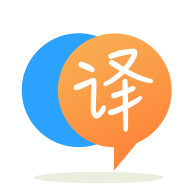
[英]In WPF, access a control that is instantiated in a XAML file from a separate Class?
[英]Load control style from separate file in wpf
我在 Windows.Resources 中添加了以下样式
<Window.Resources>
...
<!--A Style that extends the previous TextBlock Style-->
<!--This is a "named style" with an x:Key of TitleText-->
<Style BasedOn="{StaticResource {x:Type TextBlock}}"
TargetType="TextBlock"
x:Key="TitleText">
<Setter Property="FontSize" Value="26"/>
<Setter Property="Foreground">
<Setter.Value>
<LinearGradientBrush StartPoint="0.5,0" EndPoint="0.5,1">
<LinearGradientBrush.GradientStops>
<GradientStop Offset="0.0" Color="#90DDDD" />
<GradientStop Offset="1.0" Color="#5BFFFF" />
</LinearGradientBrush.GradientStops>
</LinearGradientBrush>
</Setter.Value>
</Setter>
</Style>
...
</Window.Resources>
我的 xaml 代码中有很多这些样式,我想将每个组件样式保存到一个额外的文件(而不是外部文件)中。例如,与 TextBlocks 相关的所有样式都应该在一个名为TextBlockStyles.xaml
的文件中
我将如何在 wpf 中做到这一点?
如何链接项目中的样式?
提前致谢
您使用合并的资源字典
在您的 app.xaml 中,您将使用
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary
Source="/Your.Assembly.Name;component/TextBlockStyles.xaml"/>
... other dictionaries here
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
或直接进入 UserControl 将是
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary
Source="/Your.Assembly.Name;component/TextBlockStyles.xaml"/>
... other dictionaries here
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
如果文件在同一个程序集和项目的根目录中,您可以将Source="..."
缩短为仅Source="TextBlockStyles.xaml"
,或者如果您将Source="Styles\\TextBlockStyles.xaml"
放在资源字典放入文件夹Styles
。
用例:您有一个名为MyView.xaml
的用户控件,带有一个按钮。 您想使用外部 XAML 文件设置按钮样式。
在MyView.xaml
:
<User Control ...namespaces...>
<UserControl.Resources>
<ResourceDictionary>
...converters...
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="MyButton.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
...the rest of the control...
</UserControl>
在MyButton.xaml
:
<ResourceDictionary
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:MSDNSample">
<Style x:Key="FooButton" TargetType="{x:Type Button}">
<Setter Property="Background" Value="Pink" />
</Style>
</ResourceDictionary>
回到MyView.xaml
(“控件的其余部分”):
<Button Style="{StaticResource FooButton}">
Hello World
</Button>
在解决方案资源管理器中右键单击您的项目选择添加之后单击资源字典...选择名称并添加到您的项目中。 打开App.xaml在应用程序标签中添加此代码
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="YourStyle.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
在 YourStyle.xaml 中:
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:APPNAME">
<Style x:Key="ButtonStyle" TargetType="{x:Type Button}">
<Setter Property="Background" Value="Pink" />
</Style>
</ResourceDictionary>
您正在寻找动态资源。 最好的方法是在资源中加载和调整字典。 应用程序或在控制页面上。 这是一个很好的示例。
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="Dictionary1.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
this.Resources.MergedDictionaries.Add(Smyresourcedictionary);
简单地,转到您想要包含外部文件中的资源的窗口(例如: MaindWindow.xaml
)并使用MergedDictionaries原则来引用该文件:
<Window x:Class="UseMergedResource.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow"
Height="600"
Width="600">
<Window.Resources>
<!-- DECLARING MERGED DICTIONARY -->
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source='Merged/BrushResources.xaml' />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
<!---------------------------------->
</Window.Resources>
<StackPanel>
<Rectangle Width='200'
Height='100'
Fill='{StaticResource PrimaryBrush}' /> <!-- USAGE HERE -->
</StackPanel>
</Window>
从上面
Merged/BrushResources.xaml
是资源文件的位置,它位于名为Merged
的文件夹下。
现在,如果您想知道外部文件中的声明语法应该是什么,请检查以下内容:
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<!-- Location for application brushes -->
<SolidColorBrush x:Key='BorderBrush'
Color='Orange' />
<SolidColorBrush x:Key='HighLightBrush'
Color='LightBlue' />
<SolidColorBrush x:Key='PrimaryBrush'
Color='Pink' />
<SolidColorBrush x:Key='AccentBrush'
Color='Yellow' />
</ResourceDictionary>
如果您想让资源通过所有应用程序(在所有窗口中可见)可用,请在
App.xaml
资源部分声明。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.