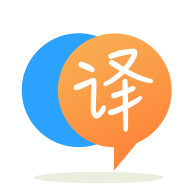
[英]How can I upload files to google cloud storage from my server to google's server using PHP to backup my server files?
[英]How do I upload both form data and multiple files from my android app, using HTTPURLConnection to my server, where I am using PHP?
我有一個向服務器發送1個或多個zip文件的android應用程序。 我想隨文件一起發送一些其他表單數據,因為表單數據將用於確定文件的配置。
我已經能夠使用多部分內容類型上傳文件,但是我似乎無法在服務器端獲取php來查看表單數據和文件。
我想知道的是如何使用Android端的HTTPUrlConnection和服務器端的PHP將表單數據和文件列表發送到服務器。
我相信我的問題與Content-Type和Content-Disposition有關。 如果有人可以向我指出一個明確的例子,或解釋我做錯了什么,我將非常感激。
感謝您抽出寶貴的時間來研究這個問題。”
這是最新的,經過修訂的android代碼:
String postDriverUpload(File[] fileList) {
HttpURLConnection connection = null;
DataOutputStream outputStream = null;
String tag = TAG + "postDriverInfo()";
URL url = null;
String result = OK_TO_CONTINUE;
String targetUrl = server + "/upload.php";
try {
url = new URL(targetUrl);
} catch (MalformedURLException e) {
result = "ERROR: [" + targetUrl + "] can't be parsed into a URL.";
Log.e(tag, result, e);
return result;
}
try {
connection = (HttpURLConnection) url.openConnection();
} catch (IOException e) {
result = "ERROR: IOException.";
Log.e(tag, result, e);
return result;
}
// Allow Inputs and Outputs
connection.setDoInput(true);
connection.setDoOutput(true);
connection.setUseCaches(false);
// Enable POST method
try {
connection.setRequestMethod("POST");
} catch (ProtocolException e) {
result = "Error: Protocol Exception.";
Log.e(tag, result, e);
return result;
}
connection.setRequestProperty("Connection", "Keep-Alive");
connection.setRequestProperty("Content-Type",
"multipart/form-data;boundary=" + BOUNDARY);
// Encode driver userName
List<NameValuePair> nameValuePairs = new ArrayList<NameValuePair>();
try {
nameValuePairs.add(new BasicNameValuePair("userName", driver
.getUserName()));
Log.d(tag, "Encoded userName='driver' is: "
+ encodeData(nameValuePairs));
// Create output Stream to send data to server
outputStream = new DataOutputStream(connection.getOutputStream());
writeBoundary(outputStream);
outputStream
.writeBytes("Content-Disposition: form-data; name=\"userName\" "
+ EOL + EOL);
outputStream.writeBytes(driver.getUserName() + EOL);
writeBoundary(outputStream);
// Starting the Files part
outputStream
.writeBytes("Content-Disposition: form-data; name=\"files\" "
+ EOL + EOL);
outputStream.writeBytes("Content-Type: multipart/mixed; boundary="
+ FILE_BOUNDARY + EOL);
// Adding the file parts
for (File file : fileList) {
writeFileBoundary(outputStream);
// write out file
String contentType = String.format(
"Content-Disposition:file; filename=\"%s\"" + EOL
+ "Content-Type: application/x-zip-compressed",
file.getName())
+ EOL + EOL;
Log.d(tag, "Content-Type = " + contentType);
outputStream.write(contentType.getBytes());
}
writeFileBoundary(outputStream);
writeBoundary(outputStream);
outputStream.flush();
outputStream.close();
} catch (UnsupportedEncodingException e) {
result = "Error: UnsupportedEncodingException ";
Log.e(tag, result, e);
return result;
} catch (IOException e) {
result = "Error: IOException ";
Log.e(tag, result, e);
return result;
}
// Get Response from server (code and message)
try {
int serverResponseCode = connection.getResponseCode();
String serverResponseMessage = connection.getResponseMessage();
Log.d(tag, "Response Code = " + serverResponseCode);
Log.d(tag, "Response Message = " + serverResponseMessage);
} catch (IOException e) {
result = "Error getting response from server.";
Log.e(tag, result, e);
return result;
}
final StringBuilder out = new StringBuilder();
try {
BufferedReader in = new BufferedReader(new InputStreamReader(
connection.getInputStream()));
String line;
while (null != (line = in.readLine())) {
if (line.contains("ERROR")) {
Log.d(tag, "HTTPResponse: " + out.toString());
result = "Error: HTTPResponse: " + out.toString();
break;
}
out.append(line + "\n\n");
}
Log.d(tag, "HTTPResponse: " + out.toString());
} catch (IOException e) {
Log.w(tag, "WARNING: ", e);
}
return result;
}
void writeBoundary(DataOutputStream out) throws IOException {
final String twoHyphens = "--";
out.writeBytes(twoHyphens + BOUNDARY + EOL);
}
void writeFileBoundary(DataOutputStream out) throws IOException {
final String twoHyphens = "--";
out.writeBytes(twoHyphens + FILE_BOUNDARY + EOL);
}
這是我在Android端登錄的輸出。 如您所見,鍵,用戶名和值rbenjamin已正確加載到$ _POST變量中,但是到那時為止還不正確。 我用BOUNDARY分隔了下一部分,但是它似乎被變量'files'的值所占用。 我已經接近了,但是我仍然想念一些東西。 (我在下面的日志文件中去除了大部分噪音。)
