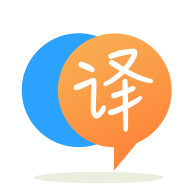
[英]Displaying the nodes of binary search tree which belongs depth path of the tree
[英]Displaying Path to a Node in a Binary Search Tree
我正在尝试显示从BST的根节点到目标节点的路径。 我具有的功能在前两层都可以正常工作,但是在此之后又变得混乱了。 例如,测试编号为6、9、4、11、10(按此顺序插入)。 如果我搜索6、9或4,则可以正常运行(例如:“ 6 9”)。 但是,如果我尝试11或10,它将同时显示它们,并且显示不正确。 我有点为难。 任何想法都很棒!
template <class T>
void BST<T>::displayPath(T searchKey, BST<T> *node)
{
if (searchKey == node->mData)
{
cout << node->mData << " ";
}
else if (searchKey < node->mData )
{
cout << node->mData << " ";
displayPath(searchKey, node->mLeft);
}
else// (searchKey > node->mData)
{
cout << node->mData << " ";
displayPath(searchKey, node->mRight);
}
}
这是插入功能。 数字按上述顺序插入。
template <class T>
void BST<T>::insert(BST<T> *&node, T data)
{
// If the tree is empty, make a new node and make it
// the root of the tree.
if (node == NULL)
{
node = new BST<T>(data, NULL, NULL);
return;
}
// If num is already in tree: return.
if (node->mData == data)
return;
// The tree is not empty: insert the new node into the
// left or right subtree.
if (data < node->mData)
insert(node->mLeft, data);
else
insert(node->mRight, data);
}
您的验证码证实了我的怀疑。 你的方法是罚款-这是您从插入内置树6, 9, 4, 11, 10
顺序如下:
第一(6):
6
第二(9)
6
\
9
第三(4)
6
/ \
4 9
第四(11):
6
/ \
/ \
4 9
\
\
11
第五(10):
6
/ \
/ \
4 9
\
\
11
/
/
10
因此,搜索10将为您提供路径(6,9,11,10)。
请注意,从根到BST中的元素的路径不能保证得到排序-如果这是您所期望的。 实际上,只有当节点位于树中最右边的叶子的路径上时,它才会被排序。
代码中的另一个问题:搜索7(或树中不存在的任何元素)会给您一些虚假的路径。
仅当输入值在树中时,该代码才有效。 当您搜索不存在的值时,您正在寻找不在树中的节点
template <class T>
void BST<T>::displayPath(T searchKey, BST<T> *node)
{
if (searchKey == node->mData)
{
cout << node->mData << " ";
}
else if (searchKey < node->mData )
{
cout << node->mData << " ";
if (node->mLeft == NULL) // When trying to access a node that isn't there
cout << "Not Found\n";
else
displayPath(searchKey, node->mLeft);
}
else // (searchKey > node->mData)
{
cout << node->mData << " ";
if (node->mRight == NULL)
cout << "Not Found\n";
else
displayPath(searchKey, node->mRight);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.