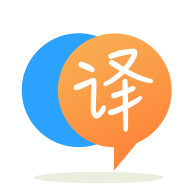
[英]Splitting a tuple in to a list without splitting it in to individual characters
[英]Converting string to tuple without splitting characters
我正在努力將字符串轉換為元組,而不在此過程中拆分字符串的字符。 有人可以建議一種簡單的方法來做到這一點。 需要一個襯里。
失敗
a = 'Quattro TT'
print tuple(a)
作品
a = ['Quattro TT']
print tuple(a)
由於我的輸入是一個字符串,我通過將字符串轉換為列表來嘗試下面的代碼,該列表再次將字符串拆分為字符..
失敗
a = 'Quattro TT'
print tuple(list(a))
預期輸出:
('Quattro TT')
生成的輸出:
('Q', 'u', 'a', 't', 't', 'r', 'o', ' ', 'T', 'T')
你可以只做(a,)
。 無需使用函數。 (注意逗號是必須的。)
本質上, tuple(a)
意味着創建一個包含a
內容的元組,而不是一個只a
本身的元組。 字符串的“內容”(迭代它時得到的)是它的字符,這就是它被拆分成字符的原因。
一個特殊的問題是包含 0 或 1 個項目的元組的構造:語法有一些額外的怪癖來適應這些。 空元組由一對空括號構成; 包含一項的元組是通過在值后面加上逗號來構造的(將單個值括在括號中是不夠的)。 丑陋,但有效。 例如:
>>> empty = () >>> singleton = 'hello', # <-- note trailing comma >>> len(empty) 0 >>> len(singleton) 1 >>> singleton ('hello',)
如果您只在字符串對象周圍放置一對括號,它們只會將該表達式轉換為帶括號的表達式(添加了強調):
帶括號的表達式列表產生表達式列表產生的任何結果:如果列表包含至少一個逗號,則產生一個元組; 否則,它產生構成表達式列表的單個表達式。
一對空括號產生一個空元組對象。 由於元組是不可變的,文字規則適用(即,空元組的兩次出現可能會或可能不會產生相同的對象)。
請注意,元組不是由括號形成的,而是使用逗號運算符形成的。 例外是空元組,因為它需要括號——在表達式中允許沒有括號的“無”會導致歧義並允許常見的錯別字未被發現。
即(假設 Python 2.7),
a = 'Quattro TT'
print tuple(a) # <-- you create a tuple from a sequence
# (which is a string)
print tuple([a]) # <-- you create a tuple from a sequence
# (which is a list containing a string)
print tuple(list(a)) # <-- you create a tuple from a sequence
# (which you create from a string)
print (a,) # <-- you create a tuple containing the string
print (a) # <-- it's just the string wrapped in parentheses
輸出如預期:
('Q', 'u', 'a', 't', 't', 'r', 'o', ' ', 'T', 'T')
('Quattro TT',)
('Q', 'u', 'a', 't', 't', 'r', 'o', ' ', 'T', 'T')
('Quattro TT',)
Quattro TT
在打印語句上添加一些注釋。 當您嘗試在 Python 2.7 中創建單元素元組作為print語句的一部分(如在print (a,)
)時,您需要使用括號形式,因為print a,
的尾隨逗號將被視為的一部分打印語句,從而導致從輸出中抑制換行符,而不是正在創建的元組:
'\\n' 字符寫在末尾,除非打印語句以逗號結尾。
在 Python 3.x 中,示例中的大多數上述用法實際上會引發SyntaxError
,因為在 Python 3 中print
變成了一個函數(您需要添加一對額外的括號)。 但特別是這可能會引起混淆:
print (a,) # <-- this prints a tuple containing `a` in Python 2.x
# but only `a` in Python 3.x
我使用此函數將字符串轉換為元組
import ast
def parse_tuple(string):
try:
s = ast.literal_eval(str(string))
if type(s) == tuple:
return s
return
except:
return
用法
parse_tuple('("A","B","C",)') # Result: ('A', 'B', 'C')
在你的情況下,你做
value = parse_tuple("('%s',)" % a)
以防萬一有人來這里想知道如何創建一個元組,將字符串“Quattro”和“TT”的每個部分分配給列表的一個元素,就像這個print tuple(a.split())
您可以使用以下解決方案:
s="jack"
tup=tuple(s.split(" "))
output=('jack')
您可以使用eval()
>>> a = ['Quattro TT']
>>> eval(str(a))
['Quattro TT']
子類化元組其中一些子類實例可能需要是單字符串實例會拋出一些有趣的東西。
class Sequence( tuple ):
def __init__( self, *args ):
# initialisation...
self.instances = []
def __new__( cls, *args ):
for arg in args:
assert isinstance( arg, unicode ), '# arg %s not unicode' % ( arg, )
if len( args ) == 1:
seq = super( Sequence, cls ).__new__( cls, ( args[ 0 ], ) )
else:
seq = super( Sequence, cls ).__new__( cls, args )
print( '# END new Sequence len %d' % ( len( seq ), ))
return seq
注意,正如我從該線程中了解到的,您必須在args[ 0 ]
之后放置逗號。
打印行顯示單個字符串沒有被拆分。
注意子類的構造函數中的逗號現在變為可選:
Sequence( u'silly' )
要么
Sequence( u'silly', )
看:
'Quattro TT'
是一個字符串。
由於字符串是一個字符列表,這與
['Q', 'u', 'a', 't', 't', 'r', 'o', ' ', 'T', 'T']
現在...
['Quattro TT']
是一個在第一個位置帶有字符串的列表。
還...
a = 'Quattro TT'
list(a)
是轉換為列表的字符串。
同樣,由於 string 是一個字符列表,因此沒有太大變化。
另一個信息...
tuple(something)
這將某些內容轉換為元組。
了解了所有這些,我想您可以得出結論,沒有任何事情會失敗。
這僅涵蓋一個簡單的情況:
a = ‘Quattro TT’
print tuple(a)
如果您只使用像 ',' 這樣的分隔符,那么它可以工作。
我使用了來自configparser
的字符串, configparser
所示:
list_users = (‘test1’, ‘test2’, ‘test3’)
and the i get from file
tmp = config_ob.get(section_name, option_name)
>>>”(‘test1’, ‘test2’, ‘test3’)”
在這種情況下,上述解決方案不起作用。 但是,這確實有效:
def fot_tuple(self, some_str):
# (‘test1’, ‘test2’, ‘test3’)
some_str = some_str.replace(‘(‘, ”)
# ‘test1’, ‘test2’, ‘test3’)
some_str = some_str.replace(‘)’, ”)
# ‘test1’, ‘test2’, ‘test3’
some_str = some_str.replace(“‘, ‘”, ‘,’)
# ‘test1,test2,test3’
some_str = some_str.replace(“‘”, ‘,’)
# test1,test2,test3
# and now i could convert to tuple
return tuple(item for item in some_str.split(‘,’) if item.strip())
val_1=input("").strip(")(").split(",") #making a list from the string
if val_1[0].isdigit()==True: #checks if your contents are all integers
val_2 = []
for i in val_1:
val_2.append(int(i))
val_2 = tuple(val_2)
print(val_2)
else: # if the contents in your tuple are of string, tis case is applied
val_2=[]
for i in range(0,len(val_1)):
if i==0:
val_2.append(val_1[i][1:-1])
else:
val_2.append(val_1[i][2:-1])
val_2=tuple(val_2)
print(val_2)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.