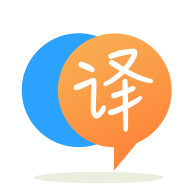
[英]Get `UITableViewCell` indexPath using `UIScrollView`
[英]Get the UITableViewCell indexPath using custom cells
我有一個包含UITableView的視圖。 此UITableView的單元格在Interface Builder中創建,以便具有不同類型的單元格。 因此,每個按鈕的操作都在單元類中進行管理,如下所示。
- 我的UITableView包含不同類型的單元格:
- 我的類的頭文件為類型一單元格(“CellTypeOne.h”) :
@interface CellTypeOne : UITableViewCell
{
}
- (IBAction)actionForFirstButton:(id)sender;
- (IBAction)actionForSecondButton:(id)sender;
- (IBAction)actionForThirdButton:(id)sender;
- (IBAction)actionForFourthButton:(id)sender;
- 我的類的頭文件,用於類型二單元格(“CellTypeTwo.h”) :
@interface CellTypeTwo : UITableViewCell
{
}
- (IBAction)actionForTheUniqueButton:(id)sender;
- 包含我的表視圖的視圖( “ViewContainingMyTableView.h” ):
@interface ViewContainingMyTableView : UIViewController <UITableViewDelegate, UITableViewDataSource>
{
UITableView *myTBV;
}
@property (retain, nonatomic) IBOutlet UITableView *myTBV;
@end
這是我想要做的事情:
例如,當我單擊第一個單元格中的第一個按鈕時,我希望能夠顯示“當前”單元格的indexPath。
例如 ,我希望在以下情況下輸出以下內容:
0
0
1
因為你正在使用自定義單元格我認為你還需要處理選擇,因為你正在觸摸自定義單元格內的按鈕而不是單元格本身,因此tableview委托方法沒有被觸發,更好,因為我在你的自定義單元格中說了一個委托方法例如在你的自定義單元格中
在你的CellTypeOne.h
添加這個
//@class CellTypeOne; //if u want t pass cell to controller
@protocol TouchDelegateForCell1 <NSObject> //this delegate is fired each time you clicked the cell
- (void)touchedTheCell:(UIButton *)button;
//- (void) touchedTheCell:(CellTypeOne *)cell; //if u want t send entire cell this may give error add `@class CellTypeOne;` at the beginning
@end
@interface CellTypeOne : UITableViewCell
{
}
@property(nonatomic, assign)id<TouchDelegateForCell1> delegate; //defining the delegate
- (IBAction)actionForFirstButton:(id)sender;
- (IBAction)actionForSecondButton:(id)sender;
- (IBAction)actionForThirdButton:(id)sender;
- (IBAction)actionForFourthButton:(id)sender;
在您的CellTypeOne.m
文件中
@synthesize delegate; //synthesize the delegate
- (IBAction)actionForFirstButton:(UIButton *)sender
{
//add this condition to all the actions becz u need to get the index path of tapped cell contains the button
if([self.delegate respondsToSelector:@selector(touchedTheCell:)])
{
[self.delegate touchedTheCell:sender];
//or u can send the whole cell itself
//for example for passing the cell itself
//[self.delegate touchedTheCell:self]; //while at the defining the delegate u must change the sender type to - (void)touchedTheCell:(CellTypeOne *)myCell; if it shows any error in the defining of the delegate add "@class CellTypeOne;" above the defying the delegate
}
}
並在您的ViewContainingMyTableView.h
@interface ViewContainingMyTableView : UIViewController <UITableViewDelegate, UITableViewDataSource ,TouchDelegateForCell1> //confirms to custom delegate like table delegates
{
UITableView *myTBV;
}
@property (retain, nonatomic) IBOutlet UITableView *myTBV;
@end
並在ViewContainingMyTableView.m
文件中
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//during the creating the custom cell
CellTypeOne *cell1 = [self.aTableView dequeueReusableCellWithIdentifier:@"cell"];
if(cell1 == nil)
{
cell1 = [[CustomCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:@"cell"];
}
cell.delegate = self; //should set the delegate to self otherwise delegate methods does not called this step is important
}
//now implement the delegate method , in this method u can get the indexpath of selected cell
- (void)touchedTheCell:(UIButton *)button
{
NSIndexPath *indexPath = [self.aTableView indexPathForCell:(UITableViewCell *)button.superview];
NSLog(@"%@",indexPath.description);
}
/* if u pass the cell itself then the delegate method would be like below
- (void)touchedTheCell:(CellTypeOne *)myCell
{
NSIndexPath *indexPath = [self.aTableView indexPathForCell:myCell];//directly get the cell's index path
//now by using the tag or properties, whatever u can access the contents of the cell
UIButton *myButton = [myCell.contentView viewWithTag:1000]; //get the button
//... u can access all the contents in cell
}
*/
在你的情況下,這是單元格中的第一個按鈕,為每個具有不同功能的按鈕添加委托方法,對單元格中的另一個按鈕重復上述過程
希望你得到這個:) hapy編碼
使用iOS7,您可以這樣:
- (IBAction)actionForTheUniqueButton:(id)sender
{
YouCellClass *clickedCell = (YouCellClass*)[[sender superview] superview];
NSIndexPath *indexPathCell = [self.tableView indexPathForCell:clickedCell];
}
您可以在每個單元格的xib中使用標記來區分它們。
在單元格類中的[self tag]
之后使用
在.h或.m文件中創建tableView IBOutlet。 您可以使用內部的actionForFirstButton:方法獲取所選表格單元的索引路徑
NSIndexPath *indexPath = [self.tableView indexPathForSelectedRow];
NSLog(@"I click on the first button in the first cell : %d", indexPath.row);
編輯 :在actionForFirstButton方法中使用按鈕的標記來獲取索引路徑
if(sender.tag == 0 || 1 || 2 || 3){
{
NSLog(@"the cell is : %d", 0);
}
else if(sender.tag == 4){
{
NSLog(@"the cell is : %d", 1);
}
else{
NSLog(@"the cell is : %d", 2);
}
您的要求只是獲取按鈕的相應行值...
然后你有一個非常簡單的方法,
@interface CellTypeOne : UITableViewCell
{
NSNumber *rowval;
}
@property (nonatomic,retain)NSNumber* rowval;
你的CellTypeTwo.h中也是一樣的
@interface CellTypeTwo : UITableViewCell
{
NSNumber *rowval;
}
@property (nonatomic,retain)NSNumber* rowval;
現在在.m文件中你必須為按鈕定義一個方法,所以在這些方法中只需粘貼這一行
NSLog(@"Number : %d",[rowval integerValue]);
在tableView cellForRowAtIndexPath:方法中也添加此行
cell.rowval = [[NSNumber alloc]initWithInt:indexPath.row ];
現在,每當您按下不同單元格的按鈕時,它將回復單元格的行值
希望這會有所幫助.. :-)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.