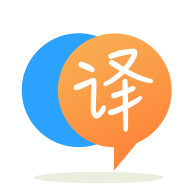
[英]Getting java.lang.UnsupportedOperationException: JsonNull reports
[英]Getting “java.lang.UnsupportedOperationException:”
我創建了一個小型JPA項目來保存學生記錄。 我使用Oracle數據庫。 我使用OpenJPA作為JPa提供程序。
我已正確創建了Table學生和相關序列。
學生實體課程
@Entity
@Table(name = "Student")
public class Student implements Serializable {
private int id;
private String name;
private static final long serialVersionUID = 1L;
public Student() {
super();
}
@Id
@Column(name = "ID")
@SequenceGenerator(name = "TRAIN_SEQ", sequenceName = "STUDENT_SEQ")
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "TRAIN_SEQ")
public int getId() {
return this.id;
}
public void setId(int id) {
this.id = id;
}
@Column(name = "NAME")
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
persistence.xml中
<?xml version="1.0" encoding="UTF-8"?>
<persistence version="2.0"
xmlns="http://java.sun.com/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd">
<persistence-unit name="JPAOracleDemo">
<provider>org.apache.openjpa.persistence.PersistenceProviderImpl</provider>
<class>com.jpa.demo.model.Student</class>
<properties>
<property name="openjpa.ConnectionURL" value="jdbc:oracle:thin:@TEST:50111:TESTPEGAD1" />
<property name="openjpa.ConnectionDriverName" value="oracle.jdbc.driver.OracleDriver" />
<property name="openjpa.ConnectionUserName" value="admin" />
<property name="openjpa.ConnectionPassword" value="admin" />
<property name="openjpa.RuntimeUnenhancedClasses" value="supported" />
<property name="openjpa.jdbc.Schema" value="MYSCHEMA" />
</properties>
</persistence-unit>
</persistence>
客戶類
OpenJPAEntityManager em = JPAUtil.getEntityManager();
OpenJPAEntityTransaction tx = em.getTransaction();
tx.begin();
// Create the instance of Employee Entity class
Student student = new Student();
student.setName("A.Ramesh");
// JPA API to store the Student instance on the database.
em.persist(student);
tx.commit();
em.close();
System.out.println("Done...");
Util類
private static OpenJPAEntityManagerFactory emf = OpenJPAPersistence
.createEntityManagerFactory("JPAOracleDemo", "META-INF/persistence.xml");
private static OpenJPAEntityManager entManager;
/**
* No need to create any instance for this Util.
*/
private JPAUtil() {
}
/**
* Get {@link EntityManager}.
*
* @return the {@link EntityManager}
*/
public static OpenJPAEntityManager getEntityManager() {
if (entManager == null || !entManager.isOpen()) {
entManager = emf.createEntityManager();
}
return entManager;
}
數據在學生表中成功保留,但我有以下錯誤
Exception in thread "Attachment 60230" java.lang.UnsupportedOperationException: cannot get the capability, performing dispose of the retransforming environment
at com.ibm.tools.attach.javaSE.Attachment.loadAgentLibraryImpl(Native Method)
at com.ibm.tools.attach.javaSE.Attachment.loadAgentLibrary(Attachment.java:253)
at com.ibm.tools.attach.javaSE.Attachment.parseLoadAgent(Attachment.java:235)
at com.ibm.tools.attach.javaSE.Attachment.doCommand(Attachment.java:154)
at com.ibm.tools.attach.javaSE.Attachment.run(Attachment.java:116)
Exception in thread "main" java.lang.UnsupportedOperationException: cannot get the capability, performing dispose of the retransforming environment
at sun.instrument.InstrumentationImpl.isRetransformClassesSupported0(Native Method)
at sun.instrument.InstrumentationImpl.isRetransformClassesSupported(InstrumentationImpl.java:124)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:48)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:37)
at java.lang.reflect.Method.invoke(Method.java:600)
at org.apache.openjpa.enhance.ClassRedefiner.canRedefineClasses(ClassRedefiner.java:123)
at org.apache.openjpa.enhance.ManagedClassSubclasser.prepareUnenhancedClasses(ManagedClassSubclasser.java:122)
at org.apache.openjpa.kernel.AbstractBrokerFactory.loadPersistentTypes(AbstractBrokerFactory.java:304)
at org.apache.openjpa.kernel.AbstractBrokerFactory.initializeBroker(AbstractBrokerFactory.java:228)
at org.apache.openjpa.kernel.AbstractBrokerFactory.newBroker(AbstractBrokerFactory.java:202)
at org.apache.openjpa.kernel.DelegatingBrokerFactory.newBroker(DelegatingBrokerFactory.java:156)
at org.apache.openjpa.persistence.EntityManagerFactoryImpl.createEntityManager(EntityManagerFactoryImpl.java:213)
at com.ibm.ws.persistence.EntityManagerFactoryImpl.createEntityManager(EntityManagerFactoryImpl.java:45)
at com.ibm.ws.persistence.EntityManagerFactoryImpl.createEntityManager(EntityManagerFactoryImpl.java:30)
at com.jpa.demo.util.JPAUtil.getEntityManager(JPAUtil.java:32)
at com.jpa.demo.client.JPAClient.main(JPAClient.java:13)
1045 JPAOracleDemo INFO [main] openjpa.Enhance - Creating subclass for "[class com.jpa.demo.model.Student]". This means that your application will be less efficient and will consume more memory than it would if you ran the OpenJPA enhancer. Additionally, lazy loading will not be available for one-to-one and many-to-one persistent attributes in types using field access; they will be loaded eagerly instead.
