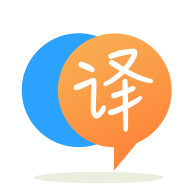
[英]How can I use AJAX to fetch data and store it in javascript variables?
[英]How do i use Javascript to “store” and “call” data?
我正在為假商店網站制作“目錄”頁面,當我單擊頁面上顯示的縮略圖之一時,Java腳本疊加層旨在顯示產品的所有信息(例如較大的圖像“ photo1')和該縮略圖(產品在SQL數據庫中)。
盡管這樣做確實可以按預期拉上疊加層,但它不僅得到了一個相關的“ photo1”,而是從表格中獲取了所有疊加層。
我從老師那里得到了幫助,但她什至沒有幫助,但是從我的收集中,我需要一種方法來存儲選擇的縮略圖,以便我可以弄清楚要疊加的信息。
主要:
<div id="overlay">
<div>
<?php
include 'includes/connection.php';
try {
$sql = 'SELECT * FROM item;';
$resultSet = $pdo->query($sql);
} // end try
catch (PDOException $e) {
echo 'Error fetching items: ' . $e->getMessage();
exit();
} // end catch
foreach ($resultSet as $row) {
// put each rows's elements into variables
$itemName = $row['itemName'];
$unitPrice = $row['unitPrice'];
$qtyOnHand = $row['qtyOnHand'];
$thumbNail = $row['thumbNail'];
$photo1 = $row['photo1'];
echo '<td><img src="' .$photo1 .'" width="500" height="500" alt="$itemName" title="$itemName" ></td>';
}
?>
<p>Content you want the user to see goes here.</p>
Click here to [<a href='#' onclick='overlay()'>close</a>]
</div>
</div>
<div id="MainHolder">
<div id="Headerbar">
<?php
include 'includes/login.php';
?>
<div class="ContentBody">
<div class="Content">
<article>
<h2>Store</h2>
<?php
include 'includes/connection.php';
try
{
$sql = 'SELECT * FROM item;';
$resultSet = $pdo->query($sql);
} // end try
catch (PDOException $e)
{
echo 'Error fetching items: ' . $e->getMessage();
exit();
} // end catch
?>
<!-- open table -->
<article>
<?php
// read result set and populate table
foreach ($resultSet as $row)
{
// put each rows's elements into variables
$itemName = $row['itemName'];
$thumbNail = $row['thumbNail'];
$qtyOnHand = $row['qtyOnHand'];
// test for out of stock condition
// if no stock, display out of stock image else display add to cart image
if ($qtyOnHand <= 0) {
echo '<td><img src="images/outOfStock.png" border="3" class="floatingImage" height="80" width="80" alt="" title=""></td>';
} else {
echo '<td><img class="floatingImage" border="3" src="' .$thumbNail .'" width="80" height="80" alt="' .$itemName .'" title="' .$itemName .'" onclick="overlay()" ></td>';
}
} // end foreach
// close table
echo '</article>';
?>
</article>
</div>
<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>
</div>
</div>
</div>
使用Javascript:
function overlay() {
el = document.getElementById("overlay");
el.style.visibility = (el.style.visibility == "visible") ? "hidden" : "visible";
}
疊加層和目錄位於同一文件中。 我仍在學習,因此對任何混亂的格式/代碼表示歉意。
編輯:我已經合並了一些代碼,這幾乎顯示了我的整個商店頁面,而不是標題,等等...
您需要編輯覆蓋函數,以將itemName發送到服務器,這將告訴您的服務器用戶單擊了哪個項目。
疊加功能:
function overlay() {
var item = this.getAttribute("title");//this gets the name of item that was clicked
}
現在我們需要向服務器設置一個ajax請求。
function ajaxRequest(item) {
if (window.XMLHttpRequest){
var xmlhttp= new XMLHttpRequest();
xmlhttp.onreadystatechange=function(){
if (xmlhttp.readyState==4 && xmlhttp.status == 200){//show the overlay after we recieve a response from the server
el = document.getElementById("overlay");
el.style.visibility = (el.style.visibility == "visible") ? "hidden" : "visible";
el.innerHtml = ''; //remove previous response
el.innerHTML=xmlhttp.responseText; //insert the response in your overlay
}
}
xmlhttp.open("GET","overlay.php?item="+ encodeURIComponent(item),true);
xmlhttp.send();
}
}
現在,我們需要編輯overlay函數來調用ajaxRequest函數:
function overlay() {
var item = this.getAttribute("title");//this gets the name of item that was clicked
ajaxRequest(item); //send the item name to server
}
完成此操作后,您的PHP將在您的服務器上收到一個變量。 創建一個名為overlay.php的新文件,並插入以下代碼。 將此文件保存在與Store.php文件相同的目錄中。
overlay.php:
<?php
if (isset($_GET['item'])) {//check if you received the name
$itemName = $_GET['item'];
//query database for the itemName
try
{
$sql = 'SELECT * FROM item WHERE itemName ='.$itemName.';';//just select data with the matching item name.
$resultSet = $pdo->query($sql);
} // end try
catch (PDOException $e)
{
echo 'Error fetching items: ' . $e->getMessage();
exit();
} // end catch
//fetch the data
foreach ($resultSet as $row) {
// put each rows's elements into variables
$itemName = $row['itemName'];
$unitPrice = $row['unitPrice'];
$qtyOnHand = $row['qtyOnHand'];
$thumbNail = $row['thumbNail'];
$photo1 = $row['photo1'];
echo '<td><img src="' .$photo1 .'" width="500" height="500" alt="$itemName" title="$itemName" ></td>';
}//end foreach
}//end if
?>
如果要絕對在客戶端存儲數據,則可以將html5的本地存儲功能與嵌入式sql lite數據庫一起使用,以使用Javascript存儲和檢索信息。
首次使用此循環從數據庫獲取產品時:
foreach ($resultSet as $row) {
// put each rows's elements into variables
$itemName = $row['itemName'];
$unitPrice = $row['unitPrice'];
$qtyOnHand = $row['qtyOnHand'];
$thumbNail = $row['thumbNail'];
$photo1 = $row['photo1'];
echo '<td><img src="' .$photo1 .'" width="500" height="500" alt="$itemName" title="$itemName" ></td>';
}
當用戶單擊疊加層的圖像時,是否要顯示所有這些屬性? 如果是這樣,只需將值存儲在實際的<img>
標記中:
echo '<td><img src="' .$photo1 .'" width="500" height="500" alt="$itemName" title="$itemName" data-unit-price="$unitPrice" data-qty="$qtyOnhand"></td>';
然后,您可以使用Javascript訪問數據並在疊加層中顯示。
我嘗試了解您的問題,並使用jQuery解決了它。
首先,您必須加載jQuery lib
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
並在疊加中的每個生產信息中添加display:none樣式(默認為隱藏)。
echo '<td><img src="' .$photo1 .'" width="500" height="500" alt="$itemName" title="$itemName" style="display:none" ></td>';
刪除縮略圖內聯onClick事件觸發器
echo '<td><img class="floatingImage" border="3" src="' .$thumbNail .'" width="80" height="80" alt="' .$itemName .'" title="' .$itemName.'"'></td>';
添加此單擊事件處理程序,以便jQuery可以顯示用戶單擊的生產信息。
$(".floatingImage").click(function(){
var itemName = $(this).attr('alt');
$('#overlay img').hide();
$('#overlay img[alt="'+itemName+'"]').show();
overlay();
});
希望這對您有幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.