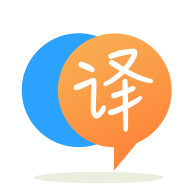
[英]Trying to read a list of floats, integers, and strings from a file with sscanf() in C is not working as expected
[英]C - reading multiple integers and floats with sscanf
我正在為我的C編程類做一個練習問題,該問題告訴我編寫一個程序來讀取文件中的變量。 在第一行,它應該讀入整數N。
從那里,它應該讀取一個整數,然后讀取N行的每行五個浮點數。 應該計算出文件中所有浮點數的總和,然后將其寫入另一個文件。
我編寫了一個程序,該程序應該使用fgets將行復制到字符串,然后使用sscanf對其進行剖析並將段分配給不同的數組位置。 但是,通過sscanf(可能為空值或換行符)獲取無關信息時遇到了一些問題。 它沒有正確存儲整數N(它會產生較大的隨機值並通過無限循環產生運行時錯誤),並且它也可能不在循環內部運行。
我該如何完善它,使其讀入整數並正確浮動?
#include <stdio.h>
#include <stdlib.h>
#define MAX_STRING 30
#define MAX_LINE_SIZE 200
int main(void)
{
FILE *f1, *f2;
char filename[MAX_STRING];
char fileline[MAX_LINE_SIZE];
int N, eN;
float totalwage = 0;
int* ssn;
float** wage;
printf ("Enter a file name for data analysis: ");
scanf ("%s", &filename); //get file name from user input for reading
f1 = fopen (filename, "r");
fgets (fileline, MAX_LINE_SIZE, f1); //read first line
sscanf (fileline, "%d", &N); //pull integer from first line to determine how many lines follow
for (eN = 0; eN < N; eN ++) //read N lines following the first
{
// VVV read single line from file
fgets (fileline, MAX_LINE_SIZE, f1);
// VVV record data from line
sscanf (fileline, "%d, %f, %f, %f, %f, %f", &ssn[eN], &wage[eN][0], &wage[eN][1], &wage[eN][2], &wage[eN][3], &wage[eN][4]);
// VVV add the 5 wages on each line to a total
totalwage += wage[eN][0] + wage[eN][1] + wage[eN][2] + wage[eN][3] + wage[eN][4];
}
fclose (f1);
printf ("Enter a file name for the result: ");
scanf ("%s", &filename); //get file name from user input for writing
f2 = fopen (filename, "w");
fprintf (f2, "%f", totalwage); //store total of wages in file specified by user
printf ("\nThe information has been stored. Press any key to exit.\n");
getchar();
}
正在讀取的文件是“ wages.txt”,其內容如下:
10
1, 10, 20, 30, 40, 50
2, 11, 12, 13, 14, 15
3, 21, 23, 25, 27, 29
4, 1, 2, 3, 4, 5
5, 30, 60, 90, 120, 150
6, 37, 38, 39, 40, 41
7, 40, 50, 60, 70, 80
8, 5, 10, 15, 20, 25
9, 80, 90, 100, 110, 120
10, 1000, 2000, 3000, 4000, 2000
回顧一下,問題在於存在運行時錯誤,由於某種無限循環,程序會崩潰。 通過一些調試,我發現它沒有在第一行中正確讀取整數。 而不是值十,它存儲較大的值,就像讀取空字符一樣。
我添加了代碼,試圖為ssn和工資分配內存。 但是,我不確定該操作是否正確完成,並且程序仍然存在崩潰的運行時錯誤。
ssn = malloc (N*MAX_STRING);
wage = malloc (N*MAX_STRING);
for (eN = 0; eN < N; eN ++)
{
wage[eN] = malloc (N*MAX_STRING);
}
您沒有為工資分配任何內存。 它被聲明為一個指向浮點數的指針。 很好,但是指針沒有指向任何地方。
ssn也一樣。
在此行之后:
sscanf (fileline, "%d", &N); //pull integer from first line to determine how many lines follow
您需要為ssn和工資分配內存。
由於這是家庭作業,因此我不會告訴您如何分配內存。 您需要能夠自己弄清楚。
正如其他人指出的
scanf ("%s", &filename);
應該:
scanf ("%s", filename);
請注意,如果列數更改,則sscanf
是一個大問題
修訂用於strtod
:
#include <stdio.h>
#include <stdlib.h>
#define MAX_STRING 30
#define MAX_LINE_SIZE 200
#define COLS 5
int main(void)
{
FILE *f1, *f2;
char filename[MAX_STRING];
char fileline[MAX_LINE_SIZE];
int N, eN, i;
float totalwage = 0;
float (*wage)[COLS];
char *p;
printf ("Enter a file name for data analysis: ");
scanf ("%s", filename); //get file name from user input for reading
f1 = fopen (filename, "r");
fgets (fileline, MAX_LINE_SIZE, f1); //read first line
sscanf (fileline, "%d", &N); //pull integer from first line to determine how many lines follow
wage = malloc((sizeof(float) * COLS) * N);
/* check malloc ... */
for (eN = 0; eN < N; eN ++) //read N lines following the first
{
// VVV read single line from file
p = fgets (fileline, MAX_LINE_SIZE, f1);
strtol(p, &p, 10); /* skip first column */
for (i = 0; i < COLS; i++) {
wage[eN][i] = strtod(p + 1, &p); /* p+1 skip comma, read a float */
totalwage += wage[eN][i]; /* sum */
}
}
free(wage);
fclose (f1);
printf ("Enter a file name for the result: ");
scanf ("%s", filename); //get file name from user input for writing
f2 = fopen (filename, "w");
fprintf (f2, "%f", totalwage); //store total of wages in file specified by user
printf ("\nThe information has been stored. Press any key to exit.\n");
getchar();
fclose(f2); /* you forget to close f2 */
return 0;
}
還要注意,您只讀取浮點數,不需要存儲它們,因此可以避免使用malloc
,並且在現實世界中檢查fopen
, fgets
的結果...
當您分配內存來存儲一個浮點數時,您需要了解一個浮點數包含四個字節,因此在調用malloc時,需要考慮到這一點,以存儲一個浮點數: malloc( sizeof(float ) )
以存儲數組大小int的float malloc( N*sizeof(float ) )
是sizeof(int)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.