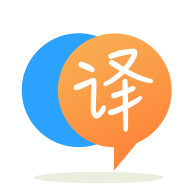
[英]Running Selenium Webdriver with a proxy in Python not changing IP
[英]Running Selenium Webdriver with a proxy in Python
我正在嘗試在 Python 中運行 Selenium Webdriver 腳本來執行一些基本任務。 當通過 Selenium IDE 界面運行它時,我可以讓機器人完美地到達 function(即:當簡單地讓 GUI 重復我的操作時)。 但是,當我將代碼導出為 Python 腳本並嘗試從命令行執行它時,Firefox 瀏覽器將打開但無法訪問起始的 URL(錯誤返回到命令行並且程序停止)。 無論我嘗試訪問哪個網站等,這都會發生在我身上。
為了演示目的,我在這里包含了一個非常基本的代碼。 我認為我沒有正確包含代碼的代理部分,因為返回的錯誤似乎是由代理生成的。
任何幫助將不勝感激。
下面的代碼只是為了打開 www.google.ie 並搜索單詞“selenium”。 對我來說,它會打開一個空白的 firefox 瀏覽器並停止。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import Select
from selenium.common.exceptions import NoSuchElementException
import unittest, time, re
from selenium.webdriver.common.proxy import *
class Testrobot2(unittest.TestCase):
def setUp(self):
myProxy = "http://149.215.113.110:70"
proxy = Proxy({
'proxyType': ProxyType.MANUAL,
'httpProxy': myProxy,
'ftpProxy': myProxy,
'sslProxy': myProxy,
'noProxy':''})
self.driver = webdriver.Firefox(proxy=proxy)
self.driver.implicitly_wait(30)
self.base_url = "https://www.google.ie/"
self.verificationErrors = []
self.accept_next_alert = True
def test_robot2(self):
driver = self.driver
driver.get(self.base_url + "/#gs_rn=17&gs_ri=psy-ab&suggest=p&cp=6&gs_id=ix&xhr=t&q=selenium&es_nrs=true&pf=p&output=search&sclient=psy-ab&oq=seleni&gs_l=&pbx=1&bav=on.2,or.r_qf.&bvm=bv.47883778,d.ZGU&fp=7c0d9024de9ac6ab&biw=592&bih=665")
driver.find_element_by_id("gbqfq").clear()
driver.find_element_by_id("gbqfq").send_keys("selenium")
def is_element_present(self, how, what):
try: self.driver.find_element(by=how, value=what)
except NoSuchElementException, e: return False
return True
def is_alert_present(self):
try: self.driver.switch_to_alert()
except NoAlertPresentException, e: return False
return True
def close_alert_and_get_its_text(self):
try:
alert = self.driver.switch_to_alert()
alert_text = alert.text
if self.accept_next_alert:
alert.accept()
else:
alert.dismiss()
return alert_text
finally: self.accept_next_alert = True
def tearDown(self):
self.driver.quit()
self.assertEqual([], self.verificationErrors)
if __name__ == "__main__":
unittest.main()
以這種方式為我工作(類似於@Amey 和@user4642224 代碼,但更短一點):
from selenium import webdriver
from selenium.webdriver.common.proxy import Proxy, ProxyType
prox = Proxy()
prox.proxy_type = ProxyType.MANUAL
prox.http_proxy = "ip_addr:port"
prox.socks_proxy = "ip_addr:port"
prox.ssl_proxy = "ip_addr:port"
capabilities = webdriver.DesiredCapabilities.CHROME
prox.add_to_capabilities(capabilities)
driver = webdriver.Chrome(desired_capabilities=capabilities)
這樣的事情怎么樣
PROXY = "149.215.113.110:70"
webdriver.DesiredCapabilities.FIREFOX['proxy'] = {
"httpProxy":PROXY,
"ftpProxy":PROXY,
"sslProxy":PROXY,
"noProxy":None,
"proxyType":"MANUAL",
"class":"org.openqa.selenium.Proxy",
"autodetect":False
}
# you have to use remote, otherwise you'll have to code it yourself in python to
driver = webdriver.Remote("http://localhost:4444/wd/hub", webdriver.DesiredCapabilities.FIREFOX)
您可以在此處閱讀更多相關信息。
我的解決方案:
def my_proxy(PROXY_HOST,PROXY_PORT):
fp = webdriver.FirefoxProfile()
# Direct = 0, Manual = 1, PAC = 2, AUTODETECT = 4, SYSTEM = 5
print PROXY_PORT
print PROXY_HOST
fp.set_preference("network.proxy.type", 1)
fp.set_preference("network.proxy.http",PROXY_HOST)
fp.set_preference("network.proxy.http_port",int(PROXY_PORT))
