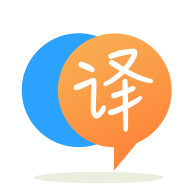
[英]Wicket ListView ajax removes always removes last element in list
[英]Removes first element but want it to remove last element
在這段代碼中,我想使用removeLast從列表中刪除最后一個對象。 但是它刪除了第一個元素。 如何使用removeLast方法刪除最后一個元素。 https://stackoverflow.com/editing-help
class List {
Customer listPtr;
int index;
public void add(Customer customer) {
Customer temp = customer;
if (listPtr == null) {
listPtr = temp;
index++;
} else {
Customer x = listPtr;
while (x.next != null) {
x = x.next;
}
x.next = temp;
index++;
}
}
public void removeLast() {
Customer temp = listPtr;
if (listPtr != null) {
listPtr = temp.next;
}
}
public void removeAll() {
Customer temp = listPtr;
while (temp != null) {
listPtr = temp.next;
temp = temp.next;
}
}
public int size() {
int size = 0;
Customer temp = listPtr;
while (temp != null) {
size++;
temp = temp.next;
}
return size;
}
public void printList() {
Customer temp = listPtr;
while (temp != null) {
System.out.println(temp);
temp = temp.next;
}
}
}
class DemoList {
public static void main(String args[]) {
List list = new List();
Customer c1 = new Customer("10011", "Jason");
Customer c2 = new Customer("10012", "George");
Customer c3 = new Customer("10013", "Sam");
list.add(c1);
list.add(c2);
list.add(c3);
list.removeLast();
list.printList();
}
}
class Customer {
String id;
String name;
Customer next;
public Customer(String id, String name) {
this.id = id;
this.name = name;
}
public String toString() {
return id + " : " + name;
}
public boolean equals(Object ob) {
Customer c = (Customer) ob;
return this.id.equals(c.id);
}
}
您還需要跟蹤倒數第二個元素
public void removeLast() {
if (listPtr != null) {
Customer x1 = listPtr;
Customer x2 = listPtr;
while (x2.next != null) {
x1 = x2;
x2 = x2.next;
}
x1.next = null; // delete last
index--;
}
}
您當前的代碼實際上只是將指針在列表中上移了一個
Customer temp = listPtr;
if (listPtr != null) {
listPtr = temp.next; // point to second element
}
問題是您需要獲取的元素不是最后一個,而是倒數第二個。 這樣,您可以告訴該元素“下一個”為null。
以下代碼可能會得到改進,但是該解決方案將為您服務。
public void removeLast() {
Customer temp = listPtr;
while(temp.next.next!=null){
temp = temp.next;
}
temp.next=null;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.