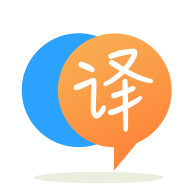
[英]How to remove duplicates ArrayList from an ArrayList of ArrayList
[英]How to remove Duplicates(Old Objects) from arraylist
我有一個Check Entity
,其中有三個屬性,包括Id,並且正在使用Id作為哈希碼,以便使用和檢查重復項。
現在,使用以下代碼刪除重復項
Set<Check> unique = new LinkedHashSet<Check>(l);
List<Check> finalLst= new java.util.ArrayList<Check>();
finalLst.addAll(unique);
在輸出中
這三個是作為result(c1,c2和c3)來的,但是我想要(c4,c5和c6)。
Check c1 = new Check(1,"one");
Check c2 = new Check(2,"two");
Check c3 = new Check(3,"three");
Check c4 = new Check(1,"one");
Check c5 = new Check(2,"two");
Check c6 = new Check(3,"three");
輸出正在獲取:
id :1 ::2013-04-30 10:42:34.311
id :2 ::2013-04-30 10:42:34.344
id :3 ::2013-04-30 10:42:34.344
id :1 ::2013-04-30 10:42:34.344
id :2 ::2013-04-30 10:42:34.345
id :3 ::2013-04-30 10:42:34.345
1 :: 2013-04-30 10:42:34.311
2 :: 2013-04-30 10:42:34.344
3 :: 2013-04-30 10:42:34.344
期望的輸出:
id :1 ::2013-04-30 10:42:34.311
id :2 ::2013-04-30 10:42:34.344
id :3 ::2013-04-30 10:42:34.344
id :1 ::2013-04-30 10:42:34.344
id :2 ::2013-04-30 10:42:34.345
id :3 ::2013-04-30 10:42:34.345
1 :: 2013-04-30 10:42:34.344
2 :: 2013-04-30 10:42:34.345
3 :: 2013-04-30 10:42:34.345
我的整個代碼在這里:
package test.collection;
import java.text.SimpleDateFormat;
import java.util.*;
public class RemoveDuplicateInArrayList
{
public static void main(String args[])
{
Check c1 = new Check(1,"one");
Check c2 = new Check(2,"two");
Check c3 = new Check(3,"three");
Check c4 = new Check(1,"one");
Check c5 = new Check(2,"two");
Check c6 = new Check(3,"three");
List<Check> l = new java.util.ArrayList<Check>();
l.add(c1);
l.add(c2);
l.add(c3);
l.add(c4);
l.add(c5);
l.add(c6);
List<Check> finalLst= removeDuplicates(l);
Iterator<Check> iter = finalLst.iterator();
while(iter.hasNext())
{
Check temp = iter.next();
System.out.println(temp.getId()+" :: "+new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S").format(temp.getCreationTme()));
}
}
public static List<Check> removeDuplicates(List<Check> l)
{
Set<Check> unique = new LinkedHashSet<Check>(l);
List<Check> finalLst= new java.util.ArrayList<Check>();
finalLst.addAll(unique);
return finalLst;
}
}
class Check
{
public Check(int id,String name)
{
this.id = id;
this.name = name;
this.creationTme = new Date();
System.out.println("id :"+this.id+" ::"+new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S").format(this.getCreationTme()));
}
private int id;
private String name;
private Date creationTme;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getCreationTme() {
return creationTme;
}
public void setCreationTme(Date creationTme) {
this.creationTme = creationTme;
}
@Override
public int hashCode()
{
return this.id;
}
@Override
public boolean equals(Object obj)
{
if(obj instanceof Check && ((Check)obj).id == this.id)
return true;
else return false;
}
}
您可以實現Comparable
接口並在您的check
類中重寫compareTo()
方法,如下所示:
class Check implements Comparable<Check>{
和compareTo()
方法
@Override
public int compareTo(Check o) {
if(creationTme.after(o.getCreationTme())){
return -1;
}else if(creationTme.before(o.getCreationTme())){
return 1;
}
return 0;
}
在刪除重復項之前,您可以像這樣對列表進行Sort
:-
Collections.sort(l);
List<Check> finalLst = removeDuplicates(l);
哈希集按以下方式工作:如果嘗試添加對象,則將計算其哈希碼。 如果哈希碼已經存在於映射中,則什么也不會發生(為什么呢?哈希碼-以及等效的Object-已經存在),因此您的輸出是完全合理的:)
如果您想擁有一個覆蓋哈希集,建議您編寫自己的。
參加帶有私有哈希集的課程。 add methode首先檢查哈希碼是否已經存在,如果是:從集合中刪除該值並添加新的。 如果否:只需添加即可。
改用地圖
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
public class RemoveDuplicateInArrayList
{
public static void main(String args[])
{
Check c1 = new Check(1,"one");
Check c2 = new Check(2,"two");
Check c3 = new Check(3,"three");
Check c4 = new Check(1,"one");
Check c5 = new Check(2,"two");
Check c6 = new Check(3,"three");
Map<Integer, Check> map = new HashMap<Integer, Check>();
map.put( c1.getId() , c1 );
map.put( c2.getId() , c2 );
map.put( c3.getId() , c3 );
map.put( c4.getId() , c4 );
map.put( c5.getId() , c5 );
map.put( c6.getId() , c6 );
System.out.println( map );
}
}
class Check
{
public Check(int id,String name)
{
this.id = id;
this.name = name;
this.creationTme = new Date();
System.out.println("id :"+this.id+" ::"+this.getCreationTme().getTime());
}
private Integer id;
private String name;
private Date creationTme;
public int getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getCreationTme() {
return creationTme;
}
public void setCreationTme(Date creationTme) {
this.creationTme = creationTme;
}
@Override
public int hashCode()
{
return this.id;
}
@Override
public boolean equals(Object obj)
{
if(obj instanceof Check && ((Check)obj).id == this.id)
return true;
else return false;
}
public String toString()
{
final String TAB = " ";
String retValue = "";
retValue = "Check ( "
+ "id = " + this.id + TAB
+ "name = " + this.name + TAB
+ "creationTme = " + this.creationTme.getTime() + TAB
+ " )";
return retValue;
}
}
PS:我不是低俗主義者:)
我對您的方法做了一些修改,以便可以得到預期的結果。 基本上,您需要最新的。 所以這里是:
public static List<Check> removeDuplicates(List<Check> l)
{
Collections.reverse(l);
Set<Check> unique = new LinkedHashSet<Check>(l);
List<Check> finalLst= new java.util.ArrayList<Check>();
finalLst.addAll(unique);
Collections.reverse(finalLst);
return finalLst;
}
試試這個,它將起作用
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.