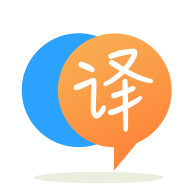
[英]Android AsyncTask.THREAD_POOL_EXECUTOR vs custom ThreadPool with Runnables
[英]what is the most simple and efficient way to return value from Runnables in Thread Pool Executor?
我在我的Android應用程序中創建了一個DataBaseManager
類,該類管理我的應用程序的所有數據庫操作。
我有不同的方法來創建,更新和從數據庫檢索值。
我在可運行對象上執行此操作,然后將其提交給線程池執行程序。
以防萬一,我必須從Runnable
返回一些值,我該如何實現它,我知道回調,但是隨着方法數量的增加,這對我來說並不麻煩。
任何幫助將不勝感激!
您需要使用Callable : Interface Callable<V>
使用它與Runnable一樣簡單:
private final class MyTask extends Callable<T>{
public T call(){
T t;
// your code
return t;
}
}
我使用T
表示引用類型,例如String
。
完成時獲取結果:
using Future<V>
:Future表示異步計算的結果。 提供了一些方法來檢查計算是否完成,以等待其完成。 計算完成后,使用get()方法檢索結果,必要時將其阻塞,直到准備就緒為止。
List<Future<T>> futures = new ArrayList<>(10); for(int i = 0; i < 10; i++){ futures.add(pool.submit(new MyTask())); } T result; for(Future<T> f: futures) result = f.get(); // get the result
上述方法的缺點是,如果第一個任務需要很長時間來計算,而所有其他任務在第一個任務之前結束,則當前線程無法在第一個任務結束之前計算結果。 因此,另一個解決方案是使用CompletionService 。
using CompletionService<V>
:一種服務,該服務將新異步任務的產生與完成任務的結果的消耗分開。 生產者提交任務以執行。 消費者執行已完成的任務,並按完成順序處理結果。 使用它很簡單,如下所示:
CompletionService<T> pool = new ExecutorCompletionService<T>(threadPool);
然后使用pool.take()。get()從可調用實例中讀取返回的結果:
for(int i = 0; i < 10; i++){ pool.submit(new MyTask()); } for(int i = 0; i < 10; i++){ T result = pool.take().get(); //your another code }
以下是使用Callable的示例代碼
import java.util.concurrent.Callable;
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
public class Test {
public static void main(String[] args) throws Exception {
ExecutorService executorService1 = Executors.newFixedThreadPool(4);
Future f1 =executorService1.submit(new callable());
Future f2 =executorService1.submit(new callable());
System.out.println("f1 " + f1.get());
System.out.println("f1 " + f2.get());
executorService1.shutdown();
}
}
class callable implements Callable<String> {
public String call() {
System.out.println(" Starting callable Asynchronous task" + Thread.currentThread().getName());
try {
Thread.currentThread().sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(" Ending callable Asynchronous task" + Thread.currentThread().getName());
return Thread.currentThread().getName();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.