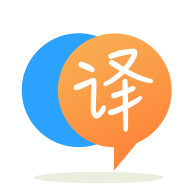
[英]Gimp, python-fu: Align layer with respect to background layer in image
[英]python-fu Copy an Image
我有一個顏色列表文件,將其讀取到python列表中,我想創建一個(或幾個)由正方形組成的圖像,這些正方形具有從文件中讀取的背景色,並作為該顏色的前景html字符串(用白色)。 即我從輸入文件中讀取#ff0000,然后創建一個100x100的正方形,紅色背景,白色字符串“#ff0000”作為前景...依此類推,對輸入文件中的每種顏色。
這是我的腳本:
#!/usr/bin/env python
from gimpfu import *
def readColors(ifc):
"""Read colors from input file and return a python list with all them"""
a = []
fd = open(ifc,"r")
for i in fd:
if not i.startswith("//"):#skip comment lines
a.append(i.rstrip('\n'))
return a
def rgb2html(col):
"""Converts a color: from (255,255,255) to #ffffff"""
r,g,b = col
return "#%x%x%x" % (r,g,b)
def html2rgb(col):
"""Converts a color: from #ffffff to (255,255,255)"""
s=col.strip('#')
r=int(s[:2],16)
g=int(s[2:4],16)
b=int(s[4:6],16)
return r,g,b
def nextColor():
"""Gets next html color from color list"""
col = nextColor.array[nextColor.counter]
nextColor.counter +=1
return col
def isNextColor():
"""Is there another color or list is over?"""
return nextColor.counter<isNextColor.colorslenght
def isPageEnded(y,YSIZE):
"""Is there enough room to draw another square?"""
return (y+100)>=YSIZE
def newPage(XSIZE,YSIZE):
"""Returns a new image"""
return gimp.Image(XSIZE,YSIZE,RGB)
def createImage(color,text):
"""Draws a square filled with color and white color text. It works!"""
gimp.set_foreground((255,255,255))#text color
gimp.set_background(color) #background color
image = gimp.Image(100,100,RGB)
back = gimp.Layer(image,"font",100,100,RGB_IMAGE,100,NORMAL_MODE)
image.add_layer(back,1)
back.fill(BACKGROUND_FILL)
lay = back.copy()
lay.name = "font"
image.add_layer(lay,0)
lay = pdb.gimp_text_fontname(image,None,2,100/4,text,2,True,18,PIXELS,"Sans")
image.merge_down(lay, NORMAL_MODE)
image.flatten()
return image
def drawRect(image,x,y):
"""Here I'd like to copy the result of createImage at current x,y position of current image. It doesn't work!"""
text = nextColor()
color = html2rgb(text)
img = createImage(color,text)
drawable = pdb.gimp_image_active_drawable(image)
image.disable_undo()
pdb.gimp_selection_none(image)
pdb.gimp_image_select_rectangle(image, 2, x, y, 100, 100)
if pdb.gimp_edit_named_copy_visible(img, "buffer"):
floating_sel = pdb.gimp_edit_named_paste(drawable, "buffer", TRUE)
pdb.gimp_selection_none(image)
image.flatten()
gimp.Display(image)#to debug
image.enable_undo()
def savePage(image,directory,filename):
"""Saves composed image to filename"""
drawable = pdb.gimp_image_active_drawable(image)
pdb.file_png_save(image, drawable, directory+"\\"+filename, filename, 0,9,1,0,0,1,1)
def draw(ifile,savedir,prefix):
"""Main method. it manage page's room, slicing it in several 100x100 squares"""
YSIZE = 1000
XSIZE = 1000
x = y = pc = 0
colorArray = readColors(ifile)
nextColor.counter = 0
nextColor.array = colorArray
isNextColor.colorslenght = len(colorArray)
pdb.gimp_context_push()
image = newPage(XSIZE,YSIZE)
while(isNextColor()):
drawRect(image,x,y)
x += 100 # move to next column
if x+100>=XSIZE:#move to next row
x = 0
y += 100
if isPageEnded(y,YSIZE):
savePage(image,savedir,prefix+str(pc)+".png")
gimp.Display(image)#to debug
pc += 1
image = newPage(XSIZE,YSIZE)
x = 0
y = 0
savePage(image,savedir,prefix+str(pc)+".png")#save last page
pdb.gimp_context_pop()
ifc = '<path to color list file>\\colors.txt'
ofc = '<path to output directory>\\palette'
prefix = 'table' #prefix for each file(i.e.: table0.png, table1.png...)
