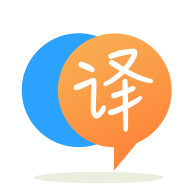
[英]How to initialize tm struct memebers in initializer list of a structure in C++ 98 standard
[英]Can we initialize structure memebers through smart pointer in initialization list?
#include <boost/scoped_ptr.hpp>
class classA
{
protected:
struct StructB
{
int iAge;
double dPrice;
};
boost::scoped_ptr<StructB> m_scpStructB;
public:
classA(int age, double price)
: m_scpStructB(new StructB)
{
m_scpStructB->iAge = age;
m_scpStructB->dPrice = price;
}
/* DO NOT COMPILE <= second block
classA(int age, double price)
: m_scpStructB(new StructB),
m_scpStructB->iAge(age),
m_scpStructB->dPrice(price)
{}
*/
};
Question1>我发现我无法使用第二段代码来初始化智能指针所指向的结构成员。 这是我们不能做的通用C ++规则吗?
如果第一个问题的答案是“您不能做到”,请放弃此问题。
Question2>据我所知,初始化列表上的赋值顺序基于成员变量定义的顺序。 假设您可以通过智能指针初始化成员变量。 您如何保证订单,以便始终先初始化智能点?
如果您不需要 StructB
为聚合/ POD类型,那么也只需为其提供一个构造函数即可:
#include <boost/scoped_ptr.hpp>
class classA
{
protected:
struct StructB
{
StructB(int age, double price) : iAge(age), dPrice(price) { }
int iAge;
double dPrice;
};
boost::scoped_ptr<StructB> m_scpStructB;
public:
classA(int age, double price) : m_scpStructB(new StructB(age, price)) { }
};
否则,您可以只使用工厂函数,这样它仍然是POD类型:
#include <boost/scoped_ptr.hpp>
class classA
{
protected:
struct StructB
{
int iAge;
double dPrice;
static StructB* make(int age, double price)
{
StructB* ret = new StructB;
ret->iAge = age;
ret->dPrice = price;
return ret;
}
};
boost::scoped_ptr<StructB> m_scpStructB;
public:
classA(int age, double price) : m_scpStructB(StructB::make(age, price)) { }
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.