById("myselect").options[i].value == "Hyndai"){
alert("Hyndai is available");
}
}
我今天遇到了这个问题,并使用这些答案提出了我自己的答案,我认为它更容易使用。
我遍历select
的选项(缓存长度),但我通过它的原型将该循环放入 HTMLSelectElement 本身,作为.contains()
函数。
HTMLSelectElement.prototype.contains = function( value ) {
for ( var i = 0, l = this.options.length; i < l; i++ ) {
if ( this.options[i].value == value ) {
return true;
}
}
return false;
}
然后要使用它,我只是这样写:
if ( select.contains( value ) ) {
我知道已经有一个选择的答案,但对于其他人从搜索到这里,我相信可以改进接受的答案,例如缓存“myselect”的选择。
我认为将逻辑包装在一个可重用的函数中并将其传递给您正在寻找的选项和对象将是有意义的:
/**
* Return if a particular option exists in a <select> object
* @param {String} needle A string representing the option you are looking for
* @param {Object} haystack A Select object
*/
function optionExists ( needle, haystack )
{
var optionExists = false,
optionsLength = haystack.length;
while ( optionsLength-- )
{
if ( haystack.options[ optionsLength ].value === needle )
{
optionExists = true;
break;
}
}
return optionExists;
}
用法:
optionExists( 'searchedOption', document.getElementById( 'myselect' ) );
我正在寻找比我更好的解决方案:
[...document.querySelector("select").options].map(o => o.value).includes("Hyndai")
另一种可能性是利用Array.prototype.find
:
/**
* @param {HTMLSelectElement} selectElement
* @param {string} optionValue
* @return {boolean}
*/
function optionExists(selectElement, optionValue) {
return !!Array.prototype.find.call(selectElement.options, function(option) {
return option.value === optionValue;
}
)
因为如果选择当前不存在,我将向该选择添加一个选项,所以我只需将选择的值设置为要检查是否存在的项目,然后读取该元素的 selectedIndex 属性。 如果它是 -1,则该选项当前不存在于控件中。
<select id="mySelect">
<option>Apple</option>
<option>Orange</option>
<option>Pineapple</option>
<option>Banana</option>
</select>
<script>
function myFunction() {
document.getElementById("mySelect").value = "Banana";
alert(document.getElementById("mySelect").selectedIndex);
//yields 3
document.getElementById("mySelect").value = "Strawberry";
alert(document.getElementById("mySelect").selectedIndex);
//yields -1
}
</script>
value
属性使用HTMLOptionElement
对象上的value
属性检查是否存在具有指定 value 属性的选项。 请注意,如果未提供此类属性,则选项的值将回退到其文本内容。
const select = document.querySelector("select");
const optionLabels = Array.from(select.options).map((opt) => opt.value);
const hasOption = optionLabels.includes("Hyndai");
<option>
显示文本要检查是否存在带有指定显示文本的选项,而不管其value
属性如何,请使用HTMLOptionElement
对象上的text
属性。 这两个片段之间的唯一区别是第二行的结尾。
const select = document.querySelector("select");
const optionLabels = Array.from(select.options).map((opt) => opt.text);
const hasOption = optionLabels.includes("Hyndai");
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.