fp.set_preference("general.useragent.override","whater_useragent")
fp.update_preferences()
return webdriver.Firefox(firefox_profile=fp)
然后調用你的代碼:
my_proxy(PROXY_HOST,PROXY_PORT)
我在使用此代碼時遇到問題,因為我將字符串作為端口 # 傳遞:
PROXY_PORT="31280"
這個很重要:
int("31280")
您必須傳遞一個整數而不是字符串,否則您的 Firefox 配置文件將無法設置為正確的端口,並且通過代理的連接將無法正常工作。
如果有人正在尋找解決方案,方法如下:
from selenium import webdriver
PROXY = "YOUR_PROXY_ADDRESS_HERE"
webdriver.DesiredCapabilities.FIREFOX['proxy']={
"httpProxy":PROXY,
"ftpProxy":PROXY,
"sslProxy":PROXY,
"noProxy":None,
"proxyType":"MANUAL",
"autodetect":False
}
driver = webdriver.Firefox()
driver.get('http://www.whatsmyip.org/')
也嘗試設置 sock5 代理。 我遇到了同樣的問題,它是通過使用襪子代理解決的
def install_proxy(PROXY_HOST,PROXY_PORT):
fp = webdriver.FirefoxProfile()
print PROXY_PORT
print PROXY_HOST
fp.set_preference("network.proxy.type", 1)
fp.set_preference("network.proxy.http",PROXY_HOST)
fp.set_preference("network.proxy.http_port",int(PROXY_PORT))
fp.set_preference("network.proxy.https",PROXY_HOST)
fp.set_preference("network.proxy.https_port",int(PROXY_PORT))
fp.set_preference("network.proxy.ssl",PROXY_HOST)
fp.set_preference("network.proxy.ssl_port",int(PROXY_PORT))
fp.set_preference("network.proxy.ftp",PROXY_HOST)
fp.set_preference("network.proxy.ftp_port",int(PROXY_PORT))
fp.set_preference("network.proxy.socks",PROXY_HOST)
fp.set_preference("network.proxy.socks_port",int(PROXY_PORT))
fp.set_preference("general.useragent.override","Mozilla/5.0 (Macintosh; Intel Mac OS X 10_9_3) AppleWebKit/537.75.14 (KHTML, like Gecko) Version/7.0.3 Safari/7046A194A")
fp.update_preferences()
return webdriver.Firefox(firefox_profile=fp)
然后從您的程序中調用install_proxy ( ip , port )
。
帶驗證的代理。 這是一個全新的 Python 腳本,參考了 Mykhail Martsyniuk 示例腳本。
# Load webdriver
from selenium import webdriver
# Load proxy option
from selenium.webdriver.common.proxy import Proxy, ProxyType
# Configure Proxy Option
prox = Proxy()
prox.proxy_type = ProxyType.MANUAL
# Proxy IP & Port
prox.http_proxy = “0.0.0.0:00000”
prox.socks_proxy = “0.0.0.0:00000”
prox.ssl_proxy = “0.0.0.0:00000”
# Configure capabilities
capabilities = webdriver.DesiredCapabilities.CHROME
prox.add_to_capabilities(capabilities)
# Configure ChromeOptions
driver = webdriver.Chrome(executable_path='/usr/local/share chromedriver',desired_capabilities=capabilities)
# Verify proxy ip
driver.get("http://www.whatsmyip.org/")
嘗試設置 FirefoxProfile
from selenium import webdriver
import time
"Define Both ProxyHost and ProxyPort as String"
ProxyHost = "54.84.95.51"
ProxyPort = "8083"
def ChangeProxy(ProxyHost ,ProxyPort):
"Define Firefox Profile with you ProxyHost and ProxyPort"
profile = webdriver.FirefoxProfile()
profile.set_preference("network.proxy.type", 1)
profile.set_preference("network.proxy.http", ProxyHost )
profile.set_preference("network.proxy.http_port", int(ProxyPort))
profile.update_preferences()
return webdriver.Firefox(firefox_profile=profile)
def FixProxy():
""Reset Firefox Profile""
profile = webdriver.FirefoxProfile()
profile.set_preference("network.proxy.type", 0)
return webdriver.Firefox(firefox_profile=profile)
driver = ChangeProxy(ProxyHost ,ProxyPort)
driver.get("http://whatismyipaddress.com")
time.sleep(5)
driver = FixProxy()
driver.get("http://whatismyipaddress.com")
該程序在 Windows 8 和 Mac OSX 上都經過測試。 如果您使用的是 Mac OSX 並且沒有更新 selenium,那么您可能會遇到selenium.common.exceptions.WebDriverException
