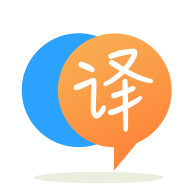
[英]GWT (Client) = How to convert Object to JSON and send to Server?
[英]how to convert Date object to string/long and send it via client/server
嗨,我在將Date對象從客戶端發送到服務器時遇到了一些麻煩,以便可以對其進行比較。 所以基本上我想做的是:1)發送Date對象2)比較它們的時間3)設置兩台計算機的時間,同時計算兩者的平均時間
到目前為止,我已經做到了:
服務器:
public class Server implements Runnable{
private int port;
private String name;
private Date mydate = new Date();
private Date date;
public Server(int port, String name){
this.port=port;
this.name=name;
}
public synchronized void run(){
while(true){
try{
method();
}
catch(Exception e){
return;
}
}
}
public synchronized void method() throws Exception{
long dates;
long average=0;
String[] values = new String[10];
DateFormat df = new SimpleDateFormat("EEE MMM dd kk:mm:ss zzz yyyy");
ServerSocket server = new ServerSocket(port);
Socket s=server.accept();
InputStream in= s.getInputStream();
OutputStream out = s.getOutputStream();
PrintWriter w = new PrintWriter(out);
Scanner r = new Scanner(in);
for(int i=0; i<10; i++){
String msg = r.next();
values[i] = msg;
}
for(int j=0; j<values.length; j++){
dates = Long.parseLong(values[j]);
date = new Date(dates);
average = average + date.getTime();
}
average = (average - mydate.getTime())/2;
mydate.setTime(average);
System.out.println(name+": "+mydate.toString());
}
}
客戶:
public class Client implements Runnable{
private int port;
private int id;
Date time = new Date();
Date time2 = new Date();
public Client(int port,int id){
this.port=port;
this.id = id;
}
public synchronized void run(){
try{
Thread.sleep(5000);
method();
}
catch(Exception e){
return;
}
}
public synchronized void method() throws Exception{
DateFormat df = new SimpleDateFormat("EEE MMM dd kk:mm:ss zzz yyyy");
Random ran = new Random(500);
int d = ran.nextInt(500)+500;
time.setTime(time.getTime()+d);
String datestr;
Socket s = new Socket("localhost", port);
InputStream in= s.getInputStream();
OutputStream out = s.getOutputStream();
PrintWriter w = new PrintWriter(out);
Scanner r = new Scanner(in);
for(int i=0; i<10; i++){
time.setTime(time.getTime()+d);
datestr = df.format(time);
w.println(datestr);
w.flush();
}
}
}
但是我得到的結果僅僅是空白。 該程序現在可以正確編譯,並帶有錯誤,並且我知道問題出在哪里,但我只是想不出如何正確解析值,以便可以正確讀取它們。 請幫我:S
在您的客戶端中,將日期作為由SimpleDateFormat格式化的字符串發送,結果類似:“ Sat Mai 04 22:27:24 PST 2013”
在服務器端,您可以使用此字符串嘗試將其解析為long,以便將來可以在Date(long date)構造函數中使用它。
新的Date(長日期)期望日期為unixtime。 調用date.getTime()會得到Unixtime。 Unixtime是毫秒為單位的毫秒,即01.01.1970 00:00:00:0000。
我希望服務器拋出som類的解析異常。
如果要使用SimpleDateFormat格式化發送端,而要在接收端使用SimpleDateFormat解析,則必須離開。 或發送unixtime。
我建議發送unixtime。 您可以通過以下方式從Date對象轉換:
Long unixtime = date.getTime()
然后回來:
Date date = new Date(unixtime)
或在服務器端根本不需要日期對象,只需使用它來調用getTime()即可,然后又返回unixtime。
服務器:
public class Server implements Runnable{
private int port;
private String name;
private Date mydate = new Date();
private Date date;
public Server(int port, String name){
this.port=port;
this.name=name;
}
public synchronized void run(){
while(true){
try{
method();
}
catch(Exception e){
return;
}
}
}
public synchronized void method() throws Exception{
long unixtime;
long average=0;
String[] values = new String[10];
ServerSocket server = new ServerSocket(port);
Socket s=server.accept();
InputStream in= s.getInputStream();
OutputStream out = s.getOutputStream();
PrintWriter w = new PrintWriter(out);
Scanner r = new Scanner(in);
for(int i=0; i<10; i++){
String msg = r.next();
values[i] = msg;
}
for(int j=0; j<values.length; j++){
unixtime= Long.parseLong(values[j]);
average = average + unixtime;
}
average = (average - mydate.getTime())/2;
mydate.setTime(average);
System.out.println(name+": "+mydate.toString());
}
}
客戶:
public class Client implements Runnable{
private int port;
private int id;
Date time = new Date();
Date time2 = new Date();
public Client(int port,int id){
this.port=port;
this.id = id;
}
public synchronized void run(){
try{
Thread.sleep(5000);
method();
}
catch(Exception e){
return;
}
}
public synchronized void method() throws Exception{
Random ran = new Random(500);
int d = ran.nextInt(500)+500;
time.setTime(time.getTime()+d);
Socket s = new Socket("localhost", port);
InputStream in= s.getInputStream();
OutputStream out = s.getOutputStream();
PrintWriter w = new PrintWriter(out);
Scanner r = new Scanner(in);
for(int i=0; i<10; i++){
time.setTime(time.getTime()+d);
w.println(time.getTime());
w.flush();
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.