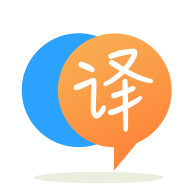
[英]Finding all combinations of words of length 2 from list of words using python
[英]finding all unique words from a list using loops
我正在嘗試根據從文本文件中提取的所有單詞列表制作唯一單詞列表。 我唯一的問題是用於迭代兩個列表的算法。
def getUniqueWords(allWords):
uniqueWords = []
uniqueWords.append(allWords[0])
for i in range(len(allWords)):
for j in range(len(uniqueWords)):
if allWords[i] == uniqueWords[j]:
pass
else:
uniqueWords.append(allWords[i])
print uniqueWords[j]
print uniqueWords
return uniqueWords
如您所見,我創建了一個空列表並開始遍歷兩個列表。 此外,我附加了列表中的第一項,因為出於某種原因,它不會嘗試嘗試匹配我假設的單詞,因為在空列表中,list[0] 不存在。 如果有人能幫我弄清楚如何正確地迭代這個,這樣我就可以生成一個很棒的單詞列表。
打印 uniqueWords[j] 只是為了調試,所以我可以看到在處理列表過程中出現了什么
我不是 python 專家,但認為這應該有效:
uniqueWords = []
for i in allWords:
if not i in uniqueWords:
uniqueWords.append(i);
return uniqueWords
編輯:
我測試過並且它有效,它只返回列表中的唯一單詞:
def getUniqueWords(allWords) :
uniqueWords = []
for i in allWords:
if not i in uniqueWords:
uniqueWords.append(i)
return uniqueWords
print getUniqueWords(['a','b','c','a','b']);
['a', 'b', 'c']
我不喜歡(嘗試)要求您選擇糟糕算法的作業問題。 例如,更好的選擇是使用set
或trie
。
您可以通過 2 個小的更改來修復您的程序
def getUniqueWords(allWords):
uniqueWords = []
uniqueWords.append(allWords[0])
for i in range(len(allWords)):
for j in range(len(uniqueWords)):
if allWords[i] == uniqueWords[j]:
break
else:
uniqueWords.append(allWords[i])
print uniqueWords[j]
print uniqueWords
return uniqueWords
首先,當您看到單詞已經存在時,您需要停止循環
for j in range(len(uniqueWords)):
if allWords[i] == uniqueWords[j]:
break # break out of the loop since you found a match
第二種是使用for
/ else
構造而不是if
/ else
for j in range(len(uniqueWords)):
if allWords[i] == uniqueWords[j]:
break
else:
uniqueWords.append(allWords[i])
print uniqueWords[j]
也許你可以使用 collections.Counter 類? (特別是如果您還想計算每個單詞在源文檔中出現的次數)。
http://docs.python.org/2/library/collections.html?highlight=counter#collections.Counter
import collections.Counter
def getUniqueWords(allWords):
uniqueWords = Counter()
for word in allWords:
uniqueWords[word]+=1
return uniqueWords.keys()
另一方面,如果你只是想統計的話,就用一個集合:
def getUniqueWords(allWords):
uniqueWords =set()
for word in allWords:
uniqueWords.add(word)
return uniquewords #if you want to return them as a set
OR
return list(uniquewords) #if you want to return a list
如果您僅限於循環,並且輸入相對較大,那么循環 + 二進制搜索是比僅循環更好的選擇 - 類似這樣:
def getUniqueWords(allWords):
uw = []
for word in allWords:
(lo,hi) = (0,len(uw)-1)
m = -1
while hi>=lo and m==-1:
mid = lo + (hi-lo)/2
if uw[mid]==word:
m = mid
elif uw[mid]<word:
lo = mid+1
else:
hi = mid-1
if m==-1:
m = lo
uw = uw[:m]+[word]+uw[m:]
return uw
如果您的輸入有大約 100000 個單詞,則使用此循環與簡單循環的區別在於,您的 PC 在執行程序時不會發出噪音:)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.