Done...
Java版本
JDK 1.6
有人請告訴我這里的問題是什么?
更新:
我使用IBM Rational Software Architect for Websphere Software進行此開發。 這個問題與這個IDE有關。 當我默認創建JPA項目時,它會添加IBM jre。 我剛剛刪除了IBM jre並嘗試了SUN jre,然后它就成功了。 請讓我知道為什么這個功能不支持IBM jre?
<property name="openjpa.RuntimeUnenhancedClasses" value="supported" />
首先,擺脫那個屬性。
這是我的增強模板,這適用於OPENJPA:`
<path id="enhance.cp">
<pathelement location="${basedir}${file.separator}${build.dir}" />
<fileset dir="${basedir}${file.separator}ext_libs/">
<include name="**/*.jar" />
</fileset>
</path>
<property name="cp" refid="enhance.cp" />
<target name="openjpa.libs.check" unless="openjpa.libs">
<fail message="Please set -Dopenjpa.libs in your builder configuration!" />
</target>
<target name="build.dir.check" unless="build.dir">
<fail message="Please set -Dbuild.dir in your builder configuration!" />
</target>
<target name="enhance" depends="openjpa.libs.check, build.dir.check">
<echo message="${cp}" />
<taskdef name="openjpac" classname="org.apache.openjpa.ant.PCEnhancerTask">
<classpath refid="enhance.cp" />
</taskdef>
<openjpac>
<classpath refid="enhance.cp" />
<configpropertiesFile="${basedir}${file.separator}src${file.separator}main${file.separator} resources${file.separator}META-INF${file.separator}persistence.xml" />
</openjpac>
</target>
`
JPA規范要求對Entity對象進行某種類型的監視,但規范沒有定義如何實現此監視。 一些JPA提供程序在運行時自動生成新的子類或代理對象,這些子類或代理對象位於用戶的Entity對象之前,而其他提供程序則使用字節碼編織技術來增強實際的Entity類對象。 OpenJPA支持這兩種機制,但強烈建議僅使用字節碼編織增強。 不建議使用子類化支持(由OpenJPA提供)(默認情況下在OpenJPA 2.0及更高版本中禁用)。(來源: http : //openjpa.apache.org/entity-enhancement.html )
這個問題的原因是我使用了實體增強的子類支持,但在OpenJPA2.0及更高版本中默認禁用。
我找到了解決這個問題的方法。 我們必須通過在啟動OpenJPA運行的JVM時提供javaagent來在運行時增強實體類。
我把以下內容作為JVM參數
-javaagent:C:/OpenJPA/apache-openjpa-2.0.0/openjpa-2.0.0.jar
我從persistence.xml中刪除了bellow行
<property name="openjpa.RuntimeUnenhancedClasses" value="supported" />
工作persistence.xml
<persistence version="2.0"
xmlns="http://java.sun.com/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd">
<persistence-unit name="DataSourceDemo">
<jta-data-source>oracleDS</jta-data-source>
<class>com.auditlog.model.BatchPrint</class>
<properties>
<property name="openjpa.ConnectionUserName" value="admin" />
<property name="openjpa.ConnectionPassword" value="test" />
<property name="openjpa.jdbc.Schema" value="defaultScheme" />
</properties>
</persistence-unit>
</persistence>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.