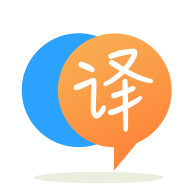
[英]How to unshift or add to the beginning of arguments object in JavaScript
[英]How to add a property at the beginning of an object in javascript
我有
var obj = {'b': 2, 'c': 3};
我想在該對象的開頭(而不是結尾)添加一個屬性:
var obj = {'a': 1, 'b': 2, 'c': 3};
有沒有干凈的方法來做到這一點?
您還可以在 ES6 (ES2015+) 中使用Object.assign( )。
let obj = {'b': 2, 'c': 3};
const returnedTarget = Object.assign({a: 1}, obj);
// Object {a: 1, b: 2, c: 3}
這些天你可以在 ES6 (ES2015+) 中使用很酷的擴展運算符 (...),嘗試以下操作:
const obj = {'b': 2, 'c': 3}; const startAdded = {'a':1 , ...obj}; console.log(startAdded); const endAdded = {...obj, 'd':4}; console.log(endAdded);
可能會幫助野外的人:)
最簡單的方法是使用擴展運算符。
let obj = {'b': 2, 'c': 3};
let newObj = {'a': 1, ...obj};
JavaScript 對象是無序的。 沒有開始也沒有結束。 如果您想要訂單,請使用數組。
var arr = [
{ key: 'b', value: 2 },
{ key: 'c', value: 3 }
];
然后,您可以使用unshift
添加到它的前面:
arr.unshift({ key: 'a', value: 1 });
var obj = {'b': 2, 'c': 3};
obj = Object.assign({a: 1}, obj);
console.log(obj); // {a: 1, b: 2, c: 3}
希望這可以幫助
對我有用的是使用臨時對象按所需順序存儲項目。 例如:
var new_object = {};
new_object[name] = value; // The property we need at the start
for (var key in old_object) { // Looping through all values of the old object
new_object[key] = old_object[key];
delete old_object[key];
}
old_object = new_object; // Replacing the old object with the desired one
Javascript 可能不會在語言本身中指定屬性的順序,但至少在我的項目(也許是你的)中,它確實表現得好像它一樣( JSON.stringify
,調試觀察窗口等)。 我從未見過for...in
order 不是最初添加屬性的順序。 因此,對於我項目中的所有意圖和目的,也許是您的項目,這是非常可預測的。
雖然這不會影響執行,但如果您想看到一個統一的列表,當不同項目的屬性可能以不同的順序添加時,這會很痛苦,這取決於對象的創建方式。 執行可能不是項目開發中唯一重要的事情。 快速目視檢查對象的能力也可能很重要。
如果你已經spread
,如果你不介意另一個對象,我會使用它。 但是如果你沒有spread
(就像在舊的谷歌應用程序腳本中一樣),或者你需要保留原始對象,你可以這樣做:
對象.js
// Insert Property
Object.defineProperty(
Object.prototype,
'insertProperty',
{
value: function(name, value, index){
// backup my properties
var backup = {};
// delete all properties after index
var propertyIndex = 0;
for(var propertyName in this) {
if(this.hasOwnProperty(propertyName) && propertyIndex++ >= index) {
backup[propertyName] = this[propertyName];
delete this[propertyName];
}
}
this[name] = value;
// restore all properties after index
for(var propertyName in backup) {
if(backup.hasOwnProperty(propertyName))
this[propertyName] = backup[propertyName];
}
return this; // same object; not a new object
},
enumerable: false,
});
用法
var obj = {'b': 2, 'c': 3};
obj.insertProperty('a',1,0); // {a:1, b:2, c:3}
筆記
index
將屬性放置在您想要的任何位置。 0 把它放在前面。object prototype
添加方法,您當然可以將函數拉出並添加一個 obj 參數。 在我的項目中,向原型添加方法對我有幫助; 它不會傷害我。在某些情況下,您可能能夠僅使用您知道它最終可能具有的所有屬性按照您希望看到它們出現的順序創建您的對象,然后,無需插入屬性,只需設置該屬性。
// creation
var a = {Id: null, foo: 'bar'};
// update later
if(!a.Id)
a.Id = '123';
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.