: Upload Button Clicked.
: Compiling upload file
: getting delivered SQL = SELECT * FROM deliveryorder WHERE delivereddatetime != ''
: uploadEnabled = true
: downloadEnabled = false
: driverEmail = ray.benjamin@gmail.com
: Starting, getting database.
: Found 1 drivers.
: Processing driver 1
: user name is rbenjamin
: email is ray.benjamin@gmail.com
: Uploading files using HTTP to http://192.168.1.17:8080
: There are 16 files.
: Checking download - enabled? false
: Encoded userName='driver' is: userName=rbenjamin
: Content-Type = Content-Disposition:file; filename="upload20130820_1015.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130820_1048.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1624.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1628.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1645.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1647.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1705.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1709.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1746.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1801.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1804.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1811.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1813.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_1821.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130821_2050.zip"
: Content-Type: application/x-zip-compressed
:
: Content-Type = Content-Disposition:file; filename="upload20130825_1957.zip"
: Content-Type: application/x-zip-compressed
:
: Response Code = 200
: Response Message = OK
: HTTPResponse: <!DOCTYPE html>
: <html>
: <body>
: POST is defined.<br>Key = userName"_, Value = rbenjamin<br>Key = files"_, Value = Content-Type: multipart/mixed; boundary=**FILE*BOUNDARY***25WLqf@***
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130820_1015.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130820_1048.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1624.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1628.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1645.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1647.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1705.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1709.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1746.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1801.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1804.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1811.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1813.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_1821.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130821_2050.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***
: Content-Disposition:file; filename="upload20130825_1957.zip"
: Content-Type: application/x-zip-compressed
: --**FILE*BOUNDARY***25WLqf@***<br>
: Notice: Undefined index: userName in /var/www-pbs/upload.php on line 15
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: User Name is: <br>FILES is defined.<br>
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 24
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: <p>Dumping _FILES</p>
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 34
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 40
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Upload: <br>
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 41
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Type : <br>
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 42
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Size : 0 kB<br>
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 43
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Stored in:
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 45
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: Notice: Undefined index: file in /var/www-pbs/upload.php on line 47
: Call Stack:
: 0.0083 638888 1. {main}() /var/www-pbs/upload.php:0
: already exists.
: </body>
: </html>
: 200
沒有人回答我的問題,但是我最終在這里找到了我需要的東西: 一篇有關以編程方式發送多部分表單的www.codejava.net文章 。 這正是醫生的命令。 本文為您提供了一個MultipartUtility類,使您可以輕松使用HTTPURLConnection進行上傳。 這不僅是一個答案,而且是一個結構良好的答案。 對於Android,我不得不對其進行一些調整,但調整不了多少。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.