。 如果是這樣,請在升級 selenium 后重試
pip install -U selenium
上述結果可能是正確的,但不適用於最新的 webdriver。 這是我對上述問題的解決方案。 簡單又甜蜜
http_proxy = "ip_addr:port"
https_proxy = "ip_addr:port"
webdriver.DesiredCapabilities.FIREFOX['proxy']={
"httpProxy":http_proxy,
"sslProxy":https_proxy,
"proxyType":"MANUAL"
}
driver = webdriver.Firefox()
或者
http_proxy = "http://ip:port"
https_proxy = "https://ip:port"
proxyDict = {
"http" : http_proxy,
"https" : https_proxy,
}
driver = webdriver.Firefox(proxy=proxyDict)
上面和這個問題的答案要么在 Linux 上使用 Selenium 3.14 和 Firefox 68.9 對我不起作用,要么不必要地復雜。 我需要使用 WPAD 配置,有時在代理后面(在 VPN 上),有時不需要。 稍微研究了一下代碼后,我想出了:
from selenium import webdriver
from selenium.webdriver.common.proxy import Proxy
from selenium.webdriver.firefox.firefox_profile import FirefoxProfile
proxy = Proxy({'proxyAutoconfigUrl': 'http://wpad/wpad.dat'})
profile = FirefoxProfile()
profile.set_proxy(proxy)
driver = webdriver.Firefox(firefox_profile=profile)
代理初始化將 proxyType 設置為 ProxyType.PAC(從 URL 自動配置)作為副作用。
它還與 Firefox 的自動檢測一起使用,使用:
from selenium.webdriver.common.proxy import ProxyType
proxy = Proxy({'proxyType': ProxyType.AUTODETECT})
但我認為這不會像 WPAD 那樣同時適用於內部 URL(未代理)和外部(代理)。 類似的代理設置也適用於手動配置。 可以在此處的代碼中查看可能的代理設置。
請注意,將 Proxy 對象作為proxy=proxy
直接傳遞給驅動程序是行不通的——它被接受但被忽略(應該有一個棄用警告,但在我的情況下,我認為 Behave 正在吞噬它)。
驚訝地發現沒有使用經過身份驗證的代理的示例。
2022 年 10 月針對經過身份驗證的代理(Firefox 和 Chrome)的解決方案:
from selenium import webdriver
PROXY_HOST = "0.0.0.0";
PROXY_PORT = "0000"
PROXY_USERNAME = "user"
PROXY_PASS = "pass"
# If you're using Firefox
profile = webdriver.FirefoxProfile()
profile.set_preference("network.proxy.type", 1)
profile.set_preference("network.proxy.http",PROXY_HOST)
profile.set_preference("network.proxy.http_port", PROXY_PORT)
fp.set_preference('network.proxy.no_proxies_on', 'localhost, 127.0.0.1')
credentials = '%s:%s' % (PROXY_USERNAME, PROXY_PASS)
credentials = b64encode(credentials.encode('ascii')).decode('utf-8')
fp.set_preference('extensions.closeproxyauth.authtoken', credentials)
driver = webdriver.Firefox(firefox_profile=profile)
# If you're using Chrome
chrome_options = WebDriver.ChromeOptions()
options.add_argument('--proxy-server=http://%s:%s@%s:%s' % (PROXY_HOST, PROXY_PORT, PROXY_USERNAME, PROXY_PASS))
driver = webdriver.Chrome(executable_path='chromedriver.exe', chrome_options=chrome_options)
# Proxied request
driver.get("https://www.google.com")
一些有用的 proxy.network 資源與快速 Selenium 集成
這對我有用並允許使用無頭瀏覽器,您只需要調用傳遞代理的方法。
def setProxy(proxy):
options = Options()
options.headless = True
#options.add_argument("--window-size=1920,1200")
options.add_argument("--disable-dev-shm-usage")
options.add_argument("--no-sandbox")
prox = Proxy()
prox.proxy_type = ProxyType.MANUAL
prox.http_proxy = proxy
prox.ssl_proxy = proxy
capabilities = webdriver.DesiredCapabilities.CHROME
prox.add_to_capabilities(capabilities)
return webdriver.Chrome(desired_capabilities=capabilities, options=options, executable_path=DRIVER_PATH)
使用抓取 API 時,從任何在線來源抓取數據都非常容易。 您可以嘗試使用scraper API 從網頁中抓取信息,它會自動解析網頁數據。 API 也可以集成到您的源代碼中。 除了使用 API 抓取數據之外,您還可以嘗試使用美麗湯中下面提到的源代碼使用 CSS 選擇器抓取數據。 在嘗試此代碼之前,請注意 select() 方法可用於查找大量元素。 除此之外,要使用 select_one() 搜索單個元素。
源代碼:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
PROXY = "177.46.141.143:59393" #your proxy (ip address: port no)
chrome_options = WebDriverWait.ChromeOptions()
chrome_options.add_argument('--proxy-server=%s' % PROXY)
