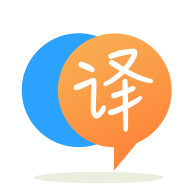
[英]How can I test if a class method is calling another method from one of the classes's private objects
[英]Passing objects from one private method to another
我现在已经编程了一段时间,我对使用公共方法有疑问。 我正在研究自动售货机程序,我有一个私有方法setUpMachine()
来初始化游戏并设置对象。 我有另一个私有方法startMachine()
,它启动游戏并提示用户输入。 然后它将输入传递给另一个私有方法checkInput()
,它检查输入是否有效......但这是我遇到的问题,而不是一个“问题”,但我觉得我没有做某事正确。 我需要访问第一个方法中的对象, setUpMachine()
用于我的第三个方法checkInput()
。 问题是我有很多物品(糖果,薯片,苏打水,饼干),并将它们全部传递到检查周边,这似乎是不对的。 换句话说,这样做:
checkInput(FoodType candy, FoodType chips, FoodType soda, FoodType cookie)
似乎不对。 这是否意味着,如果我使用私有方法,每次我想使用它时都必须传递对象? 我读到制作公共方法是不好的做法。
对此的解释将是很好的,而不是解释告诉我我的编码是低效的,但更多的解释描述何时以及如何使用私有方法,或者是否有另一种方法去做这件事。
如果您不想传递对象,可以将它们配置为实例变量:
public class VendingMachine {
private FoodType candy;
private FoodType chips;
private FoodType sodas;
private FoodType cookies;
private static String errorMessage = "A really bad error occurred.";
public VendingMachine(){
this.setupMachine();
}
private void setUpMachine(){
this.candy = new FoodType();
this.chips = new FoodType();
this.sodas = new FoodType();
this.cookies = new FoodType();
}
private boolean checkInput(){
if (this.candy==null || this.chips==null || this.sodas==null || this.cookies==null)
return false;
else
return true;
}
public void doSomething() throws Exception() {
if (!this.checkInput()) throw new Exception(VendingMachine.errorMessage);
// do things
}
}
然后可以将此类称为
VendingMachine vendingMachine = new VendingMachine();
try {
//vendingMachine.checkInput() is not available because it is private
vendingMachine.doSomething(); // public method is available
} catch (Exception e){
// validation failed and threw an Exception
}
通常,当您想要向外界展示某些东西时,应该使用公共方法。 通常,它是一种更高级别的方法,其中私有方法用于提供详细信息。 私有方法可以轻松更改,而不会影响其他类,而公共方法则不会。
考虑这个例子
public void run() {
setUpGame();
validateInput();
doSomethingInteresting();
endGame();
notifyUserGameIsComplete();
}
在此示例中, run
是public,但没有其他方法。 这是因为公共方法的调用者只知道他们想要运行游戏,而不关心它究竟是如何工作的细节。
有很多私人方法是可以肯定的。 方法应该是单一的,每个只做一件事。 这使您可以更轻松地读取,调试和维护代码。
如果多个方法需要共享数据,则可以将它们声明为类的成员变量。 这意味着他们是阶级的状态。 例如:
public class Person {
private String name;
public String getName() { return name; }
public boolean nameMatches(String pattern) { // do some regex testing against the name }
}
在此示例中,methds共享对name
访问权限,而name
是该人员的一部分。
如果你真的需要传递一堆参数,你可以创建一个对象来保存所有参数。 因此,您可以创建数据传输对象,而不是传递param1,param2,param3等
class MyStuff {
private final String param1;
private final String param2;
private final String param3;
}
现在,您可以通过MyStuff
传递,而不是列出单个参数。
希望这有助于增加一些清晰度。
私人方法对于ouside世界是不可见的。 您只能从同一个类的方法中调用私有方法。
私有方法可用于逻辑上将大方法分解为许多较小的部分(步骤)。
此外,如果您的某些代码似乎在您的类中的多个方法中重复,那么您可以使用私有方法。
如果对象也是类中的一个字段,则不需要将对象定义为私有方法的parementer,但如果没有,则必须这样做。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.