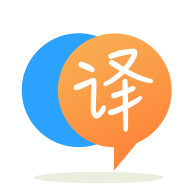
[英]How can I easily check in Python that all items in one list are included in the other list?
[英]How do I check to see if all of the items in one list are in a second list in python?
我是python的新手,並且在Python和一些Googling上的一小節課中將它們組合在一起。 我正在嘗試比較兩個字符串列表,以查看列表A的所有項目是否都在列表B中。如果列表B中沒有任何項目,我希望它打印一條通知消息。
List_A = ["test_1", "test_2", "test_3", "test_4", "test_5"]
List_B = ["test_1", "test_2", "test_3", "test_4"]
碼:
for item in List_A:
match = any(('[%s]'%item) in b for b in List_B)
print "%10s %s" % (item, "Exists" if match else "No Match in List B")
輸出:
test_1列表B中沒有匹配項
test_2列表B中沒有匹配項
test_3列表B中沒有匹配項
test_4列表B中沒有匹配項
test_5列表B中沒有匹配項
前四個應該匹配但不匹配,第五個是正確的。 我不知道為什么它不起作用。 有人可以告訴我我在做什么錯嗎? 任何幫助將不勝感激。
>>> '[%s]'%"test_1"
'[test_1]'
您正在檢查“ [test_1]”是否為list_B
中某個字符串的子字符串,依此類推。
這應該工作:
for item in List_A:
match = any(item in b for b in List_B)
print "%10s %s" % (item, "Exists" if match else "No Match in List B")
但既然你是不是真的找子,你應該測試in
,僅此而已:
for item in List_A:
match = item in List_B
print "%10s %s" % (item, "Exists" if match else "No Match in List B")
但是您可以簡單地使用集合差異:
print set(List_A) - set(List_B)
您可以將List_B
轉換為集合,因為集合提供了O(1)查找:
不過請注意,集合僅允許您存儲可哈希(不可變)的項目。 如果List_B
包含可變項,則首先將它們轉換為不可變項;如果不可能,則對List_B
排序並使用bisect
模塊對排序后的List_B
進行二進制搜索。
List_A = ["test_1", "test_2", "test_3", "test_4", "test_5"]
List_B = ["test_1", "test_2", "test_3", "test_4"]
s = set(List_B)
for item in List_A:
match = item in s # check if item is in set s
print "%10s %s" % (item, "Exists" if match else "No Match in List B")
輸出:
test_1 Exists
test_2 Exists
test_3 Exists
test_4 Exists
test_5 No Match in List B
只需使用一個簡單的for循環:
List_A = ["test_1", "test_2", "test_3", "test_4", "test_5"]
List_B = ["test_1", "test_2", "test_3", "test_4"]
for i in List_A:
print "{:>10} {}".format(i, "Exists" if i in List_B else "No Match in List B")
List_A = ["test_1", "test_2", "test_3", "test_4", "test_5"]
List_B = ["test_1", "test_2", "test_3", "test_4"]
for item in List_A:
match = any((str(item)) in b for b in List_B)
print "%10s %s" % (item, "Exists" if match else "No Match in List B")
將輸出
test_1 Exists
test_2 Exists
test_3 Exists
test_4 Exists
test_5 No Match in List B
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.