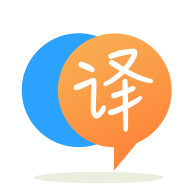
[英]Using a regular expression in JavaScript, how do I group my matches?
[英]How can I call a function from regular expression matches in javascript
我有一個正則表達式,我想知道是否可以將所有匹配項用作函數的參數。 例如,假設我有一個數據集
Hello heelo hhhheEEeloo eelloooo
和一個正則表達式
/[Hh]{1,}[Ee]{1,}[Ll]{1,}[Oo]{1,}/
哪個會匹配
Hello heelo hhhheEEeloo
我如何獲得一個javascript函數以將每個匹配項作為參數,例如
function isHello(arg) {
if (arg == 'Hello') { return 1 }
else { return 0}
}
將.replace
與回調一起使用
"Hello heelo hhhheEEeloo eelloooo".replace(/[Hh]{1,}[Ee]{1,}[Ll]{1,}[Oo]{1,}/g,function(match){
//Your function code here
return match;
})
或更簡單的例子:
var count=0;
"aaaaaaa".replace(/a/g,function(match){
console.log("I matched another 'a'",count++);
// just to not replace anything, technically this doesn't matter
//since it doesn't operate on the actual string
return match;
});
var string = "Hello heelo hhhheEEeloo eelloooo",
regex = /[Hh]{1,}[Ee]{1,}[Ll]{1,}[Oo]{1,}/g,
fn = function(arg){
if (arg == 'Hello')
return 1;
return 0
};
string.match(regex).forEach(fn);
注意添加到正則表達式中的g
標志進行匹配,以提供所需的匹配。
這是使用match()
的示例:
var s = "Hello heelo hhhheEEeloo eelloooo";
s.match(/[Hh]{1,}[Ee]{1,}[Ll]{1,}[Oo]{1,}/g).forEach(function(entry) {
// your function code here, the following is just an example
if (entry === "Hello")
console.log("Found Hello!");
else
console.log(entry + " is not Hello");
return;
});
示例: http : //jsfiddle.net/wTMuF/
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.