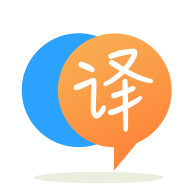
[英]How to decrement count of barrier on thread completion using pthreads in C
[英]Increasing pthreads thread count has no effect on speed
在以下程序中,增加線程數並不會產生任何加速優勢(使用linux下的time命令測量)。 我在以下處理器上運行它:
在我看來,在線程之間划分工作的邏輯是正確的。 我甚至嘗試取下鎖,這顯然會給出錯誤的結果,但仍然沒有增加速度。 有任何想法嗎?
#include <pthread.h>
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
double sum;
pthread_mutex_t mutex;
typedef struct {
int start;
int end;
}sumArg;
void *sumRoots(void *arg) {
sumArg s = *((sumArg *) arg);
int i = s.start;
double tmp;
while(i <= s.end) {
tmp = sqrt(i);
pthread_mutex_lock(&mutex);
sum += tmp;
pthread_mutex_unlock(&mutex);
i++;
}
free(arg);
}
int main(int argc, char const *argv[]) {
int threadCount = atoi(argv[1]);
int N = atoi(argv[2]);
if (N < 1 || threadCount < 1) printf("Usage: ./sumOfRoots threads N\n");
pthread_t tid[threadCount];
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_mutex_init(&mutex, NULL);
sumArg *s;
int i = 0;
while(i < threadCount) {
s = (sumArg *) malloc(sizeof(sumArg));
s->start = ((N/threadCount) * i) + 1;
s->end = (N/threadCount) * (i + 1);
pthread_create(&tid[i], &attr, sumRoots, s);
i++;
}
i = 0;
while(i < threadCount) pthread_join(tid[i++], NULL);
printf("sum: %f\n", sum);
return 0;
}
編輯:以下是程序的一些運行,全部在i5 M520上運行:
time ./sumOfRoots 1 1000000000
sum: 21081851083600.558594
real 0m21.933s
user 0m21.268s
sys 0m0.000s
time ./sumOfRoots 2 1000000000
sum: 21081851083600.691406
real 0m21.207s
user 0m21.020s
sys 0m0.008s
time ./sumOfRoots 4 1000000000
sum: 21081851083600.863281
real 0m21.488s
user 0m21.116s
sys 0m0.016s
time ./sumOfRoots 8 1000000000
sum: 21081851083601.777344
real 0m21.432s
user 0m21.092s
sys 0m0.020s
我相信總和的變化是由浮點精度損失引起的。
時間基本保持不變的原因在於它以同步為主導。 在我的計算機上,單線程解決方案甚至更快!
如下更改代碼會使時間符合預期:
void *sumRoots(void *arg) {
sumArg s = *((sumArg *) arg);
int i = s.start;
double tmp = 0;
while(i <= s.end) {
tmp += sqrt(i++);
}
pthread_mutex_lock(&mutex);
sum += tmp;
pthread_mutex_unlock(&mutex);
free(arg);
return 0;
}
現在你的線程運行了一段時間沒有同步,然后在添加過程中只進行一次同步。
我在系統上看到的時間如下:
> time ./a.out 1 1000000000
sum: 21081851083600.558594
real 0m13.220s
user 0m13.098s
sys 0m0.009s
> time ./a.out 2 1000000000
sum: 21081851083600.863281
real 0m6.613s
user 0m12.930s
sys 0m0.027s
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.