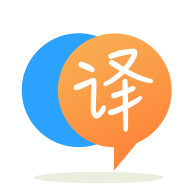
[英]How to change value of KeyValuePair in a List as a value in a Dictionary?
[英]How to modify a KeyValuePair value?
当我尝试修改项目的值时遇到问题,因为它只是一个只读字段。
KeyValuePair<Tkey, Tvalue>
我尝试了不同的选择,例如:
Dictionary<Tkey, Tvalue>
但我有同样的问题。 有没有办法将值字段设置为新值?
你不能修改它,你可以用一个新的替换它。
var newEntry = new KeyValuePair<Tkey, Tvalue>(oldEntry.Key, newValue);
或字典:
dictionary[oldEntry.Key] = newValue;
在这里,如果您想让 KeyValuePair 可变。
制作自定义类。
public class KeyVal<Key, Val>
{
public Key Id { get; set; }
public Val Text { get; set; }
public KeyVal() { }
public KeyVal(Key key, Val val)
{
this.Id = key;
this.Text = val;
}
}
所以我们可以在 KeyValuePair 的任何地方使用它。
KeyValuePair<TKey, TValue>
是不可变的。 您需要使用修改后的键或值创建一个新的。 你接下来实际做什么取决于你的场景,以及你到底想做什么......
KeyValuePair 是不可变的,
namespace System.Collections.Generic
{
[Serializable]
public struct KeyValuePair<TKey, TValue>
{
public KeyValuePair(TKey key, TValue value);
public TKey Key { get; }
public TValue Value { get; }
public override string ToString();
}
}
如果您要更新 KeyValuePair 中的任何现有值,那么您可以尝试删除现有值,然后添加修改后的值
例如:
var list = new List<KeyValuePair<string, int>>();
list.Add(new KeyValuePair<string, int>("Cat", 1));
list.Add(new KeyValuePair<string, int>("Dog", 2));
list.Add(new KeyValuePair<string, int>("Rabbit", 4));
int removalStatus = list.RemoveAll(x => x.Key == "Rabbit");
if (removalStatus == 1)
{
list.Add(new KeyValuePair<string, int>("Rabbit", 5));
}
KeyValuePair<TKey, TValue>
是一个结构体,C# 中的结构体是值类型,并且被提到是不可变的。 原因很明显, Dictionary<TKey,TValue>
应该是高性能的数据结构。 使用引用类型而不是值类型会使用过多的内存开销。 与字典中直接存储的值类型不同,另外将为字典中的每个条目分配 32 位或 64 位引用。 这些引用将指向入口实例的堆。 整体性能会迅速下降。
选择 struct 而不是Dictionary<TKey,TValue>
满足的类的 Microsoft 规则:
✔️ 如果类型的实例很小且通常寿命很短或通常嵌入其他对象中,请考虑定义结构而不是类。
❌ 避免定义结构,除非该类型具有以下所有特征:
您不能修改 KeyValuePair,但可以像这样修改字典值:
foreach (KeyValuePair<String, int> entry in dict.ToList())
{
dict[entry.Key] = entry.Value + 1;
}
或者像这样:
foreach (String entry in dict.Keys.ToList())
{
dict[entry] = dict[entry] + 1;
};
Dictionary<long, int> _rowItems = new Dictionary<long, int>();
_rowItems.Where(x => x.Value > 1).ToList().ForEach(x => { _rowItems[x.Key] = x.Value - 1; });
对于 Dictionary 我们可以根据某些条件以这种方式更新值。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.