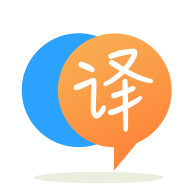
[英]Why isn't this program repainting a certain part of an applet even when repaint() is called
[英]repaint() isn't called in Java program
我有一个简单的程序(大部分将用于其他东西)只是在用户点击drawPanel JPanel并显示椭圆时绘制椭圆。 问题是repaint()方法没有调用paintComponent()。 为什么是这样?
这是代码:
// Imports Used:
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import javax.swing.*;
import java.awt.geom.*;
// Class DrawPolygon
public class DrawPolygon extends JPanel implements MouseListener
{
// Variables used in GUI
static JFrame frame;
static JPanel drawPanel;
static JPanel labelPanel;
static JPanel primaryPanel;
static JButton loadButton;
static JButton saveButton;
static JButton clearButton;
static JButton addButton;
static JButton moveButton;
static JButton exitButton;
// Variables used for GUI interaction
static final int SIZE = 6;
static int numVertices;
ArrayList<Point> vertices;
static Color outlineColor = Color.RED;
static int lineWidth = 10;
static Color fillColor = Color.BLACK;
// Constructor for new Polygon Drawing Application
public DrawPolygon()
{
// Create Frame
frame = new JFrame("Vector Painter");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Set Location of GUI on screen
frame.setLocation(225,100);
// Primary Panel to store Draw Panel and Lable/Button Panel
primaryPanel = new JPanel();
// Create Label Panel
createLabelPanel();
// Create Draw Panel
createDrawPanel();
// Add panels to Primary
primaryPanel.add(labelPanel);
primaryPanel.add(drawPanel);
// Add to frame
frame.getContentPane().add(primaryPanel);
frame.pack();
frame.setVisible(true);
}
// Add Buttons to left side
public void createLabelPanel()
{
// Label Panel to add Buttons
labelPanel = new JPanel();
labelPanel.setBackground(Color.BLACK);
labelPanel.setPreferredSize(new Dimension(200,600));
// Create JButtons
loadButton = new JButton("LOAD");
loadButton.setPreferredSize(new Dimension(180,75));
loadButton.setBackground(Color.BLACK);
loadButton.setForeground(Color.WHITE);
loadButton.addMouseListener(this);
saveButton = new JButton("SAVE");
saveButton.setPreferredSize(new Dimension(180,75));
saveButton.setBackground(Color.BLACK);
saveButton.setForeground(Color.WHITE);
saveButton.addMouseListener(this);
clearButton = new JButton("CLEAR");
clearButton.setPreferredSize(new Dimension(180,75));
clearButton.setBackground(Color.BLACK);
clearButton.setForeground(Color.WHITE);
clearButton.addMouseListener(this);
addButton = new JButton("ADD");
addButton.setPreferredSize(new Dimension(180,75));
addButton.setBackground(Color.BLACK);
addButton.setForeground(Color.WHITE);
addButton.addMouseListener(this);
moveButton = new JButton("MOVE");
moveButton.setPreferredSize(new Dimension(180,75));
moveButton.setBackground(Color.BLACK);
moveButton.setForeground(Color.WHITE);
moveButton.addMouseListener(this);
exitButton = new JButton("EXIT");
exitButton.setPreferredSize(new Dimension(180,75));
exitButton.setBackground(Color.BLACK);
exitButton.setForeground(Color.WHITE);
exitButton.addMouseListener(this);
// Add Buttons to Label Panel
labelPanel.add(loadButton);
labelPanel.add(saveButton);
labelPanel.add(clearButton);
labelPanel.add(addButton);
labelPanel.add(moveButton);
labelPanel.add(exitButton);
}
// Creates Draw Panel
public void createDrawPanel()
{
// Draw Panel to Draw Polygons
drawPanel = new JPanel();
drawPanel.setBackground(Color.BLACK);
drawPanel.setPreferredSize(new Dimension(600,600));
drawPanel.addMouseListener(this);
vertices = new ArrayList<>();
}
@Override
public void paintComponent(Graphics g)
{
super.paintComponent(g);
g.setColor(Color.ORANGE);
for (Point spot : vertices)
{
g.fillOval(spot.x-SIZE, spot.y-SIZE, SIZE*2, SIZE*2);
}
g.drawString("Count: " + vertices.size(), 5, 15);
System.out.println("repaint is working?");
}
// Execute when Load button is clicked
public void loadButton()
{
System.out.println("Load Button CLICKED!!");
}
// Execute when Save button is clicked
public void saveButton()
{
System.out.println("Save Button CLICKED!!");
}
// Execute when Clear button is clicked
public void clearButton()
{
System.out.println("Clear Button CLICKED!!");
}
// Execute when Add button is clicked
public void addButton()
{
System.out.println("Add Button CLICKED!!");
}
// Execute when Move button is clicked
public void moveButton()
{
System.out.println("Move Button CLICKED!!");
}
public void mouseClicked(MouseEvent e)
{
if (e.getSource() == loadButton)
{
loadButton();
}
else if (e.getSource() == saveButton)
{
saveButton();
}
else if (e.getSource() == clearButton)
{
clearButton();
}
else if (e.getSource() == addButton)
{
addButton();
}
else if (e.getSource() == moveButton)
{
moveButton();
}
else if (e.getSource() == exitButton)
{
System.exit(0);
}
else if (e.getSource() == drawPanel)
{
System.out.println("TEST");
vertices.add(e.getPoint());
repaint();
}
}
// These are here because program wouldn't compile without them
public void mousePressed(MouseEvent e){}
public void mouseReleased(MouseEvent e){}
public void mouseExited(MouseEvent e){}
public void mouseEntered(MouseEvent e){}
// Main Function
public static void main(String[] args)
{
// Create Frame
new DrawPolygon();
}
}
实际上正在调用重绘,但在当前的DrawPolygon对象上,但由于您似乎没有显示其中一个paintComponent(...)
将永远不会被调用。 为了澄清,您当前的类DrawPolygon扩展了JPanel,但它似乎没有被添加到任何属于GUI层次结构的容器中。 也许你想把你当前的对象,你的this
,添加到GUI的某个地方? 实际上,您可能应该考虑使用当前对象代替drawPanel JPanel对象。 我会考虑完全删除该变量。
与您的问题无关,您几乎不想在JButton上使用MouseListeners,而应该使用ActionListeners。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.