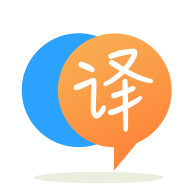
[英]How to make the soft keyboard hide when clicked outside of the focused EditText BUT not if another EditText is clicked?
[英]How to make EditText not focused when creating Activity
我已經閱讀了討論這個問題的其他問題,除了第一個創建的布局之外,所有這些問題都適用於我的布局。
目前,這是我的onCreate
方法的頂部:
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN);
^ 這使得至少鍵盤不會在啟動時彈出,但EditText
仍然專注於。
這是我的EditText
的XML
:
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/changePass"
android:layout_centerHorizontal="true"
android:layout_marginTop="167dp"
android:ems="10"
android:imeOptions="flagNoExtractUi"
android:inputType="textPassword"
android:maxLength="30" >
</EditText>
這是我提出活動時的樣子:
問題在於,對於某些手機,當EditText
像這樣聚焦時,它們無法在其中寫入。 我希望它不集中。
我所做的工作適用於下一個布局,因為EditTexts
沒有關注它,並且看起來更像這樣:
請注意,它是相同的布局。 這是用戶返回此屏幕后的屏幕,這表明XML
沒有任何問題,因為這是相同的XML
,但問題是EditText
僅在創建活動時才關注。
我已經完成了研究,所有其他問題都沒有幫助我解決這個問題(但是他們確實幫助鍵盤沒有出現,謝天謝地)。 我怎樣才能使啟動時的EditText
看起來像第二個屏幕截圖,而不是第一個屏幕截圖?
您可以設置布局的屬性,如android:descendantFocusability="beforeDescendants"
和android:focusableInTouchMode="true"
例子:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/mainLayout"
android:descendantFocusability="beforeDescendants"
android:focusableInTouchMode="true" >
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/changePass"
android:layout_centerHorizontal="true"
android:layout_marginTop="167dp"
android:ems="10"
android:imeOptions="flagNoExtractUi"
android:inputType="textPassword"
android:maxLength="30" >
</EditText>
</RelativeLayout>
願這有幫助;)
XML 代碼:
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/changePass"
android:layout_centerHorizontal="true"
android:layout_marginTop="167dp"
android:ems="10"
android:focusable="false"
android:imeOptions="flagNoExtractUi"
android:inputType="textPassword"
android:maxLength="30" >
</EditText>
Java代碼:
EditText edPwd = (EditText)findViewById(R.id.password);
edtPwd.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
v.setFocusable(true);
v.setFocusableInTouchMode(true);
return false;
}
});
在 xml 中設置可聚焦的 false 並通過代碼將其設置為 true
在你的 main_layout 添加這 2 行:
android:descendantFocusability="beforeDescendants"
android:focusableInTouchMode="true"
例子:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:descendantFocusability="beforeDescendants"
android:focusableInTouchMode="true"> *YOUR LAYOUT CODE* </RelativeLayout>
最好的解決方案在這里: https ://stackoverflow.com/a/45139132/3172843
正確而簡單的解決方案是 setFocusable false 和setFocusableInTouchMode true 。 所以只有當用戶觸摸 EditText 時 EditText 才會獲得焦點
android:focusable="false"
android:focusableInTouchMode="true"
在 onCreate() 中添加這個
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN);
或在 onCreateView()
getActivity().getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN);
XML 代碼
<EditText
android:imeOptions="flagNoExtractUi"
android:focusable="false"
/>
onClickListerner 中的 Java 代碼
mEdtEnterPhoneNumber.setFocusable(true);
mEdtEnterPhoneNumber.setFocusableInTouchMode(true);
每當我在 Spinner 的選擇項之后嘗試打開 Editext 時,所以當時無法打開鍵盤並寫下 Edit 文本中的值,但我用這段代碼解決了我的問題。
android:descendantFocusability="beforeDescendants"
安卓:focusableInTouchMode="true"
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:descendantFocusability="beforeDescendants"
android:focusableInTouchMode="true">
<androidx.appcompat.widget.AppCompatEditText
android:id="@+id/titleName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textCapWords"
android:hint="@string/hint_your_full_name"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
謝謝!
可以使用android:focusable="false"
來禁用它,但如果由於某種原因這不起作用,那么您可以簡單地放置一個LinearLayout
,它會在不破壞您的布局的情況下獲得焦點。
注意:Eclipse 會給你一個錯誤,說你的LinearLayout
是無用的,因為它沒有內容。 您應該可以毫無問題地忽略它。
例如:
<LinearLayout
android:focusable="true"
android:focusableInTouchMode="false"
android:layout_width="0dp"
android:layout_height="0dp"
/>
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/changePass"
android:layout_centerHorizontal="true"
android:layout_marginTop="167dp"
android:ems="10"
android:imeOptions="flagNoExtractUi"
android:inputType="textPassword"
android:maxLength="30" >
</EditText>
</LinearLayout>
您可以以編程方式執行此操作。 在您的活動的onStart()
方法中使用editText.setEnabled(false)
(而不是在 onCreate() - 因為這是一些用於初始化 GUI 組件的方法)
<activity
android:name=".MainActivity"
android:windowSoftInputMode="stateAlwaysHidden" />
android:windowSoftInputMode="stateAlwaysHidden"
將此行添加到 Manifest.xml 文件的活動標記中。當您單擊 EditText 時,鍵盤將成為焦點。
最簡單的方法是添加
android:windowSoftInputMode="stateAlwaysHidden|adjustPan"
在 Manifest.xml 文件的活動標記中
1)它最簡單,打開清單並將以下代碼放在活動標簽之間:
android:windowSoftInputMode="stateHidden"
2)將此屬性放在父布局中
android:focusable="true"
android:focusableInTouchMode="true"
在 Manifest 中,復制並粘貼下面的代碼。
<activity
android:name=".LoginActivity"
android:windowSoftInputMode="stateAlwaysHidden"/>
使用android:focusable="false"
禁用對焦
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/changePass"
android:layout_centerHorizontal="true"
android:layout_marginTop="167dp"
android:ems="10"
android:imeOptions="flagNoExtractUi"
android:inputType="textPassword"
android:maxLength="30"
android:focusable="false" >
</EditText>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.