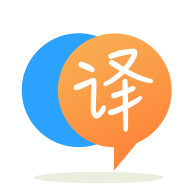
[英]How to compare two lists (list2 with list1 and find how many elements are <= list1) in Python
[英]Iterate over two nested 2D lists where list2 has list1's row numbers
我是Python的新手。 所以我想用循環來完成這個,而不使用像發電機這樣的奇特東西。 我有兩個2D數組,一個整數數組和另一個字符串數組,如下所示:
整數2D列表:
這里,dataset2d [0] [0]是表中的行數,dataset [0] [1]是列數。 所以下面的2D列表有6行4列
dataset2d = [ [6, 4], [0, 0, 0, 1], [1, 0, 2, 0], [2, 2, 0, 1], [1, 1, 1, 0], [0, 0, 1, 1], [1, 0, 2, 1] ]
字符串2D列表:
partition2d = [ ['A', '1', '2', '4'], ['B', '3', '5'], ['C', '6'] ]
partition[*][0]
即第一列是標簽。 對於A組,1,2和4是我需要從dataset2d中獲取的行號並應用公式。 所以這意味着我將讀取1,轉到dataset2d
的第1行並讀取第一列值,即dataset2d[1][0]
,然后我將從partition2d
讀取2,轉到數據集2d的第2行並讀取第一列,即dataset2d[2][0]
。 同樣下一個,我將閱讀dataset2d[4][0]
。
然后我將進行一些計算,獲取一個值並將其存儲在2D列表中,然后轉到dataset2d中的下一列以獲取這些行。 因此,在此示例中,讀取的下一列值將是dataset2d[1][1]
, dataset2d[2][1]
, dataset2d[4][1]
。 然后再做一些計算並為該列獲取一個值,存儲它。 我會這樣做,直到我到達dataset2d
的最后一列。
partition2d
的下一行是[B, 3, 5]
。 所以我將從dataset2d[3][0]
, dataset2d[5][0]
。 獲取該列的值為公式。 然后是真實的dataset2d [3][1]
, dataset2d[5][1]
等等,直到我到達最后一列。 我這樣做,直到讀取partition2d中的所有行。
我嘗試了什么:
for partitionRow in partition2d:
for partitionCol in partitionRow:
for colDataset in dataset2d:
print dataset2d[partitionCol][colDataset]
我面臨的問題是什么:
UPDATE1:
我正在從文本文件中讀取內容,2D列表中的數據可能會有所不同,具體取決於文件內容和大小,但file1即dataset2d和file2即partition2d的結構將是相同的。
Update2:因為Eric詢問輸出應該是什么樣子。
0.842322 0.94322 0.34232 0.900009 (For A)
0.642322 0.44322 0.24232 0.800009 (For B)
這只是一個例子,數字是我隨機輸入的。 因此,第一個數字0.842322是將公式應用於dataset2d的第0列的結果,即對於已考慮行1,2,4的A組的dataset2d [parttionCol] [0]。
第二個數字0.94322是將公式應用於dataset2d的第1列的結果,即對於已考慮行1,2 4的A組的dataset2d [partitionCol] [1]。
第三個數字,0.34232是將公式應用於dataset2d的第2列的結果,即數據集2d [partitionCol] [2],對於已考慮行1,2的組A.同樣,我們得到0.900009。
第二行中的第一個數字,即0.642322是將公式應用於dataset2d的第0列的結果,即對於考慮了行3,5的組B的dataset2d [parttionCol] [0]。 等等。
你可以使用Numpy (我希望這不適合你):
import numpy
dataset2D = [ [6, 4], [0, 0, 0, 1], [1, 0, 2, 0], [2, 2, 0, 1], [1, 1, 1, 0], [0, 0, 1, 1], [1, 0, 2, 1] ]
dataset2D_size = dataset2D[0]
dataset2D = numpy.array(dataset2D)
partition2D = [ ['A', '1', '2', '4'], ['B', '3', '5'], ['C', '6'] ]
for partition in partition2D:
label = partition[0]
row_indices = [int(i) for i in partition[1:]]
# Take the specified rows
rows = dataset2D[row_indices]
