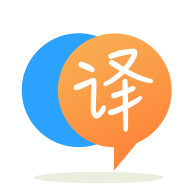
[英]How can I tell whether it is a call to a static method or an instance method?
[英]How to call instance method in static method
所以我想做的是讀取.txt
文件並使用eclipse在其上添加一些記錄。 我將我命名為“ fileName
”的資源設置為私有,當我嘗試在main方法中調用它時,出現了一些錯誤。 這是我的代碼:
public class FileController {
private String fileName;
public FileController() {
}
public FileController(String fileName) {
fileName = "student.txt";
}
public void readLine() {
try {
FileReader fr = new FileReader(fileName);
Scanner sc = new Scanner(fr);
// read in the file line by line
while (sc.hasNextLine()) {
System.out.println(sc.nextLine());
}
fr.close();
} catch (FileNotFoundException exception) {
System.out.println("The file " + fileName + " was not found.");
} catch (IOException exception) {
System.out.println(exception);
}
}
public void writeLine() {
try {
// create the PrintWriter
FileWriter fw = new FileWriter(fileName, true);
BufferedWriter bw = new BufferedWriter(fw);
PrintWriter outFile = new PrintWriter(bw);
// write value out to the file
outFile.println("Coke is nice");
outFile.println("Diet Coke is even better cos won't put on weight =)");
// close the file
outFile.close();
System.out.println("File created: " + fileName);
} catch (IOException exception) {
System.out.println(exception);
}
}
public static void main(String[] args) {
FileController fs = new FileController();
fs.readLine();
fs.writeLine();
}
}
有人可以給我一些線索嗎? 這些代碼不斷給我NullPointerException錯誤 。 我知道這是從FileController fs = new FileController()
那一行開始的,但是我不知道如何在靜態方法中調用實例方法。
FileController fs = new FileController();
應該
FileController fs = new FileController("fileName");
您還應該像這樣編輯構造函數。由於構造函數中的類變量fileName和參數名稱具有相同的名稱,因此必須使用“ this”關鍵字進行賦值。
public FileController(String fileName) {
this.fileName = fileName;
}
如果參數名稱和類變量名稱不同,則可以執行此操作。
public FileController(String name) {
fileName = name;
}
嘗試:
public FileController() {
fileName = "student.txt";
}
public FileController(String fileName) {
this.fileName = filename;
}
我認為您的構造函數應如下所示:
public FileController(String fileName) {
this.fileName = fileName;
}
像這樣的無參數構造函數:
public FileController() {
this("student.txt");
}
您沒有在構造函數中傳遞文件名。 您應該在其中傳遞有效的文件名,以使邏輯正常工作
您需要使用參數調用構造函數,或者您的默認構造函數應在主目錄中提供默認文件名,例如:
FileController fs = new FileController("somefile.txt")
並且您的構造函數需要更改為:
public FileController(String filename) {
this.fileName = filename;
}
您可以更改默認構造函數:
public FileController() {
this("someDefaultFile.txt");
}
請記住,僅當要查找默認文件時,后面的選項才有意義,否則,您應該顯式傳遞文件名。
可以使用要調用其方法的類的對象引用在靜態方法中訪問實例方法。
因此,如您正確指出的那樣,使用new關鍵字將解決您的問題。
問題是通過調用new FileController(); 您沒有初始化fileName字段。 您可能希望構造函數如下所示:
public FileController() {
this.fileName = "student.txt";
}
public FileController(String fileName) {
this.fileName = fileName;
}
然后將調用new FileController(); 合法。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.