draw(ifc,ofc,prefix) #main method call
我當前的問題是drawRect方法。 我無法復制在drawRect方法中較大圖像的坐標x,y處createImage方法返回的圖像。 在drawRect方法中,我嘗試使用gimp的“復制到選擇中”:選擇一個區域,然后粘貼從另一張圖像復制的內容。 但是我在圖層上遇到了麻煩,無法將圖像復制到正確的位置。
為了完整起見,這是ifc文件中的幾行:
#b6ffff
#f079f3
#9979f3
#79c2f3
#7a79f3
#79f380
#f3799a
在此先感謝您的幫助。
阿爾貝托
也許對其他人有用(它必須另存為“ draw-colors.py”並復制到GIMP 2 / lib / gimp / 2.0 / plug-ins文件夾中):
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from gimpfu import *
def readColors(ifile):
"""Read colors from input file and return a python list with all them"""
a = []
fd = open(ifile,"r")
for i in fd:
if not i.startswith('//'):
a.append(i)
return a
def rgb2html(col):
"""Converts a color: from (255,255,255) to #ffffff"""
r,g,b = col
return "#%x%x%x" % (r,g,b)
def html2rgb(col):
"""Converts a color: from #ffffff to (255,255,255)"""
s=col.strip('#')
r=int(s[:2],16)
g=int(s[2:4],16)
b=int(s[4:6],16)
return r,g,b
def nextColor():
"""Gets next html color from color list"""
col = html2rgb(nextColor.array[nextColor.counter])
nextColor.counter +=1
return col
def isNextColor():
"""Is there another color or list is over?"""
return nextColor.counter<isNextColor.colorslenght
def isPageEnded(y,sizey):
"""Is there enough room to draw another square?"""
return (y+100)>=sizey
def newPage(sizex,sizey):
"""Returns a new image"""
image = pdb.gimp_image_new(sizex, sizey, RGB)
layer = pdb.gimp_layer_new(image, sizex, sizey, RGB, "layer", 0, 0)
pdb.gimp_image_add_layer(image, layer, 0)
drw = pdb.gimp_image_active_drawable(image)
pdb.gimp_context_set_background((255,255,255))
pdb.gimp_drawable_fill(drw, BACKGROUND_FILL)
pdb.gimp_context_set_brush('Circle (01)')
return image
def savePage(image,filename):
"""Saves composed image to filename"""
layers = image.layers
last_layer = len(layers)-1
try:
disable=pdb.gimp_image_undo_disable(image)
pdb.gimp_layer_add_alpha(layers[0])
pdb.plug_in_colortoalpha(image,image.active_layer,(0,0,0))
layer = pdb.gimp_image_merge_visible_layers(image, 1)
enable = pdb.gimp_image_undo_enable(image)
pdb.file_png_save(image, image.active_layer, filename, filename, 0,9,1,0,0,1,1)
except Exception as e:
raise e
def drawRect(x,y,color,image):
"""draw a single square"""
text = rgb2html(color)
pdb.gimp_image_select_rectangle(image, 2, x, y, 100, 100)
pdb.gimp_context_set_background(color)
pdb.gimp_edit_fill(image.active_layer, 1)
try:
text_layer = pdb.gimp_text_fontname(image, None, x, y+(100/2), text, 2, 1, 18, 0, "Sans")
pdb.gimp_image_merge_down(image, text_layer, 0)
except Exception as e:
raise e
def draw(ifile,odir,prefix,sizex,sizey):
"""Main method. it manage page's room, slicing it in several 100x100 squares"""
colorArray = readColors(ifile)
nextColor.counter = 0
nextColor.array = colorArray
isNextColor.colorslenght = len(colorArray)
pc = x = y = 0
image = newPage(sizex,sizey)
try:
while(isNextColor()):
pdb.gimp_context_push()
drawRect(x,y,nextColor(),image)
x += 100#cambia colonna
if x+100>=sizex:#cambia riga
x = 0
y += 100
if isPageEnded(y,sizey):#salva pagina
savePage(image,odir+'\\'+prefix+str(pc)+".png")
pc += 1
image = newPage(sizex,sizey)
x = y = 0
savePage(image,odir+'\\'+prefix+str(pc)+".png")
pdb.gimp_context_pop()
except Exception as e:
raise e
register(
"draw-colors",
N_("Create a color map from a text color file"),
"Create a color map from a text color file",
"Alberto",
"@Copyright Alberto 2014",
"2014/10/21",
N_("Draw Colors..."),
"",
[
(PF_FILE, "ifile", N_("Color input file:"), 'default\\input\\colorfile\\path\\colorlist.txt'),
(PF_DIRNAME, "odir", N_("Path for png export:"), 'default\\output\\path'),
(PF_STRING, "prefix", N_("Filename prefix for export:"), "table"),
(PF_INT8, "sizex", N_("Image's x size:"), 1024),
(PF_INT8, "sizey", N_("Image's y size:"), 1024)
],
[],
draw, #main method call
menu="<Image>/Filters/Alberto",
domain=("gimp20-python", gimp.locale_directory)
)
main()
輸出示例:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.