# Iterate the columns (this is the power of Python!)
for column in zip(*rows):
# Now, column will contain one column of data from specified row indices
print column, # Apply your formula here
print
或者如果你不想安裝Numpy ,你可以做到這一點(實際上這就是你想要的):
dataset2D = [ [6, 4], [0, 0, 0, 1], [1, 0, 2, 0], [2, 2, 0, 1], [1, 1, 1, 0], [0, 0, 1, 1], [1, 0, 2, 1] ]
partition2D = [ ['A', '1', '2', '4'], ['B', '3', '5'], ['C', '6'] ]
dataset2D_size = dataset2D[0]
for partition in partition2D:
label = partition[0]
row_indices = [int(i) for i in partition[1:]]
rows = [dataset2D[row_idx] for row_idx in row_indices]
for column in zip(*rows):
print column,
print
兩者都會打印:
(0, 1, 1) (0, 0, 1) (0, 2, 1) (1, 0, 0) (2, 0) (2, 0) (0, 1) (1, 1) (1,) (0,) (2,) (1,)
第二個代碼的解釋(沒有Numpy) :
[dataset2D[row_idx] for row_idx in row_indices]
這基本上是你采取每一行( dataset2D[row_idx]
)並將它們整理為一個列表。 所以這個表達式的結果是一個列表列表(來自指定的行索引)
for column in zip(*rows):
然后zip(*rows)
將逐列迭代 (您想要的那個)。 這通過獲取每一行的第一個元素,然后將它們組合在一起形成一個元組來工作 。 在每次迭代中,結果存儲在變量column
。
然后for column in zip(*rows):
的for column in zip(*rows):
你已經從指定的行中獲得了預期的列式迭代元素!
要應用您的公式,只需將print column,
更改為您想要的內容。 例如,我修改代碼以包含行號和列號:
print 'Processing partition %s' % label
for (col_num, column) in enumerate(zip(*rows)):
print 'Column number: %d' % col_num
for (row_num, element) in enumerate(column):
print '[%d,%d]: %d' % (row_indices[row_num], col_num, element)
這將導致:
Processing partition A Column number: 0 [1,0]: 0 [2,0]: 1 [4,0]: 1 Column number: 1 [1,1]: 0 [2,1]: 0 [4,1]: 1 Column number: 2 [1,2]: 0 [2,2]: 2 [4,2]: 1 Column number: 3 [1,3]: 1 [2,3]: 0 [4,3]: 0 Processing partition B Column number: 0 [3,0]: 2 [5,0]: 0 Column number: 1 [3,1]: 2 [5,1]: 0 Column number: 2 [3,2]: 0 [5,2]: 1 Column number: 3 [3,3]: 1 [5,3]: 1 Processing partition C Column number: 0 [6,0]: 1 Column number: 1 [6,1]: 0 Column number: 2 [6,3]: 2 Column number: 3 [6,3]: 1
我希望這有幫助。
這是使用迭代器的可擴展解決方案:
def partitions(data, p):
for partition in p:
label = partition[0]
row_indices = partition[1:]
rows = [dataset2D[row_idx] for row_idx in row_indices]
columns = zip(*rows)
yield label, columns
for label, columns in partitions(dataset2D, partitions2d):
print "Processing", label
for column in columns:
print column
partition2d是一個字符串數組,我需要跳過第一列,其中包含A,B,C等字符。
這稱為切片:
for partitionCol in partitionRow[1:]:
上面的代碼段將跳過第一列。
for colDataset in dataset2d:
已經做了你想要的。 這里沒有像C ++循環那樣的結構。 雖然你可以用Unpythonic方式做事:
i=0
for i in range(len(dataset2d)):
print dataset2d[partitionCol][i]
i=+1
這是一種非常糟糕的做事方式。 對於數組和矩陣,我建議你不要重新發明輪子(也就是Pythonic的東西), 看看Numpy
。 特別是在: numpy.loadtxt
解決你的問題:
我面臨的問題是什么:
- partition2d是一個字符串數組,我需要跳過第一列,其中包含A,B,C等字符。
- 我想僅在partition2d中給出的行號上以列為單位迭代dataset2d。 因此,只有在完成該列之后,colDataset才會增加。
問題1可以使用切片來解決 - 如果你想從第二個元素迭代partition2d,你只能for partitionCol in partitionRow[1:]
。 這將從第二個元素開始切片到結尾。
所以類似於:
for partitionRow in partition2d:
for partitionCol in partitionRow[1:]:
for colDataset in dataset2d:
print dataset2d[partitionCol][colDataset]
問題2我不明白你想要什么:)
建立:
d = [[6,4],[0,0,0,1],[1,0,2,0],[2,2,0,1],[1,1,1,0],[0,0,1,1],[1,0,2,1]]
s = [['A',1,2,4],['B',3,5],['C',6]]
結果列入清單l
l = []
for r in s: #go over each [character,index0,index1,...]
new_r = [r[0]] #create a new list to values given by indexN. Add in the character by default
for i,c in enumerate(r[1:]): #go over each indexN. Using enumerate to keep track of what N is.
new_r.append(d[c][i]) #i is now the N in indexN. c is the column.
l.append(new_r) #add that new list to l
導致
>>> l
[['A', 0, 0, 1], ['B', 2, 0], ['C', 1]]
第一次迭代的執行如下所示:
for r in s:
#-> r = ['A',1,2,4]
new_r = [r[0]] #= ['A']
for i,c in enumerate([r[1:] = [1,2,4])
#-> i = 0, c = 1
new_r.append(d[1][i])
#-> i = 1, c = 2
#...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.