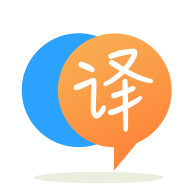
[英]How to write Nunit test for a method which passes an object (C#)
[英]How write stub method with NUnit in C#
我有2节课:
SecondDeep.cs
我做了简单的代码,例如:
class FirstDeep
{
public FirstDeep() { }
public string AddA(string str)
{
SecondDeep sd = new SecondDeep();
bool flag = sd.SomethingToDo(str);
if (flag == true)
str = string.Concat(str, "AAA");
else
str = string.Concat(str, "BBB");
return str;
}
}
和
class SecondDeep
{
public bool SomethingToDo(string str)
{
bool flag = false;
if (str.Length < 10)
{
//todo something in DB, and after that flag should be TRUE
}
return flag;
}
}
然后,我想为方法“ AddA”编写单元测试:
class Tests
{
[Test]
public void AddATest()
{
string expected = "ABCAAA";
FirstDeep fd = new FirstDeep();
string res = fd.AddA("ABC");
Assert.AreEqual(expected, res);
}
}
之后,我遇到了麻烦,我不知道在Test类中为方法SomethingToDo编写正确的存根。 我总是有假的。 我应该返回TRUE。 但是如何?
允许您编写存根的一个好方法是使用依赖注入 。 FirstDeep
取决于SecondDeep
,在测试中,您想用存根替换SecondDeep
。
首先通过提取用于SecondDeep
的接口来更改现有代码,然后将其注入构造函数中的FirstDeep
中:
interface ISecondDeep {
Boolean SomethingToDo(String str);
}
class SecondDeep : ISecondDeep { ... }
class FirstDeep {
readonly ISecondDeep secondDeep;
public FirstDeep(ISecondDeep secondDeep) {
this.secondDeep = secondDeep;
}
public String AddA(String str) {
var flag = this.secondDeep.SomethingToDo(str);
...
}
}
请注意, FirstDeep
不再创建SecondDeep
实例。 而是将一个实例注入构造函数中。
在测试中,您可以为ISecondDeep
创建存根,其中SomethingToDo
始终返回true:
class SecondDeepStub : ISecondDeep {
public Boolean SomethingToDo(String str) {
return true;
}
}
在测试中,您使用存根:
var firstDeep = new FirstDeep(new SecondDeepStub());
在生产代码中,使用“真实的” SecondDeep
:
var firstDeep = new FirstDeep(new SecondDeep());
使用依赖项注入容器和存根框架可以使很多操作变得更容易。
如果您不想重写代码,则可以使用诸如Microsoft Moles之类的框架来拦截调用。 在Visual Studio的下一版本中,类似的技术将在Fakes Framework中可用。
为了使您的代码可测试,请不要在类内部实例化依赖关系。 使用依赖注入 (通过构造函数,属性或参数)。 还可以使用抽象类或接口来模拟依赖项:
class FirstDeep
{
private ISecondDeep oa;
public FirstDeep(ISecondDeep oa)
{
this.oa = oa;
}
public string AddA(string str)
{
return String.Concat(str, oa.SomethingToDo(str) ? "AAA" : "BBB");
}
}
依赖于抽象允许您隔离测试类。
interface ISecondDeep
{
bool SomethingToDo(string str);
}
class SecondDeep : ISecondDeep
{
public bool SomethingToDo(string str)
{
bool flag = false;
if (str.Length < 10)
{
// without abstraction your test will require database
}
return flag;
}
}
这是测试样本(使用Moq )。 它显示了如何从调用返回true
到模拟的依赖项:
[TestFixture]
class Tests
{
[Test]
public void AddAAATest()
{
// Arrange
Mock<ISecondDeep> secondDeep = new Mock<ISecondDeep>();
secondDeep.Setup(x => x.SomethingToDo(It.IsAny<string>())).Returns(true);
// Act
FirstDeep fd = new FirstDeep(secondDeep.Object);
// Assert
Assert.That(fd.AddA("ABD"), Is.EqualTo("ABCAAA"));
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.