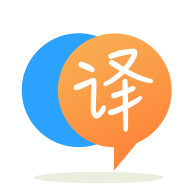
[英]JAXB/MOXy: Do not call XmlElementWrapper setter when element missing?
[英]JAXB/MOXy Adapting an XMLElementWrapper
我正在嘗試使用JAXB / MOXy來映射由一組具有鮮明特征的接口表示的域:所有多值元素都是由Iterables
封裝的,而不是數組或Collection
。 下面是一個玩具示例來說明我的情況。
Actor.java(注意Iterable<Movie>
)
package test.moxy;
public interface Actor {
public String getName();
public void setName(String name);
public Iterable<Movie> getMovies();
public void setMovies(Iterable<Movie> movies);
}
Movie.java
package test.moxy;
public interface Movie {
public String getTitle();
public void setTitle(String title);
}
主班
package test.moxy;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import org.eclipse.persistence.jaxb.JAXBContextProperties;
import org.xml.sax.InputSource;
public class TestBinding {
public static void main(String[] args) throws FileNotFoundException, JAXBException {
Class<?>[] types = new Class<?>[] { Actor.class, Movie.class };
List<String> mappings = new ArrayList<String>();
mappings.add("test/moxy/oxm.xml");
Map<String, Object> properties = new HashMap<String, Object>();
properties.put(JAXBContextProperties.OXM_METADATA_SOURCE, mappings);
JAXBContext jc = JAXBContext.newInstance(types, properties);
Unmarshaller unmarshaller = jc.createUnmarshaller();
InputSource is = new InputSource(new FileInputStream("src/main/java/test/moxy/input.xml"));
Actor actor = (Actor) unmarshaller.unmarshal(is);
Marshaller marshaller = jc.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(actor, System.out);
}
}
input.xml中
<?xml version="1.0" encoding="UTF-8"?>
<actor>
<name>John Smith</name>
<movies>
<movie>
<title>Smith's Trilogy - Part I</title>
</movie>
<movie>
<title>Smith's Trilogy - Part II</title>
</movie>
<movie>
<title>Smith's Trilogy - Part III</title>
</movie>
</movies>
</actor>
oxm.xml
<?xml version="1.0" encoding="UTF-8"?>
<xml-bindings xmlns="http://www.eclipse.org/eclipselink/xsds/persistence/oxm"
package-name="test.moxy" xml-mapping-metadata-complete="true">
<java-types>
<java-type name="Actor">
<xml-root-element name="actor" />
<xml-type prop-order="name movies" factory-class="test.moxy.ProxyFactory"
factory-method="initActor" />
<java-attributes>
<xml-element java-attribute="name" />
<xml-element java-attribute="movies" name="movie" >
<xml-element-wrapper name="movies" />
</xml-element>
</java-attributes>
</java-type>
<java-type name="Movie">
<xml-root-element name="movie" />
<xml-type factory-class="test.moxy.ProxyFactory"
factory-method="initMovie" />
<java-attributes>
<xml-element java-attribute="title" />
</java-attributes>
</java-type>
</java-types>
</xml-bindings>
ProxyFactory
是一種標准工廠,例如http://blog.bdoughan.com/2010/07/moxy-jaxb-map-interfaces-to-xml.html中示例的工廠
就目前而言,我得到以下JAXBException
:異常描述: XmlElementWrapper
只允許在collection或array屬性上使用,但[movies]不是collection或array屬性。
我嘗試在電影上使用XMLAdapter
在Iterable<T>
和List<T>
之間進行轉換,但它似乎適用於每個movie
元素,而不是movies
包裝器。 我想知道是否以及如何為包裝器指定諸如XMLAdapter
類的東西?
作為例外,您將無法使用@XmlElementWrapper
映射Iterable
的屬性。
Exception in thread "main" javax.xml.bind.JAXBException:
Exception Description: XmlElementWrapper is only allowed on a collection or array property but [movies] is not a collection or array property.
- with linked exception:
[Exception [EclipseLink-50015] (Eclipse Persistence Services - @VERSION@.@QUALIFIER@): org.eclipse.persistence.exceptions.JAXBException
Exception Description: XmlElementWrapper is only allowed on a collection or array property but [movies] is not a collection or array property.]
at org.eclipse.persistence.jaxb.JAXBContext$TypeMappingInfoInput.createContextState(JAXBContext.java:1068)
at org.eclipse.persistence.jaxb.JAXBContext.<init>(JAXBContext.java:182)
XmlAdapter(MoviesAdapter)
相反,我們將使用XmlAdapter
將Iterable
轉換為可映射的對象。 在這種情況下,我們將組成一個名為Movies
的類的實例,該實例具有Movie
實例List
。
package test.moxy;
import java.util.*;
import javax.xml.bind.annotation.adapters.XmlAdapter;
public class MoviesAdapter extends XmlAdapter<MoviesAdapter.Movies, Iterable<Movie>> {
public static class Movies {
public List<Movie> movie = new ArrayList<Movie>();
}
@Override
public Iterable<Movie> unmarshal(Movies v) throws Exception {
return v.movie;
}
@Override
public Movies marshal(Iterable<Movie> v) throws Exception {
Movies movies = new Movies();
for(Movie movie : v) {
movies.movie.add(movie);
}
return movies;
}
}
外部元數據(oxm.xml)
下面是修改后的oxm.xml
引用XmlAdapter
。 使用新的映射, Movies
對象將導致movies
元素存在,因此我們不再需要@XmlElementWrapper
元數據。
<?xml version="1.0" encoding="UTF-8"?>
<xml-bindings xmlns="http://www.eclipse.org/eclipselink/xsds/persistence/oxm"
package-name="test.moxy" xml-mapping-metadata-complete="true">
<java-types>
<java-type name="Actor">
<xml-root-element name="actor" />
<xml-type prop-order="name movies" factory-class="test.moxy.ProxyFactory"
factory-method="initActor" />
<java-attributes>
<xml-element java-attribute="name" />
<xml-element java-attribute="movies">
<xml-java-type-adapter value="test.moxy.MoviesAdapter"/>
</xml-element>
</java-attributes>
</java-type>
<java-type name="Movie">
<xml-root-element name="movie" />
<xml-type factory-class="test.moxy.ProxyFactory"
factory-method="initMovie" />
<java-attributes>
<xml-element java-attribute="title" />
</java-attributes>
</java-type>
</java-types>
</xml-bindings>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.