chrome = webdriver.Chrome(chrome_options=chrome_options)
chrome.get("https://www.ipchicken.com/")
正如@Dugini 所述,一些配置條目已被刪除。 最大:
webdriver.DesiredCapabilities.FIREFOX['proxy'] = {
"httpProxy":PROXY,
"ftpProxy":PROXY,
"sslProxy":PROXY,
"noProxy":[],
"proxyType":"MANUAL"
}
這是一個相當古老的帖子,但是,對於其他人來說,它可能仍然可以通過提供今天的答案而受益,但最初的作者非常接近一個可行的解決方案。
首先,此時不再支持ftpProxy設置,會報錯
proxy = Proxy({
'proxyType': ProxyType.MANUAL,
'httpProxy': myProxy,
'ftpProxy': myProxy, # this will throw an error
'sslProxy': myProxy,
'noProxy':''})
這在 2022 年 9 月對我有幫助 - 使用 Auth 用戶 + 密碼代理 selenium
import os
import zipfile
from selenium import webdriver
PROXY_HOST = '192.168.3.2' # rotating proxy or host
PROXY_PORT = 8080 # port
PROXY_USER = 'proxy-user' # username
PROXY_PASS = 'proxy-password' # password
manifest_json = """
{
"version": "1.0.0",
"manifest_version": 2,
"name": "Chrome Proxy",
"permissions": [
"proxy",
"tabs",
"unlimitedStorage",
"storage",
"<all_urls>",
"webRequest",
"webRequestBlocking"
],
"background": {
"scripts": ["background.js"]
},
"minimum_chrome_version":"22.0.0"
}
"""
background_js = """
var config = {
mode: "fixed_servers",
rules: {
singleProxy: {
scheme: "http",
host: "%s",
port: parseInt(%s)
},
bypassList: ["localhost"]
}
};
chrome.proxy.settings.set({value: config, scope: "regular"}, function() {});
function callbackFn(details) {
return {
authCredentials: {
username: "%s",
password: "%s"
}
};
}
chrome.webRequest.onAuthRequired.addListener(
callbackFn,
{urls: ["<all_urls>"]},
['blocking']
);
""" % (PROXY_HOST, PROXY_PORT, PROXY_USER, PROXY_PASS)
def get_chromedriver(use_proxy=False, user_agent=None):
path = os.path.dirname(os.path.abspath(__file__))
chrome_options = webdriver.ChromeOptions()
if use_proxy:
pluginfile = 'proxy_auth_plugin.zip'
with zipfile.ZipFile(pluginfile, 'w') as zp:
zp.writestr("manifest.json", manifest_json)
zp.writestr("background.js", background_js)
chrome_options.add_extension(pluginfile)
if user_agent:
chrome_options.add_argument('--user-agent=%s' % user_agent)
driver = webdriver.Chrome(
os.path.join(path, 'chromedriver'),
chrome_options=chrome_options)
return driver
def main():
driver = get_chromedriver(use_proxy=True)
driver.get('https://ifconfig.me/)
if __name__ == '__main__':
main()
2023 年 1 月解決方案,帶身份驗證和 Firefox 的 SSL
from selenium import webdriver
PROXY_HOST = "ip"
PROXY_PORT = "port"
PROXY_USERNAME = "username"
PROXY_PASS = "password"
profile = webdriver.FirefoxProfile()
profile.set_preference("network.proxy.type", 1)
profile.set_preference('network.proxy.ssl_port', int(PROXY_PORT))
profile.set_preference('network.proxy.ssl', PROXY_HOST)
profile.set_preference("network.proxy.http", PROXY_HOST)
profile.set_preference("network.proxy.http_port", int(PROXY_PORT))
profile.set_preference("network.proxy.no_proxies_on", 'localhost, 127.0.0.1')
profile.set_preference("network.proxy.socks_username", PROXY_USERNAME)
profile.set_preference("network.proxy.socks_password", PROXY_PASS)
profile.update_preferences()
driver = webdriver.Firefox(firefox_profile=profile)
driver.get("https://2ip.ru")
此外,您可能需要在代理提供商帳戶設置中鎖定您的 ip 地址。 否則,系統會提示您在頁面加載時輸入憑據。
嘗試運行 Tor 服務,將以下函數添加到您的代碼中。
def connect_tor(port):
socks.set_default_proxy(socks.PROXY_TYPE_SOCKS5, '127.0.0.1', port, True)
socket.socket = socks.socksocket
def main():
connect_tor()
driver = webdriver.Firefox()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.